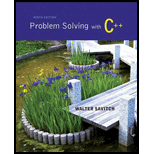
Problem Solving with C++ (9th Edition)
9th Edition
ISBN: 9780133591743
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 17.1, Problem 6STE
Program Plan Intro
Function template:
In C++, a function template is referred as a “generic” function, which can work with different data types.
- While writing a function template, a programmer should use a “type parameter” to denote a “generic” data type instead of using the actual parameter.
- The compiler generates the code, when it encounters a function call to a function template. This code will handle the particular data type which is used in the function call.
- The compiler identifies the argument type and generates the code to work with those types.
- The generated code is referred as “template function”.
Example:
For example consider the following function template for finding a cube of value:
template <class T>
T cube(T x)
{
return x * x * x ;
}
- A function template must begin with the keyword “template” and it is followed by a pair of angle brackets, which contains one or more “generic” data types.
- A “generic” type must start with the keyword “class”, followed by an argument name which stands for the data type.
- The statement “T cube(T x)” is referred as function header, where “T” is a “type parameter”, “cube” is the function name and the variable “x” is declared for the type “T”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Information Security Risk and Vulnerability Assessment
1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset?
Please do not use AI and add refrence
Find the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714V
Resolver por superposicion
Chapter 17 Solutions
Problem Solving with C++ (9th Edition)
Ch. 17.1 - Write a function template named maximum. The...Ch. 17.1 - Prob. 2STECh. 17.1 - Define or characterize the template facility for...Ch. 17.1 - Prob. 4STECh. 17.1 - Display 7.10 shows a function called search, which...Ch. 17.1 - Prob. 6STECh. 17.2 - Give the definition for the member function...Ch. 17.2 - Give the definition for the constructor with zero...Ch. 17.2 - Give the definition of a template class called...Ch. 17.2 - Is the following true or false? Friends are used...
Ch. 17 - Write a function template for a function that has...Ch. 17 - Prob. 2PCh. 17 - Prob. 3PCh. 17 - Redo Programming Project 3 in Chapter 7, but this...Ch. 17 - Display 17.3 gives a template function for sorting...Ch. 17 - (This project requires that you know what a stack...Ch. 17 - Prob. 6PPCh. 17 - Prob. 7PPCh. 17 - This project requires that you complete...
Knowledge Booster
Similar questions
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
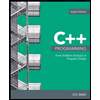
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
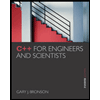
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage