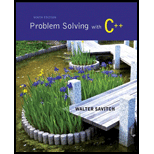
- Include required library files.
- Define a class named “NegativeAmount” and “InsufficientFunds”.
- Inside the “Account” class,
- Add the negative amount exception in the “deposit()” function.
- Add the negative amount and insufficient funds exception in the “withdraw()” function.
- Define a “main()” function to test the program to deposit and withdraw amounts.
- Create an object and test the deposit and withdraw using the appropriate function.
- Then print the result.
- Add the negative amount exception in the “deposit()” function.
- Add the negative amount and insufficient funds exception in the “withdraw()” function.
- Create an object and test the deposit and withdraw using the appropriate function.
- Then print the result.

Program to rewrite the given class “Account” that throws an appropriate exceptions and test the program to deposit and withdraw amounts are as follows,
Explanation of Solution
// Include required library files
#include <iostream>
using namespace std;
// Definition of class NegativeAmount
class NegativeAmount
{
};
// Definition of class insufficientFunds
class InsufficientFunds
{
};
// Definition of class Account
class Account
{
// Access specifier
private:
// Declaration of double variable
double balance;
// Access specifier
public:
// Definition of default constructor
Account()
{
// Assign 0 to balance
balance = 0;
}
// Definition of constructor to assign value
Account(double initialDeposit)
{
// Assign initialDeposit to balance
balance = initialDeposit;
}
// Definition of getBalance() function to return balance
double getBalance()
{
// Return balance
return balance;
}
// Definition of deposit() function to return new balance
// or throw NegativeAmount exception
double deposit(double amount) throw (NegativeAmount)
{
// Check amount is greater than 0
if (amount > 0)
// Condition is trur, compute balance
balance += amount;
// Otherwise
else
// Throw exception
throw NegativeAmount();
// Return balance
return balance;
}
// Definition of withdraw function to return new balance
// or throw NegativeAmount or InsufficientFunds
double withdraw(double amount) throw (NegativeAmount,
InsufficientFunds)
{
// Check amount is greater than balance
if (amount > balance)
// Throw insufficient exception
throw InsufficientFunds();
// Otherwise check amount is lesser than 0
else if (amount < 0)
// Throw negative amount execption
throw NegativeAmount();
// Otherwise
else
// Compute balance
balance -= amount;
// Return balance
return balance;
}
};
// Definition of main() function
int main()
{
// Creation of object and passing 100 to the constructor
Account a(100);
// Try block
try
{
// Call deposit() function to deposit 50
a.deposit(50);
// Call deposit() function to deposit 10
a.deposit(10);
// Call withdraw() function to deposit 220
a.withdraw(220);
// Call getBalance() function to get print balance amount
cout << a.getBalance() << endl;
}
// Catch block for negative amount
catch (NegativeAmount)
{
// Print the message regarding negative amount
cout << "You can't deposit or withdraw a negative amount."
<< endl;
}
// Catch block for insufficient funds
catch (InsufficientFunds)
{
// Print the message for insufficient funds
cout << "Insufficient funds for withdrawal." << endl;
}
// Declaration of character variable
char ch;
// Get an character
cin >> ch;
// Return 0
return 0;
}
Output:
Insufficient funds for withdrawal.
Want to see more full solutions like this?
Chapter 16 Solutions
Problem Solving with C++ plus MyProgrammingLab with Pearson eText-- Access Card Package (9th Edition)
- using r language a continuous random variable X has density function f(x)=1/4x^3e^-(pi/2)^4,x>=0 derive the probability inverse transformation F^(-1)x where F(x) is the cdf of the random variable Xarrow_forwardusing r language in an accelerated failure test, components are operated under extreme conditions so that a substantial number will fail in a rather short time. in such a test involving two types of microships 600 chips manufactured by an existing process were tested and 125 of them failed then 800 chips manufactured by a new process were tested and 130 of them failed what is the 90%confidence interval for the difference between the proportions of failure for chips manufactured by two processes? using r languagearrow_forwardI want a picture of the tools and the pictures used Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forward
- A. What will be printed executing the code above?B. What is the simplest way to set a variable of the class Full_Date to January 26 2020?C. Are there any empty constructors in this class Full_Date?a. If there is(are) in which code line(s)?b. If there is not, how would an empty constructor be? (create the code lines for it)D. Can the command std::cout << d1.m << std::endl; be included after line 28 withoutcausing an error?a. If it can, what will be printed?b. If it cannot, how could this command be fixed?arrow_forwardCisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardTransform the TM below that accepts words over the alphabet Σ= {a, b} with an even number of a's and b's in order that the output tape head is positioned over the first letter of the input, if the word is accepted, and all letters a should be replaced by the letter x. For example, for the input aabbaa the tape and head at the end should be: [x]xbbxx z/z,R b/b,R F ① a/a,R b/b,R a/a, R a/a,R b/b.R K a/a,R L b/b,Rarrow_forward
- Given the C++ code below, create a TM that performs the same operation, i.e., given an input over the alphabet Σ= {a, b} it prints the number of letters b in binary. 1 #include 2 #include 3 4- int main() { std::cout > str; for (char c : str) { if (c == 'b') count++; 5 std::string str; 6 int count = 0; 7 char buffer [1000]; 8 9 10 11- 12 13 14 } 15 16- 17 18 19 } 20 21 22} std::string binary while (count > 0) { binary = std::to_string(count % 2) + binary; count /= 2; std::cout << binary << std::endl; return 0;arrow_forwardConsidering the CFG described below, answer the following questions. Σ = {a, b} • NT = {S} Productions: P1 S⇒aSa P2 P3 SbSb S⇒ a P4 S⇒ b A. List one sequence of productions that can accept the word abaaaba; B. Give three 5-letter words that can be accepted by this CFG; C. Create a Pushdown automaton capable of accepting the language accepted by this CFG.arrow_forwardGiven the FSA below, answer the following questions. b 1 3 a a b b с 2 A. Write a RegEx that is equivalent to this FSA as it is; B. Write a RegEx that is equivalent to this FSA removing the states and edges corresponding to the letter c. Note: To both items feel free to use any method you want, including analyzing which words are accepted by the FSA, to generate your RegEx.arrow_forward
- 3) Finite State Automata Given the FSA below, answer the following questions. a b a b 0 1 2 b b 3 A. Give three 4-letter words that can be accepted by this FSA; B. Give three 4-letter words that cannot be accepted by this FSA; C. How could you describe the words accepted by this FSA? D. Is this FSA deterministic or non-deterministic?arrow_forwardConsidering the TM below, answer the following questions. a/x,R €/E,L €/E,R €/E,L x/E,R c/c,R b/E.L c/c,L x/x,R I J K L M F b/E.L D A. Give three 4-letter words that can be accepted by this TM; B. Give three 4-letter words that cannot be accepted by this TM; C. How could you describe the words accepted by this TM? D. What is the alphabet of the language accepted by this TM?arrow_forwardWhat is the generator? Explain motor generator motorarrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
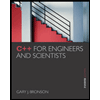
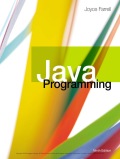
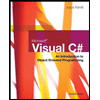
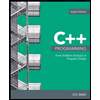
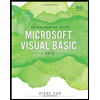