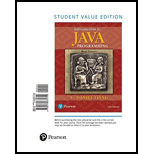
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
11th Edition
ISBN: 9780134671710
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 19, Problem 19.4PE
Program Plan Intro
Generic linear search
Program Plan:
- Define the class named “Exercise19_04”.
- Define the generic method named “linearSearch()” which passes array list and key as arguments.
- Using “for” loop, check the list values equal to key value.
- If the condition is true, return position of the key value.
- Otherwise, return “-1” to calling function.
- Using “for” loop, check the list values equal to key value.
- Define the main method.
- Declare and initialize an array “arr[]” in type of integer.
- Call the generic method “linearSearch()” with array and key elements. Print the resultant positions on screen.
- Define the generic method named “linearSearch()” which passes array list and key as arguments.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Do not use 2d lists, sets, dicts, arrays or recursion.
Code in Python.
Write the function destructiveRemoveRepeats(L), whichtakes a list L and destructively returns a new list in which any repeating elements in L are removed. Thus, this function should directly modify the provided list to not have any repeating elements. Since this is a destructive function, it should not return any value at all (so, implicitly, it should return None).
For example:
L = [1, 3, 5, 3, 3, 2, 1, 7, 5] destructiveRemoveRepeats(L) assert(L == [1, 3, 5, 2, 7])
Go program using generics
Output
True
False
John here
Need correct code for the following question
Chapter 19 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
Ch. 19.2 - Are there any compile errors in (a) and (b)?Ch. 19.2 - Prob. 19.2.2CPCh. 19.2 - Prob. 19.2.3CPCh. 19.3 - Prob. 19.3.1CPCh. 19.3 - Prob. 19.3.2CPCh. 19.3 - Prob. 19.3.3CPCh. 19.3 - Prob. 19.3.4CPCh. 19.4 - Prob. 19.4.1CPCh. 19.4 - Prob. 19.4.2CPCh. 19.5 - Prob. 19.5.1CP
Ch. 19.5 - Prob. 19.5.2CPCh. 19.6 - What is a raw type? Why is a raw type unsafe? Why...Ch. 19.6 - Prob. 19.6.2CPCh. 19.7 - Prob. 19.7.1CPCh. 19.7 - Prob. 19.7.2CPCh. 19.7 - Prob. 19.7.3CPCh. 19.7 - Prob. 19.7.4CPCh. 19.8 - Prob. 19.8.1CPCh. 19.8 - Prob. 19.8.2CPCh. 19.8 - Prob. 19.8.3CPCh. 19.8 - Prob. 19.8.4CPCh. 19.8 - Prob. 19.8.5CPCh. 19.9 - Prob. 19.9.1CPCh. 19.9 - How are the add, multiple, and zero methods...Ch. 19.9 - How are the add, multiple, and zero methods...Ch. 19.9 - What would be wrong if the printResult method is...Ch. 19 - (Revising Listing 19.1) Revise the GenericStack...Ch. 19 - Prob. 19.2PECh. 19 - (Distinct elements in ArrayList) Write the...Ch. 19 - Prob. 19.4PECh. 19 - (Maximum element in an array) Implement the...Ch. 19 - (Maximum element in a two-dimensional array) Write...Ch. 19 - Prob. 19.7PECh. 19 - (Shuffle ArrayList) Write the following method...Ch. 19 - (Sort ArrayList) Write the following method that...Ch. 19 - (Largest element in an ArrayList) Write the...Ch. 19 - Prob. 19.11PE
Knowledge Booster
Similar questions
- Write a Python Code for the given function and conditions: (Use LinkedList Manipulation) Given function: def remove(self, deletekey) (4) Pre-condition: List is not empty. Post-condition: Removes the element from a list that contains the deleteKey and returns the deleted key value.arrow_forwardFind the error in each of the segments. If the error can be corrected, explain how ? short* numPtr, result;void* genericPtr{numPtr};result = *genericPtr + 7;arrow_forwardC++arrow_forward
