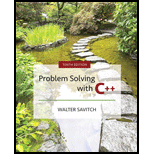
Sorting of ten numbers
Program Plan:
- Include required header file.
- Include required “std” namespace.
- Define main function
- Declare “deque” variable to store the numbers in “double” data type.
- Declare “deque” variable to store the result in “double” data type.
- Declare a variable “values” in “double” data type.
- Display prompt statement.
- Read ten numbers from user and then store in “deque” using “push_back()” function.
- Before sorting, display the ten numbers using “for” loop.
- Then sort the ten numbers using generic “sort” function.
- Finally display the sorted numbers using “for” loop.

The below C++ program is used to sorts the ten “double” numbers in the “deque” using the generic “sort” function.
Explanation of Solution
Program:
//Header file
#include <iostream>
#include <deque>
#include <algorithm>
//Std namespace
using std::cout;
using std::cin;
using std::endl;
using std::deque;
using std::sort;
//Main function
int main()
{
/* Declare deque to store the numbers in "double" type */
deque<double> numbers;
/* Declare deque to iterator */
deque<double>::iterator result;
/* Declare "values" in "double" data type */
double values;
/* Display prompt statement */
cout << "Enter ten numbers" << endl;
/*Read ten numbers */
for(int i = 0; i < 10; i++)
{
cin>>values;
/* Store the ten numbers in deque */
numbers.push_back(values);
}
/* Display statement */
cout << "Before sorting, the ten double numbers are " << endl;
/* Display numbers before sorting */
for(result = numbers.begin(); result != numbers.end();result++)
cout << *result << endl;
/* Sort the numbers in "deque" using "sort" function */
sort(numbers.begin(), numbers.end());
/* Display statement */
cout << "After sorting, the ten double numbers are " << endl;
/* Display sorted numbers */
for(result = numbers.begin(); result != numbers.end();result++)
cout << *result << endl;
return 0;
}
Enter ten numbers
40
30.12
12
10
32.10
54.6
80
15.8
98.4
34
Before sorting, the ten double numbers are
40
30.12
12
10
32.1
54.6
80
15.8
98.4
34
After sorting, the ten double numbers are
10
12
15.8
30.12
32.1
34
40
54.6
80
98.4
Want to see more full solutions like this?
Chapter 18 Solutions
Problem Solving with C++ (10th Edition)
- Answer in C++ Programming Language. C++, Array Write a program that will ask the user to enter a set of numbers and outputs all the subsets of that set. The size of the array is 5. Ask the user if he wants to repeat the program by pressing y/Y.arrow_forwardWrite a program that defines a multidimensional array $clubs that stores information about four football clubs, including the name, and the year founded. The program should print the contents of the array using a foreach loop. The program should also sort the array by the club name using the sort() function. Finally, it should print the sorted array.Complete the code:<?php// Define a multidimensional array$clubs = array(array("Newcastle United","England", 1892),array("Gezira Sporting Club", "Egypt", 1882),array("Zamalek Sporting Club", "Egypt", 1911),array("Bidvest Wits South Africa", "Egypt", 1921));// Print the contents of the arrayecho "List of football clubs:\n";foreach ( //Add code) {//Add code}// Sort the array by the first columnsort(//Add code);// Print the sorted arrayprint_r(//Add code);?>arrow_forwardProblem Attachedarrow_forward
- In C++ Sort an array from largest to smallest (you may use one of the sorts we did, you just have to change it so that it goes from largest to smallest instead).arrow_forwardYou can shuffle a list using random.shuffle(lst). Write your own function without using random.shuffle(lst) to shuffle a list and return the list. Use the following function header:def shuffle(lst):Write a test program that prompts the user to enter a list of numbers, invokes the function to shuffle the numbers, and displays the numbers.arrow_forwardplease use pythonarrow_forward
- in c++ vs2019 You should write a program to sort an array. For this purpose you will implement two sorting algorithms: Insertion Sort and Merge Sort. For each algorithm, you are supposed to have a counter and count how many times a comparison is made to sort the array. Once this logic is implemented, create integer arrays of 1000 and 10000 elements with random values inside (you can implement a random number generator for this purpose and randomize their elements). Then go ahead and run your application. The output should show us how many comparisons it made for each algorithm for 1000 and 10000 elements. Do it seperate files. 1 cpp file 1 header file 1 main.cpp filearrow_forwardProgramming in C language.arrow_forwardIn C++.arrow_forward
- use c language You wish to create a simple educational game for pre-school children to learn number sorting of either descending or ascending. Your program only supports 2-number sorting. Firstly, the program asks user for a preference, either descending (enter ‘d’ or ‘D)’ or ascending (enter’a’ or ‘A’). Then, it asks user to enter 2 numbers to sort and stores the 2 numbers in an array. Then, the program sort the numbers in ascending and descending order and store the sorted numbers (large, small) in SEPARATE array. Finally, it displaysarrow_forwardGive me answer fast please.arrow_forwardUsing JavaScriptDefine a function getMonth which accepts number from 1 to 12 as an argument and return the descriptive name of the month. For example: getMonth(1) should return January while getMonth(12) returns December, finally getMonth(-1) returns null. Use array or object to define a list of names for the month and refrain from using if statement to check the argument if it's 1, 2, etc.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
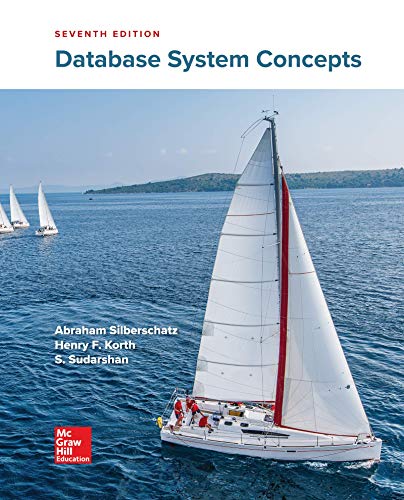
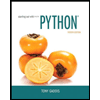
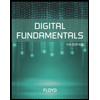
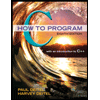
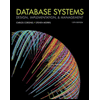
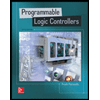