Integer division:
When both operands are integer data types, the resultant statement will be in integer division which means the outcome of the division will also be an integer data type. If there is a remainder, it must be ignored.
Example:
The example for the integer division is as follows:
double value;
value = 5 / 3;
In the above example, the outcome of the “5” by “3” is stored in the variable “value”. The value “1” is stored in the variable “value” because both “5” and “3” are integers, so the decimal part of the result must be truncated.
Floating-point division:
When both operands are floating-point or any one of the operand is a floating-point, the resultant statement will be in floating-point division, which means the outcome of the division will be a floating-point data type.
Example:
The example for the floating-point division is as follows:
double value;
value = 5.0 / 3;
In the above example, the result of the “5.0” by “3” is stored in the variable “value”. The value “1.6667” is stored in the variable “value”, because “5.0” is the floating-point data type and “3” is an integer data type, so the result has a floating-point value.

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
STARTING OUT WITH C++ MPL
- An automated donation machine accepts donations via 10 or 20 bills to a maximum of $990. The machine needs to print a receipt with the donation amount in English, rather than numerically. Complete the following function which converts any number between 10 to 990 dollars to English.NOTE: You may define and use another function if necessary.NOTE: You should use arrays for this task. Use of switch statements or very long if/else if statements for printing is not allowed. However, using if/else if/else statements for the logic of your algorithm is permitted.NOTE: This function returns the result, and does not print it. Make sure the result is not lost when you return. Example function input and output (donation is 110):Input: 110Output: “one hundred ten dollars” string convertToEnglish (int donation); in C++ #include <iostream>#include <string>using namespace std; string convertToEnglish (int donation); int main() {int donation;cin>>donation;…arrow_forwardWrite a function that checks whether an integer is an even digit or an odd digit integer using the following header: int getType(int n)arrow_forwardGiven: int num1, num2, newNum;double x, y;Which of the following assignments are valid? If an assignment is not valid,state the reason.a. num1 = 35;b. newNum = num1 – num2;c. num1 = 5; num2 = 2 + num1; num1 = num2 / 3;d. num1 * num2 = newNum;e. x = 12 * num1 - 15.3;f. num1 * 2 = newNum + num2;g. x / y = x * y;h. num2 = num1 % 2.0;i. newNum = static_cast<int> (x) % 5;j. x = x + y - 5;k. newNum = num1 + static_cast<int> (4.6 / 2);arrow_forward
- Write a program that computes the number of extraterrestrial civilizations capable of communicating with us. - The program should use the following as constants in accordance with Drake's calculations: R. = 1, f = 1, f₁ =1 - The program should prompt the user for the values of every other variable and store those variables as a data-type that makes sense given the type of information that the variable represents. - The program should compute the number of civilizations, N, and write this value to the console window.arrow_forwardIn Java:arrow_forwardC++arrow_forward
- If you have the following function: int Func1(int a, int b) { return a+b; } Which of the following call is correct? Func1(12, 4); Func1(12, "Value2"); O Func1("Value1", "Value2"); O Func1("Values"); O Func1(4);arrow_forwardC programming language questionarrow_forwardjava please Create 2 functions that returns the hundreds andthousands digit from aninteger.int thousandsDigit(int a); // Ex, thousandsDigit(1234) => 1int tensDigit(int a); // Ex, tensDigit(1234) => 3//Use the % operator to return the tenth and thousandth value. %10 and%1000Create a function that counts the number of digits in a positive integer.int countDigits(int a); // Ex, countDigits(1234)=> 4//Convert an integer to String and then returns its length.arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
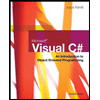
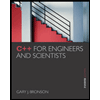
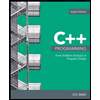