- java program java method: Write a method replace to be included in the class KWLinkedList (for doubly linked list) that accepts two parameters, searchItem and repItem of type E. The method searches for searchItem in the doubly linked list, if found then replace it with repItem and return true. If the searchItem is not found in the doubly linked list, then insert repItem at the end of the linked list and return false. Assume that the list is not empty. You can use ListIterator and its methods to search the searchItem in the list and replace it with repItem if found. Do not call any method of class KWLinkedList to add a new node at the end of the list. Method Heading: public boolean replace(E searchItem, E repItem) Example: searchItem: 15 repItem: 17 List (before method call): 9 10 15 20 4 5 6 List (after method call) : 9 10 17 20 4 5 6arrow_forwarddef remove_occurences[T](xs:List[T], elem: T, n:Int) : List[A] = {} Using the function above, can you show me how to make a polymorphic function in scala where the program removes elem , n number of times from the list. If n > elem then remove all elem from the list For example: remove_occurences(List(4,5,6,7,4),4,1) -> List(5,6,7,4)remove_occurences(List(4,5,6,7,4),4,2) -> List(5,6,7)remove_occurences(List(4,5,6,7,4),2,2) -> List(4,5,6,7,4)arrow_forwardDirection: Continue the code below and add case 4, case 5, and case 6. Add 3 more functions aside from insert, getValue, and clear from List ADT import java.util.LinkedList; import java.util.Scanner; class SampleLL { } public static void main(String[] args) { LinkedList 11s = new LinkedList(); String msg = "Choose a function: \n [1] Insert, [2]Get Value, [3]Clear, [0] Exit"; System.out.println(msg); Scanner scan= new Scanner(System.in); int choice scan.nextInt (); while(true) { } if (choice =0) { } System.exit(0); switch(choice) { } case 1: System.out.println("Enter a word/symbol:"); break; case 2: System.out.println("Enter a number: "); break; 11s.add(scan.next()); break; case 3 11s.clear(); default: System.out.println("Invalid input!"); break; System.out.println(11s.get (scan.nextInt())); System.out.println(msg); choice scan.nextInt ();arrow_forward
- Data Structure and algorithms ( in Java ) Please solve it urgent basis: Make a programe in Java with complete comments detail and attach outputs image: Question is inside the image also: a). Write a function to insert elements in the sorted manner in the linked list. This means that the elements of the list will always be in ascending order, whenever you insert the data. For example, After calling insert method with the given data your list should be as follows: Insert 50 List:- 50 Insert 40 List:- 40 50 Insert 25 List:- 25 40 50 Insert 35 List:- 25 35 40 50 Insert 40 List:- 25 35 40 40 50 Insert 70 List:- 25 35 40 50 70 b). Write a program…arrow_forwardDescribe the HeapAlloc function.arrow_forwardCan you help me write a C++ Program to do the following: Create a generic function increment(start, stop, x) thatadds x to every element in the range [start,stop). The addition isdone using the + operator. The arguments start and stop are bidirectional iterators. Write a test driverarrow_forward
- Exercise G -- Implement a function halves that takes a list of integers and divides each element of the list by two (using the integer division operator //) NOTE use the map function combined with a lambda expression to do the division with a neat solution halves : List Int -> List Int halves xs = [ ] --remove this line and implement your halves function herearrow_forwardThe statement "Linear collection of self-referential class objects, called nodes, connected by pointer links" is best describing a/an 7. O A. data structure B. union O c. array list O D. linked listarrow_forwardC++ Create a generic function increment(start, stop, x) that adds x to every element in the range [start,stop). The addition is done using the + operator. The arguments start and stop are bidirectional iterators. Write a test driver.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
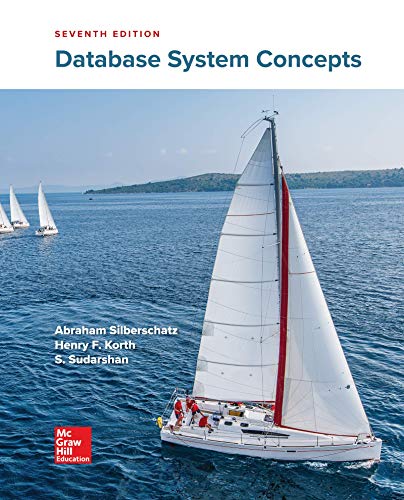
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
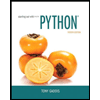
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
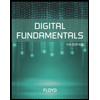
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
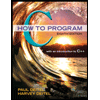
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
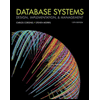
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
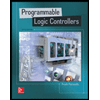
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education