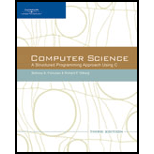
Concept explainers
Find any errors in the following

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Computer Science: A Structured Programming Approach Using C, Third Edition
- Hi I need help with this it is printing out YEAR instead of the answer // This program calculates your age in the year 2050.// Input: None.// Output: Your current age followed by your age in 2050. public class NewAge2{ public static void main(String args[]) { int currentAge = 32; int newAge; int currentYear = 2022; // Declare a constant named YEAR and initialize it to 2050 final int YEAR = 2050; // Edit this statement so that it uses the constant named YEAR. newAge = currentAge + ( YEAR - currentYear ); System.out.println( " My Current Age is " + currentAge); // Edit this output statement so that is uses the constant named YEAR. System.out.println(" I will be " + newAge + " in + YEAR "); System.exit(0); }}arrow_forwardIdentify the errors in this code and how to fix them. #Arguments i-current e-Voltage #Compute the resistance using i=e/r def main(): voltage1=float(input("Please enter the voltage of circuit 1 :")) current1=float(input("Please enter the current of 1 :")) voltage2=float(input("\nPlease enter the voltage of circuit 2 :")) current2=float(input("Please enter the voltage of current 2 :")) voltage3=float(input("\nPlease enter the voltage of circuit 3 :")) current3=float(input("Please enter the current of circuit 3 :")) r1=resistance(i1,e1) r2=resistance(i2,e2) r3=resistance(i3,e3) rs=series_resistance(r1,r2,r3) rp=parallel_resistance(r1,r2,r3) print("\n In a parallel circuit arrangement the total current would be:%.2f'%rp) print('Resistance in Series Circuit :%.2f'%rs) def resistance(i,e): return e/i #Compute the total resistance as per the given formula def parallel_resistance(r1,r2,r3): return 1.0/((1.0/r1)+(1.0/r2)+(1.0/r3)) #Compute the series resistance…arrow_forwardConvert totalMeters to hectometers, decameters, and meters, finding the maximum number of hectometers, then decameters, then meters. Ex: If the input is 815, then the output is: Hectometers: 8 Decameters: 1 Meters: 5 Note: A hectometer is 100 meters. A decameter is 10 meters. 1 #include 2 using namespace std; 3 4 int main() { 5 6 7 8 9 10 11 12 13 14 15 16 17 18 int totalMeters; int numHectometers; int numDecameters; int numMeters; cin >>totalMeters; cout << "Hectometers: cout << "Decameters: cout << "Meters: " << numMeters << endl; " << numHectometers << endl; << numDecameters << endl; 2 3arrow_forward
- Language: Python Write a program that computes and displays the charges for a patient's hospital stay. First, the program should ask if the patient was admitted as an in-patient or an out-patient. If the patient was an in-patient the following data should be entered: - The number of days spent in the hospital - The daily rate -Charges for hospital services (lab tests, etc.) -Hospital medication charges If the patient was an out-patient the following data should be entered: -Charges for hospital services (lab tests, etx.) -Hospital medication charges Use a single, seperate function to validate that no input is less than zero. If it is, it should be re-entered before being returned. Once the required data has been input and validated, the program should use two seperate functions to calculate the total charges. One of the functions should accept arguments for the in-patient data, which the other function accepts arguments for out-patient data. Both functions should return the total…arrow_forwardComplete the following methods:arrow_forwardFunctions Exercise (Parking Charges) A parking garage charges a $2.00 minimum fee to park for one hour. The maximum charge for any given 24-hour period is $10.00. Assume that no car parks for longer than 24 hours at a time. Write a program that calculates and prints the parking charges for each of three customers who parked their cars in this garage yesterday. You should enter the hours parked for each customer. Your program should print the results in a neat tabular format and should calculate and print the total of yesterday's receipts. The program should use the function Charge to determine the charge for each customer. Car Hours Charge 1 2 Computer skills (Engineering) 3 1 4 24 TOTAL 2 8 10 20arrow_forward
- Assignment: Carefully read the instructions and write a program that reads the following information and prints a payroll statement. Employee’s name (e.g., Smith)Number of hours worked in a week (e.g., 10)Hourly pay rate (e.g., 9.75)Federal tax withholding rate (e.g., 20%)State tax withholding rate (e.g., 9%) In summary, design a program to• Prompt user for 5 values and read the values using Scannero Use method .nextLine() to get the String for the nameo Use method .nextDouble() to get all other numeric values• Calculating the Gross payo Gross pay = hours worked * hourly pay rate• Calculating the Federal withholdingo Federal withholding = Gross pay * federal tax withholding rate• Calculating the State withholdingo State withholding = Gross pay * state tax withholding rate• Calculating the Total deductiono Total deduction = Federal withholding + State withholding• Calculating the Net Payo Net Pay = Gross pay – Total deduction• Formatting the output same as the Sample run (Use ONLY…arrow_forwardThe correct declaration statement is: int X; Y; int X, Y; int X, Y int X, Y,arrow_forwardi. Find the loop invariant for the program code below. void main() { int y = 1, x = 0; while (x < 4) { y = y * z; x = x + 1; }arrow_forward
- // SuperMarket.java - This program creates a report that lists weekly hours worked // by employees of a supermarket. The report lists total hours for // each day of one week. // Input: Interactive // Output: Report. import java.util.Scanner; public class SuperMarket { public static void main(String args[]) { // Declare variables. final String HEAD1 = "WEEKLY HOURS WORKED"; final String DAY_FOOTER = " Day Total "; // Leading spaces are intentional. final String SENTINEL = "done"; // Named constant for sentinel value. double hoursWorked = 0; // Current record hours. String hoursWorkedString = ""; // String version of hours String dayOfWeek; // Current record day of week. double hoursTotal = 0; // Hours total for a day. String prevDay = ""; // Previous day of week. boolean done = false; // loop control Scanner input = new…arrow_forwardLanguage: JAVA Leap-Year Write a program to find if a year is a leap year or not. We generally assume that if a year number is divisible by 4 it is a leap year. But it is not the only case. A year is a leap year if − It is evenly divisible by 100 If it is divisible by 100, then it should also be divisible by 400 Except this, all other years evenly divisible by 4 are leap years. So the leap year algorithm is, given the year number Y, Check if Y is divisible by 4 but not 100, DISPLAY "leap year" Check if Y is divisible by 400, DISPLAY "leap year" Otherwise, DISPLAY "not leap year" For this program you have to take the input, that is the year number from an input file input.txt that is provided to you. The input file contains multiple input year numbers. Use a while loop to input the year numbers from the input file one at a time and check if that year is a leap year or not. Create a class named LeapYear which will contain the main method and write all your code in the main…arrow_forwardPlease explain this question void main() {int a =300; char *ptr = (char*) &a ; ptr ++; *ptr =2; printf("%d", a); }arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
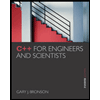