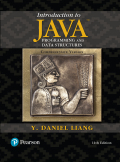
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134700144
Author: Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 26.7, Problem 26.7.4CP
Program Plan Intro
AVL tree: It is a self-balancing binary search tree (BST). If the tree is not balanced, the tree performs rotation operation.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Using the notation
you can select multipy options
For each of the following, decide whether the claim is True or False and select the True ones:
Suppose we discover that the 3SAT can be solved in worst-case cubic time. Then it would
mean that all problems in NP can also be solved in cubic time.
If a problem can be solved using Dynamic Programming, then it is not NP-complete.
Suppose X and Y are two NP-complete problems. Then, there must be a polynomial-time
reduction from X to Y and also one from Y to X.
Chapter 26 Solutions
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
Ch. 26.2 - Prob. 26.2.1CPCh. 26.2 - Prob. 26.2.2CPCh. 26.2 - Prob. 26.2.3CPCh. 26.3 - Prob. 26.3.1CPCh. 26.3 - Prob. 26.3.2CPCh. 26.3 - Prob. 26.3.3CPCh. 26.4 - Prob. 26.4.1CPCh. 26.4 - Prob. 26.4.2CPCh. 26.4 - Prob. 26.4.3CPCh. 26.4 - Prob. 26.4.4CP
Ch. 26.5 - Use Listing 26.2 as a template to describe the...Ch. 26.6 - Prob. 26.6.1CPCh. 26.6 - Prob. 26.6.2CPCh. 26.6 - Prob. 26.6.3CPCh. 26.6 - Prob. 26.6.4CPCh. 26.7 - Prob. 26.7.1CPCh. 26.7 - Prob. 26.7.2CPCh. 26.7 - Prob. 26.7.3CPCh. 26.7 - Prob. 26.7.4CPCh. 26.8 - Prob. 26.8.1CPCh. 26.8 - Prob. 26.8.2CPCh. 26.8 - Prob. 26.8.3CPCh. 26.9 - Prob. 26.9.1CPCh. 26.9 - Prob. 26.9.2CPCh. 26.9 - Prob. 26.9.3CPCh. 26 - Prob. 26.5PE
Knowledge Booster
Similar questions
- Maximum Independent Set problem is known to be NP-Complete. Suppose we have a graph G in which the maximum degree of each node is some constant c. Then, is the following greedy algorithm guaranteed to find an independent set whose size is within a constant factor of the optimal? 1) Initialize S = empty 2) Arbitrarily pick a vertex v, add v to S delete v and its neighbors from G 3) Repeat step 2 until G is empty Return S Yes Noarrow_forwardPlease help me answer this coding question in the images below for me(it is not a graded question):write the code using python and also provide the outputs requiredarrow_forwardWhat does the reduction showing Vertex Cover (VC) is NP-Complete do: Transforms any instance of VC to an instance of 3SAT Transforms any instance of 3SAT to an instance of VC Transforms any instance of VC to an instance of 3SAT AND transforms any instance of 3SAT to an instance of VC none of the abovearrow_forward
- Please assist me by writing out the code with its output (in python) using the information provided in the 2 images below.for the IP Address, it has been changed to: 172.21.5.204the serve code has not been open yet though but the ouput must be something along these lines(using command prompt):c:\Users\japha\Desktop>python "Sbongakonke.py"Enter the server IP address (127.0.0.1 or 172.21.5.199): 172.21.5.204Enter your student number: 4125035Connected to server!It's your turn to pour! Enter the amount to your pour (in mL):Please work it out until it gets the correct outputsNB: THIS QUESTION IS NOT A GRADED QUESTIONarrow_forwardneed help with a html code and css code that will match this image.arrow_forwardneed help with a html code and css code that will match this image. Part B - A Navigation Part B is the navigation component of a page. Information you need includes: Color Codes: Visiting links: #ff6666 Unvisited links: #ccff66 Hovered links: white Search box: #2ec4b6 rebeccapurple white Font: Google Font (Roboto) Icons: Font Awesome (fa-quidditch, fa-search) This is a flexbox based navigation menu. Other then padding, all spacing/positioning should be controlled using flex properties. The home link in the nav should point to your assignment file (to triggers visited styling). In the "state" screenshot below, Home is visited, Services is hovered (the mouse doesn't show up in the screenshot) and Products is unvisited.arrow_forward
- MGMT SS STATS, an umbrella body that facilitates and serves various Social Security Organizations/Departments within the Caribbean territories, stood poised to meet the needs of its stakeholders by launching an online database. The database will provide members and the public access to the complete set of services that can (also) be initiated face-to-face, and it will provide managed, private, secure access to a repository of public and/or personal information. Ideally, the database will have basic details of pension plans recorded in the registry, member plan statistics, and cash inflows and outflows from pension funds.For example, insured persons accumulate contributions. Records for these persons will include information on the insured persons able to acquire various benefits once work is interrupted due to sickness, death, retirement, and maternity or employment injury. They will also include information on pensions such as invalidity, disability, and survivors that stem from one…arrow_forwardWhy all appvif i want to sign in its required phone number why not using google or apple its make me frustratedarrow_forwardWhy is the accuracy of time important in data visualizations? Detail a scenario from your professional experience in which time was structured poorly in a data visualization. How did this affect the understanding of the data presented? How do you think this error or oversight occurred?arrow_forward
- Write the KeanStudent class. The UML diagram of the class is represented below: KeanStudent - fullName: String - keanID: int -keanEmailAddress: String cellPhoneNumber: String + numberOfStudent: int + KeanStudent() + KeanStudent(fullName: String, keanID: int, keanEmailAddress: String, cellPhoneNumber: String) +getFullName(): String +setFullName(newFullName: String): void +getKeanIDO): int +getKeanEmailAddress(): String +getCellPhoneNumber(): String + setCellPhoneNumber(newCellPhoneNumber: String): void +toString(): String 1. Implement the KeanStudent class strictly according to its UML one-to-one (do not include anything extra, do not miss any data fields or methods) 2. Implement a StudentTest class to test the class KeanStudent you just created. • Create two KeanStudent objects using a no-args constructor and one from the constructor with all fields. o Print the contents of both objects. 。 Print numberOfStudent. 3. Add comments to your program (mark where data fields, constructors,…arrow_forwardMGMT SS STATS, an umbrella body that facilitates and serves various Social SecurityOrganizations/Departments within the Caribbean territories, stoodpoised to meet the needs of its stakeholders by launching an onlinedatabase at www.SSDCI.gov. The database will provide membersand the public access to the complete set of services that can (also)be initiated face-to-face, and it will provide managed, private, secure access to a repository ofpublic and/or personal information. Ideally, the database will have basic details of pensionplans recorded in the registry, member plan statistics, and cash inflows and outflows frompension funds.For example, insured persons accumulate contributions. Records for these persons will includeinformation on the insured persons able to acquire various benefits once work is interrupteddue to sickness, death, retirement, and maternity or employment injury. They will also includeinformation on pensions such as invalidity, disability, and survivors that stem from…arrow_forward(c) Consider the following set of processes: Process ID Arrival Time Priority Burst Time A 2 3 100 B 6 C 10 1 (highest) 2 40 80 D 16 4 (lowest) 20arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
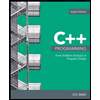
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
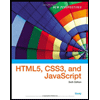
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
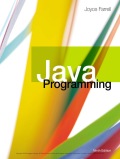
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
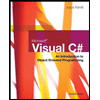
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
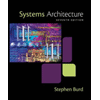
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning