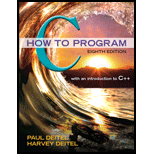
C How to Program (8th Edition)
8th Edition
ISBN: 9780133976892
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 22, Problem 22.31E
Program Plan Intro
- To define a function first which throws an exception.
- To define four more functions and make them call the previous function i.e. create a nested function. For example, the function second calls the function first and so on.
- Use a catch handler to catch the exception and display the result.
Summary Introduction- The program throws an exception in the deeply nested function and catches in the main.
Program Description- The purpose of the program to throw an exception in the deeply nested function and catches in the main.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
(Stack Unwinding) Write a program that throws an exception from a deeply nested function and still has the catch handler following the try block enclosing the initial call in main catchthe exception.
10) In C++, try-catch blocks can be nested. Also, an exception can be thrown again using “throw; ” true or false
8) Exceptions are run-time anomalies or abnormal conditions that a program encounters during its execution
true or false
Instructions:
Exception handing
Add exception handling to this game (try, catch, throw). You are expected to use the standard exception classes to try functions, throw objects, and catch those objects when problems occur.
At a minimum, you should have your code throw an invalid_argument object when data from the user is wrong.
File GamePurse.h:
class GamePurse
{
// data
int purseAmount;
public:
// public functions
GamePurse(int);
void Win(int);
void Loose(int);
int GetAmount();
};
File GamePurse.cpp:
#include "GamePurse.h"
// constructor initilaizes the purseAmount variable
GamePurse::GamePurse(int amount){
purseAmount = amount;
}
// function definations
// add a winning amount to the purseAmount
void GamePurse:: Win(int amount){
purseAmount+= amount;
}
// deduct an amount from the purseAmount.
void GamePurse:: Loose(int amount){
purseAmount-= amount;
}
// return the value of purseAmount.
int GamePurse::GetAmount(){
return purseAmount;
}
File…
Chapter 22 Solutions
C How to Program (8th Edition)
Ch. 22 - Prob. 22.15ECh. 22 - (Catch Parameter) Under what circumstances would...Ch. 22 - (throw Statement) A program contains the statement...Ch. 22 - (Exception Handling vs. Other Schemes) Compare and...Ch. 22 - Prob. 22.19ECh. 22 - Prob. 22.20ECh. 22 - Prob. 22.21ECh. 22 - (Catching Derived-Class Exceptions) Use...Ch. 22 - Prob. 22.23ECh. 22 - Prob. 22.24E
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- (Throwing Exceptions from a catch) Suppose a program throws an exception and the appropriate exception handler begins executing. Now suppose that the exception handler itself throwsthe same exception. Does this create infinite recursion? Write a program to check your observation.arrow_forwarddef test_func(a,b,c): return (a+b)/c This function is normally designed to be used with three numbers: a, b, and c. However, a careless coder may call this function with an ill combination of arguments to cause certain exceptions. Specifically, if any of a, b or c is not a valid number, then this code will produce a TypeError; and if a and b are valid numbers, and c is 0, then the code will produce a ZeroDivisionError. Your job is to enhance this function by adding proper try...except... blocks, surrounding and capturing the exceptions. When a TypeError occurs, instead of crashing, your code must print on the screen: "Code produced TypeError". And, when a ZeroDivisionError occurs, instead of crashing, your code must print on the screen: "Code produced ZeroDivisionError". In both cases, your function will not crash, will not throw an exception, and silently return None.arrow_forwardd) Handle the file while reading with an exception best suited.arrow_forward
- 2. Catching Exceptions Implement Exception handling for the programs you have written in the laboratory exercises as listed below. Note that all data to be used for processing must be input data (data entry from the user). When an invalid data is entered, the program should loop back to data entry until the input becomes valid. Sample Output: run: *******CIRCLE CALCULATOR******* Enter the radius --> a INVALID INPUT! Enter the radius --> ? INVALID INPUT! Enter the radius --> 10 Result: Area:314.0 Diameter:20.0 Circumference:62.800000000000004 BUILD SUCCESSFUL (total time: 20 seconds)arrow_forwardPlease answer all the questions, thank you!arrow_forwardCUSTOM EXCEPTION 1 Donor Eligibility Write a C++ program to handle the exception. Read all the details and check for the eligibilty of the donors. Conditions: Age must be greater than 17. Minimum weight should be greater than 44 kg. Maximum amount of blood can be drawn is 350ml. if any of the conditions are not met then throw an exceptibn Refer the sample input/output below. Input and Output Format: Refer sample input and output for formatting specifications. All text in bold corresponds to input and the rest corresponds to output. [All text in bold corresponds to input and P Type here to searcharrow_forward
- (True/False): In protected mode, each procedure call uses a minimum of 4 bytes of stackspace.arrow_forwardComputer Science Help please! I need my code to use an exception handling method to check if the user entered a correct word. Here is the logic: When given two words, the child may claim that transformation of the first word into the second is possible. In this case, the program asks the child to demonstrate the correctness of the answer by typing a sequence of words starting with the first and ending with the second. Such a sequence is an acceptable proof sequence if each word is obtained from its predecessor by swapping a single pair of adjacent letters. For example, the sequence tops, tosp, tsop, stop, sotp, sopt, spot proves that the word tops can be transformed into spot. If the proof sequence is accepted, the child earns a point and play proceeds to the next round with a fresh pair of words. A proof sequence is rejected if a word cannot be obtained from its predecessor by swapping an adjacent pair of letters, or if the first word in the sequence is not the first word of the pair…arrow_forwardMust throwing an exception cause program termination?arrow_forward
- (Replace strings) Write the following function that replaces the occurrence of a substring old_substring with a new substring new_substring in the string s. The function returns true if string s is changed, and otherwise, it returns false. bool replace_strings (string& s, const string& old_string, const string& new_string) Write a test program that prompts the user to enter three strings, i.e., s, old string, and new_string, and display the replaced string.arrow_forwardOOP question i) Exceptions can be traced and controlled using conditional statements. ii) For critical exceptions compiler provides the handler A - Only (i) is true B - Only (ii) is true C - Both (i) & (ii) are true D - Both (i) && (ii) are falsearrow_forwardTask 4: User Defined Exceptions (Java) A school is enrolling contestants for a competition for students of age 15 and above. Write a Java application to read the ID and age of a student. If the ID does not start with the characters ST or if the age is less than 15, throw a user defined exception InvalidStudentException and display the message “The student is not eligible!!”. If the details entered are valid, display the message “The student is eligible”. Sample Test case: Enter Student ID: ST1500 Enter Age: 14 The student is not eligible!!arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
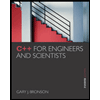
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
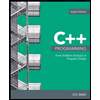
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
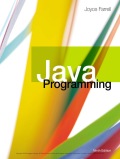
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT