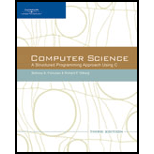
Computer Science: A Structured Programming Approach Using C, Third Edition
3rd Edition
ISBN: 9780534491321
Author: Behrouz A. Forouzan, Richard F. Gilberg
Publisher: Course Technology, Inc.
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 2, Problem 40PS
Write a
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a program that displays a table of pounds and equivalent weights in kilograms. You can find the conversion factor online if necessary. Store this factor in a properly named constant. Allow the user to specify the range of pounds for the table by prompting for the start, stop, and step values for the pounds column. Display the pounds accurate to two decimal places centered in a column 10 characters wide. The kilometers should display accurate to three decimal places and right-aligned in a column 12 characters wide (see page 75-78). Column headings should be displayed with the same alignments. See Sample Output.
Write a program that reads from the user a character (*q' or 'c').
If the character is 'c', the program reads from the user the radius r of a circle and prints its area.
If the user enters 'q', the program asks the user to enter the length and width of a quadrilateral. We
assume the quadrilateral is either a square or rectangle. You should print if the quadrilateral is square
or rectangle.
Otherwise, it prints "Wrong character"
PS: Use the following formulas :
area of circle=3.14*r?
I
Write a program that generates a plate number based on the user's answer to the following questions:
1. What is your favorite color of the rainbow (ROYGVIB)?
a. Use the first letter of the chosen color as the first character of the plate number.
b. Display "Invalid Input" and ask the user to re-enter his/her answer if the answer is not a valid color of the rainbow.
2. What is your month of birth?
a.
Use the last letter of the month as the second character of the plate number.
b. Display "Invalid Input" and ask the user to re-enter his/her answer if the answer is not a valid month.
3. What is your first name?
a. Use the third letter of the first name as the third character of the plate number. No need to include the second name if you have a second name.
Make sure to use "cout" and "cin" to collect the necessary inputs from the user.
For the number section:
1. Ask the user his/her age and use the age as the first and second digits of the plate number.
a. Display "Invalid Input" and ask…
Chapter 2 Solutions
Computer Science: A Structured Programming Approach Using C, Third Edition
Ch. 2 - The purpose of a header file, such as stdio.h, is...Ch. 2 - Prob. 2PSCh. 2 - The C standard function that receives data from...Ch. 2 - Which of the following statements about the...Ch. 2 - Which of the following statements about block...Ch. 2 - Prob. 6PSCh. 2 - Which of the following is not a data type?...Ch. 2 - The code that establishes the original value for a...Ch. 2 - Prob. 9PSCh. 2 - Prob. 10PS
Ch. 2 - To print data left justified, you would use a in...Ch. 2 - Prob. 12PSCh. 2 - One of the most common errors for new programmers...Ch. 2 - Which of the following is not a character constant...Ch. 2 - Which of the following is not an integer constant...Ch. 2 - Which of the following is not a floating-point...Ch. 2 - Prob. 17PSCh. 2 - Which of the following is not a valid identifier...Ch. 2 - What is the type of each of the following...Ch. 2 - What is the type of each of the following...Ch. 2 - Which of the following identifiers are valid and...Ch. 2 - Which of the following identifiers are valid and...Ch. 2 - What is output from the following program...Ch. 2 - Prob. 24PSCh. 2 - Find any errors in the following program....Ch. 2 - Find any errors in the following program....Ch. 2 - Prob. 27PSCh. 2 - Prob. 28PSCh. 2 - Prob. 29PSCh. 2 - Code the variable declarations for each of the...Ch. 2 - Code the variable declarations for each of the...Ch. 2 - Write a statement to print the following line....Ch. 2 - Write a program that uses four print statements to...Ch. 2 - Write a program that uses four print statements to...Ch. 2 - Write a program that uses defined constants for...Ch. 2 - Prob. 36PSCh. 2 - Prob. 37PSCh. 2 - Write a program that prompts the user to enter an...Ch. 2 - Write a C program using printf statements to print...Ch. 2 - Write a program that reads a character, an...Ch. 2 - Write a program that prompts the user to enter...Ch. 2 - Write a program that reads 10 integers and prints...Ch. 2 - Write a program that reads nine integers and...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a program that prompts the user to input two POSITIVE numbers — a dividend (numerator) and a divisor (denominator). Your program should then divide the numerator by the denominator, and display the quotient followed by the remainder.arrow_forwardHere is a classic “introduction to computer programming” problem: Write a program that converts temperature from Fahrenheit to Celsius and vice versa. The program will accept a number (temperature) and a temperature unit designation (i.e., character F or C) from the user, and then convert the temperature into the other unit system. The program will echo the input as part of the final conversion output. The temperature units should be printed in full (Fahrenheit or Celsius). Your program should use real numbers—not integers—for both input and output. Formula: T(F) = (T(C) * 9 / 5) + 32T(C) = (T(F) − 32 ) * 5 / 9 Sample Run: This program converts Temperatures from Fahrenheit to Celsius and vice versa. Please enter your temperature: 32 Please enter the units (F/C): F A temperature of 32 degrees Fahrenheit is equivalent to 0 degrees Celsius. Do you wish another conversion? (Y/N): Y Please enter your temperature: 100 Please enter the units (F/C): C A temperature of 100 degrees Celsius is…arrow_forwardWrite a program that prompts the user to enter the students’ names and their scores on one line, and prints student names in increasing orderof their scores.arrow_forward
