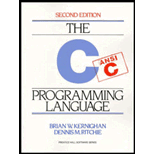
C Programming Language
2nd Edition
ISBN: 9780131103627
Author: Brian W. Kernighan, Dennis M. Ritchie, Dennis Ritchie
Publisher: Prentice Hall
expand_more
expand_more
format_list_bulleted
Question
Chapter 2, Problem 6E
Program Plan Intro
- Initialize the header files and main() function.
- To define the function setbits(x, p, n, y) to return x with the n bits that begin at position p set to the right most n bits of y, leaving the other bits unchanged.
- Finally prints the position.
Summary Introduction- The program displays the x value after applying bitwise operators.
Program Description- The purpose of the program is to define the function setbits(x, p, n, y) to return x with the n bits that begin at position p set to the right most n bits of y, leaving the other bits unchanged.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Design and draw a high-level "as-is" process diagram that illustrates a current process related to a product or service offered through the SSDCI.gov database.
Compare last-mile connections for connecting homes and businesses to the Internet
Explain wireless networking standards
Chapter 2 Solutions
C Programming Language
Ch. 2 - Prob. 1ECh. 2 - Write a loop equivalent to the for loop above...Ch. 2 - Write the function htoi(s), which converts a suing...Ch. 2 - Write an alternate version of squeeze(s1,s2) that...Ch. 2 - Prob. 5ECh. 2 - Prob. 6ECh. 2 - Prob. 7ECh. 2 - Prob. 8ECh. 2 - Prob. 9ECh. 2 - Rewrite the function lower, which converts upper...
Knowledge Booster
Similar questions
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
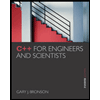
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
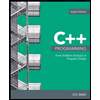
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
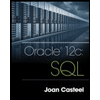
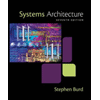
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage