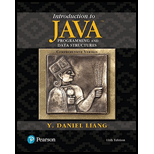
Concept explainers
Generic bubble sort
Program Plan:
- Import the required packages.
- Create a class “Sorting”:
- Method to sort the numbers gets defined.
- Call the method bubble sort that implements the comparable interface.
- Method to sort the string given.
- Call the method bubble sort to implement the comparator interface.
- Loop that iterates to sort the values of the list.
- Perform swap operation by comparing the list.
- Return the sorted list.
- Define the main method
- Initialize the list that needs to be sorted.
- Call the bubble sort.
- Display the sorted list.
- Initialize list that contain strings.
- Call the method bubble sort.
- Display the sorted list.

The below program is perform a generic bubble sort.
Explanation of Solution
Program:
//define the required packages
import java.util.Comparator;
//define the class sorting
public class Sorting
{
//method to sort the numbers
public static <E extends Comparable<E>> void bubbleSort(E[] list)
{
//perform bubble sort
bubbleSort(list, (e1, e2) -> ((Comparable<E>)e1).compareTo(e2));
}
//method definition
public static <E> void bubbleSort(E[] list,
Comparator<? super E> comparator)
{
//declare the necessary variables
boolean needNextPass = true;
/*loop that iterates to sort the elements of the array*/
for (int k = 1; k < list.length && needNextPass; k++)
{
/*condition when the given array is sorted*/
needNextPass = false;
for (int i = 0; i < list.length - k; i++)
{
/*condition to validate the elements*/
if (comparator.compare(list[i], list[i + 1]) > 0)
{
// swap the elements
E temp = list[i];
//assign values
list[i] = list[i + 1];
//assign the value
list[i + 1] = temp;
/*assign value for the next path*/
needNextPass = true;
}
}
}
}
//define the values
public static void main(String[] args)
{
//define anew list
Integer[] new_list = {-8, 10, 5, 7, 14, 12, -12, 31, 141, 102};
//call the method bubble sort
bubbleSort(new_list);
//loop that iterates to sort the list
for (int i = 0; i < new_list.length; i++)
{
//display the list
System.out.print(new_list[i] + " ");
}
System.out.println();
//define anew list
String[] new_list1 = {"Sorting", "Data", "Fun", "Happy", "
//call bubble sort
bubbleSort(new_list1, (s1, s2) -> s1.compareToIgnoreCase(s2));
//loop that iterates to sort the values
for (int i = 0; i < new_list1.length; i++)
{
//display the list
System.out.print(new_list1[i] + " ");
}
}
}
-12 -8 5 7 10 12 14 31 102 141
Data Fun Happy Nice Programming Sorting
Want to see more full solutions like this?
Chapter 23 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
- Provide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forwardComplete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forward
- Solve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forwardInformation Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forward
- Could you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forwardFor this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
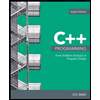
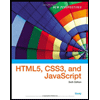
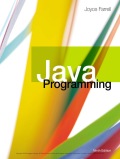
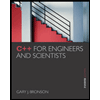
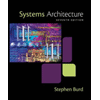