- Write a program that takes your full name as input and displays the abbreviations ofthe first and middle names except the last name which is displayed as it is. For example, if your name is Robert Brett Roser, then the output should be R.B.Roser.arrow_forwardwrite a program that reads in an integer value between 1 and 5(inclusive) and a text word from the keyboard and prints out whethe the two represent the same number. For exemple: Enter integer value: 4 Enter word: four 4 and four are the same number. or Enter integer value: 4 Enter word: five 4 and five are not the same number. you may assume that the user will only enter integer values between 1 and 5 (inclusive). only use conditional statement like if, else, elif.arrow_forwardProcedure 2. Write a program that takes the x-y coordinates of a point in the Cartesian plane and prints a message telling either an axis on which the point lies or the quadrant in which it is found. If x or y is 0, the code will print out "The point is on x or y axis". For example, if your input x is 2.5, and y is 1, it will print out "The point is in Quadrant One"; if the input x is -2.3, and y is -3.2, it will print out "The point is in Quadrant Three"; if the input x is 0, and y is 20, it will print out "The point is on x or y axis ". Requirements: 1. The inputs of x and y should be float data type. 2. The input should prompt a message on the screen to ask for the input x and y.arrow_forward
- Write a python program that does the following: 1. prompts the user to enter a word 2. using that word, first prints out the word where all characters are replaced by “_”’s (there will be an example to better illustrate this below). 3. the program will continue to print the word revealing one character at a time. i.e., The second line printed should print the first character followed by “_”’s representing the rest of the word. 4. The program should end after printing the entire word once. example Enter a word: kangaroo ________ k_______ ka______ kan_____ kang____ kanga___ kangar__ kangaro_ kangarooarrow_forward6. A palindrome is a number or text phrase that reads the same backwards or forwards. For example, each of the following five-digit integers is a palindrome: 12321, 55555, 45554 and 11611. Write a program that reads a 5 digit integer and determines whether it is a palindrome. Hint: One way to do this is to use division (/) and modulus (%) operators to separate the number into individual digits.arrow_forwardWrite a program that takes a positive integer as the height of the triangle and prints an alphabetical triangle, using lowercase English letters as shown below. Each subsequent row of the triangle should increase in length by two letters. Make sure your program validates the user input. If the user does not input a positive integer, print "Invalid input."arrow_forward
- Write a python program that draws a rectangle filled with random colors horizontally. Let the user enter the side and width of the rectangle (hint: use a random number generator to set the pen rgb color value).arrow_forwardWrite a program that reads a four-digit integer, such as 2014, and then displays it, one digit per line in reverse order, like so: 4 1 0 2 Your prompt should tell the user to enter a four-digit integer. You can then assume that the user follows directions. (Hint: Use the division andm remainder operators.)arrow_forwardThis is the question - Write a program that inserts parentheses, a space, and a dash into a string of 10 user-entered numbers to format it as a phone number. For example, 5153458912 becomes (515) 345-8912. If the user does not enter exactly 10 digits, display an error message. Continue to accept user input until the user enters 999. This is the code I have - However it isn't seeming to accept my output. It doesn't like what I have for some reason - import java.util.*; public class PhoneNumberFormat { public static void main(String[] args) { // Write your code here //Create an instance of Scanner class Scanner keyboard=new Scanner(System.in); //declare string data type variables String phoneNumber= ""; String formattedPhoneNumber=""; /*Run the loop infinitely until user enters 999 to exit from program*/ while(true) { System.out.println("Enter phone number: "); phoneNumber=keyboard.nextLine(); //exit…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
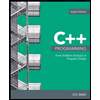
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
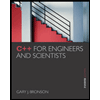
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Control Structure in Data Structure - Data Structures - Computer Science Class 12; Author: Ekeeda;https://www.youtube.com/watch?v=9FTw2pXLhv4;License: Standard YouTube License, CC-BY