Concept explainers
Explanation of Solution
using namespace std;
include <#ioStream>
The preprocessor commands are processed by the preprocessor before the program goes through the compiler. To use cin and cout, the program must include the header file iostream and either include the statement using namespace std; or refer to these identifiers as std::cin and std::cout. Therefore, the above statements are in the wrong order. The include preprocessor command should precede the using statement. Additionally, there are multiple errors in the include preprocessor command. All preprocessor commands start with the symbol # and the correct header file name is iostream and not ioStream (C++ is a case sensitive language). Correct statements:
#include <iostream>
using namespace std;
int main() {
(no errors)
int num1; num2;
The identifier num2 appears after the statement terminator semi-colon. Hence, the semicolon should be replaced by a comma. Correct statement;
int num, num2;
string str1;
(no errors)
cout << "Enter a string without any blanks in it ": ;
There is a colon which is intended to be part of the output string but appears after the string terminator double quotation marks. Correct statement:
cout << "Enter a string without any blanks in it :" ;
cin >> string
Multiple errors - instead of using the identifier str1, the data type string has been placed to receive the input. Also the statement terminator is missing. Correct statement:
cin >> str1;
cout << endl;
cout << "Enter two integers: ";
(no errors)
cin << num1, num2;
The first stream operator should be an extraction operator which is >> and the second stream extraction operator is missing altogether and a comma has been used instead. Correct statement:
cin >> num1 >> num2;
cout << endl;
(no errors)
return 0;
The return statement should be the last statement in any function and in this case, the main function...

Trending nowThis is a popular solution!

Chapter 2 Solutions
EBK MINDTAPV2.0 FOR MALIK'S C++ PROGRAM
- #include using namespace std; int main() int x=1,y=2; for (int i=0; i<3; i++) e{ x=x*y; 8{ } cout<arrow_forwardC++ PROGRAMMING Create a C++ program that accepts an English sentence and converts it to Pig Latin. The rules of Pig Latin are as follows: If the word starts with a vowel, add the word "yay" in the end. If the word starts with a consonant, move the first letter of the word in the end and add "ay" in the end. If the word starts with a capital letter, follow the rule and convert the first letter of the converted Pig Latin word into a capital letter. (i.e. Hello -> ElloHay) If there are numbers in the sentence, they should not be translated. A word with a combination of letters and numbers will not be tested. Spacing and punctations must be preserved. Sample Input: A quick brown fox jumps over the 100 lazy dogs. Expected Output: Ayay uickqay rownbay oxfay umpsjay overyay hetay 100 azylay ogsday.arrow_forward8. Sentence Capitalizer Write a program with a function that accepts a string as an argument and returns a copy of the string with the first character of each sentence capitalized. For instance, if the argu- ment is “hello. my name is Joe. what is your name?" the function should return the string "Hello. My name is Joe. What is your name?" The program should let the user enter a string and then pass it to the function. The modified string should be displayed.arrow_forwardCreate the following code in C++ DESIGN and IMPLEMENT a short program that will: Allow the user to enter a string with up to 100 letters. Display the user-entered string: Forward Backward Vertical As a triangle made from the letters of the string Display the number of letters in the string. Once everything above is displayed, the program will ask the user if he or she wishes to enter a different string or quit.arrow_forwardC++arrow_forwardlanguage C++arrow_forwardComputer Science Programming question C++ Codearrow_forwardAssignment#1-Part-2write a c# program which takes 2 inputs for alphabet(sigma) and your program will generate different combinations/ concatenation string(10 length). Then you give the string as a input, so your program validates/match this string with your generated-concatenation-string by using Regular-Expression.arrow_forwardIn C++ struct gameType { string title; int numPlayers; bool online; }; gameType Awesome[50]; Write the C++ Write the C++ statements that correctly initialize the online value of each game in Awesome to 1.arrow_forwardC++ programarrow_forwardNumber guessing Game Write a C program that implements the “guess my number” game. The computer chooses a random number using the following random generator function srand(time(NULL)); int r = rand() % 100 + 1; that creates a random number between 1 and 100 and puts it in the variable r. (Note that you have to include <time.h>) Then it asks the user to make a guess. Each time the user makes a guess, the program tells the user if the entered number is larger or smaller than its number. The user then keeps guessing till he/she finds the number. If the user doesn’t find the number after 10 guesses, a proper game over message will be shown and the actual guess is revealed. If the user makes a correct guess in its allowed 10 guesses, then a proper message will be shown and the number of guesses the user made to get the correct answer is also printed. After each correct guess or game over, the user decides to play again or quit and based on the user choice, the computer will make…arrow_forward7bact1 Please help me answer this in python programming.arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
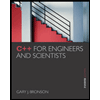
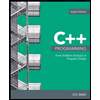