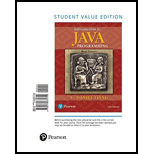
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
11th Edition
ISBN: 9780134671710
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 19.7, Problem 19.7.3CP
Program Plan Intro
Given generic method in “Listing 19.9”:
The given generic method “add()” which has generic type “<T>” as arguments “stack1” and “stack2” for “GenericStack” class.
//Given Generic method
public static <T> void add(GenericStack<T> stack1, GenericStack<? super T> stack2)
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write the SQL code that permits to implement the tables: Student and Transcript. NB: Add the constraints on the attributes – keys and other.
Draw an ERD that will involve the entity types: Professor, Student, Department and Course. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Draw an ERD that represents a book in a library system. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Chapter 19 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
Ch. 19.2 - Are there any compile errors in (a) and (b)?Ch. 19.2 - Prob. 19.2.2CPCh. 19.2 - Prob. 19.2.3CPCh. 19.3 - Prob. 19.3.1CPCh. 19.3 - Prob. 19.3.2CPCh. 19.3 - Prob. 19.3.3CPCh. 19.3 - Prob. 19.3.4CPCh. 19.4 - Prob. 19.4.1CPCh. 19.4 - Prob. 19.4.2CPCh. 19.5 - Prob. 19.5.1CP
Ch. 19.5 - Prob. 19.5.2CPCh. 19.6 - What is a raw type? Why is a raw type unsafe? Why...Ch. 19.6 - Prob. 19.6.2CPCh. 19.7 - Prob. 19.7.1CPCh. 19.7 - Prob. 19.7.2CPCh. 19.7 - Prob. 19.7.3CPCh. 19.7 - Prob. 19.7.4CPCh. 19.8 - Prob. 19.8.1CPCh. 19.8 - Prob. 19.8.2CPCh. 19.8 - Prob. 19.8.3CPCh. 19.8 - Prob. 19.8.4CPCh. 19.8 - Prob. 19.8.5CPCh. 19.9 - Prob. 19.9.1CPCh. 19.9 - How are the add, multiple, and zero methods...Ch. 19.9 - How are the add, multiple, and zero methods...Ch. 19.9 - What would be wrong if the printResult method is...Ch. 19 - (Revising Listing 19.1) Revise the GenericStack...Ch. 19 - Prob. 19.2PECh. 19 - (Distinct elements in ArrayList) Write the...Ch. 19 - Prob. 19.4PECh. 19 - (Maximum element in an array) Implement the...Ch. 19 - (Maximum element in a two-dimensional array) Write...Ch. 19 - Prob. 19.7PECh. 19 - (Shuffle ArrayList) Write the following method...Ch. 19 - (Sort ArrayList) Write the following method that...Ch. 19 - (Largest element in an ArrayList) Write the...Ch. 19 - Prob. 19.11PE
Knowledge Booster
Similar questions
- 2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forward
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
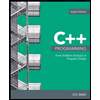
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
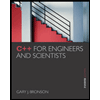
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
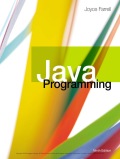
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
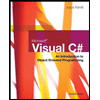
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage