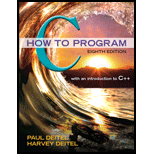
Concept explainers
(Account Class) Create an Account class that a bank might use to represent customers’ bank accounts. Include a data member of type int to represent the account balance. Provide a constructor that receives an initial balance arid uses it to initialize the data member. The constructor should validate the initial 0balance to ensure that it’s greater than or equal to 0. If not, set the balance to 0 and display an error message indicating that the initial balance was invalid. Provide three member functions. Member function credit should add an amount to the current balance. Member function debit should withdraw money from the Account and ensure that the debit amount does not exceed the Account’s balance. If it does, the balance should be left unchanged and the function should print a message indicating “Debit amount exceeded account balance.” Member function getBalance should return the current balance. Create a

Want to see the full answer?
Check out a sample textbook solution
Chapter 16 Solutions
C How to Program (8th Edition)
Additional Engineering Textbook Solutions
Electric Circuits. (11th Edition)
Degarmo's Materials And Processes In Manufacturing
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
SURVEY OF OPERATING SYSTEMS
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
- 1. Complete the routing table for R2 as per the table shown below when implementing RIP routing Protocol? (14 marks) 195.2.4.0 130.10.0.0 195.2.4.1 m1 130.10.0.2 mo R2 R3 130.10.0.1 195.2.5.1 195.2.5.0 195.2.5.2 195.2.6.1 195.2.6.0 m2 130.11.0.0 130.11.0.2 205.5.5.0 205.5.5.1 R4 130.11.0.1 205.5.6.1 205.5.6.0arrow_forwardAnalyze the charts and introduce each charts by describing each. Identify the patterns in the given data. And determine how are the data points are related. Refer to the raw data (table):arrow_forward3A) Generate a hash table for the following values: 11, 9, 6, 28, 19, 46, 34, 14. Assume the table size is 9 and the primary hash function is h(k) = k % 9. i) Hash table using quadratic probing ii) Hash table with a secondary hash function of h2(k) = 7- (k%7) 3B) Demonstrate with a suitable example, any three possible ways to remove the keys and yet maintaining the properties of a B-Tree. 3C) Differentiate between Greedy and Dynamic Programming.arrow_forward
- What are the charts (with their title name) that could be use to illustrate the data? Please give picture examples.arrow_forwardA design for a synchronous divide-by-six Gray counter isrequired which meets the following specification.The system has 2 inputs, PAUSE and SKIP:• While PAUSE and SKIP are not asserted (logic 0), thecounter continually loops through the Gray coded binarysequence {0002, 0012, 0112, 0102, 1102, 1112}.• If PAUSE is asserted (logic 1) when the counter is onnumber 0102, it stays here until it becomes unasserted (atwhich point it continues counting as before).• While SKIP is asserted (logic 1), the counter misses outodd numbers, i.e. it loops through the sequence {0002,0112, 1102}.The system has 4 outputs, BIT3, BIT2, BIT1, and WAITING:• BIT3, BIT2, and BIT1 are unconditional outputsrepresenting the current number, where BIT3 is the mostsignificant-bit and BIT1 is the least-significant-bit.• An active-high conditional output WAITING should beasserted (logic 1) whenever the counter is paused at 0102.(a) Draw an ASM chart for a synchronous system to providethe functionality described above.(b)…arrow_forwardS A B D FL I C J E G H T K L Figure 1: Search tree 1. Uninformed search algorithms (6 points) Based on the search tree in Figure 1, provide the trace to find a path from the start node S to a goal node T for the following three uninformed search algorithms. When a node has multiple successors, use the left-to-right convention. a. Depth first search (2 points) b. Breadth first search (2 points) c. Iterative deepening search (2 points)arrow_forward
- We want to get an idea of how many tickets we have and what our issues are. Print the ticket ID number, ticket description, ticket priority, ticket status, and, if the information is available, employee first name assigned to it for our records. Include all tickets regardless of whether they have been assigned to an employee or not. Sort it alphabetically by ticket status, and then numerically by ticket ID, with the lower ticket IDs on top.arrow_forwardFigure 1 shows an ASM chart representing the operation of a controller. Stateassignments for each state are indicated in square brackets for [Q1, Q0].Using the ASM design technique:(a) Produce a State Transition Table from the ASM Chart in Figure 1.(b) Extract minimised Boolean expressions from your state transition tablefor Q1, Q0, DISPATCH and REJECT. Show all your working.(c) Implement your design using AND/OR/NOT logic gates and risingedgetriggered D-type Flip Flops. Your answer should include a circuitschematic.arrow_forwardA controller is required for a home security alarm, providing the followingfunctionality. The alarm does nothing while it is disarmed (‘switched off’). It canbe armed (‘switched on’) by entering a PIN on the keypad. Whenever thealarm is armed, it can be disarmed by entering the PIN on the keypad.If motion is detected while the alarm is armed, the siren should sound AND asingle SMS message sent to the police to notify them. Further motion shouldnot result in more messages being sent. If the siren is sounding, it can only bedisarmed by entering the PIN on the keypad. Once the alarm is disarmed, asingle SMS should be sent to the police to notify them.Two (active-high) input signals are provided to the controller:MOTION: Asserted while motion is detected inside the home.PIN: Asserted for a single clock cycle whenever the PIN has beencorrectly entered on the keypad.The controller must provide two (active-high) outputs:SIREN: The siren sounds while this output is asserted.POLICE: One SMS…arrow_forward
- 4G+ Vo) % 1.1. LTE1 : Q B NIS شوز طبي ۱:۱۷ کا A X حاز هذا على إعجاب Mohamed Bashar. MEDICAL SHOE شوز طبي ممول . اقوى عرض بالعراق بلاش سعر القطعة ١٥ الف سعر القطعتين ٢٥ الف سعر 3 قطع ٣٥ الف القياسات : 40-41-42-43-44- افحص وكدر ثم ادفع خدمة التوصيل 5 الف لكافة محافظات العراق ופרסם BNI SH ופרסם DON JU WORLD DON JU MORISO DON JU إرسال رسالة III Messenger التواصل مع شوز طبي تعليق باسم اواب حمیدarrow_forwardA manipulator is identified by the following table of parameters and variables:a. Obtain the transformation matrices between adjacent coordinate frames and calculate the global transformation matrix.arrow_forwardWhich tool takes the 2 provided input datasets and produces the following output dataset? Input 1: Record First Last Output: 1 Enzo Cordova Record 2 Maggie Freelund Input 2: Record Frist Last MI ? First 1 Enzo Last MI Cordova [Null] 2 Maggie Freelund [Null] 3 Jason Wayans T. 4 Ruby Landry [Null] 1 Jason Wayans T. 5 Devonn Unger [Null] 2 Ruby Landry [Null] 6 Bradley Freelund [Null] 3 Devonn Unger [Null] 4 Bradley Freelund [Null] OA. Append Fields O B. Union OC. Join OD. Find Replace Clear selectionarrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
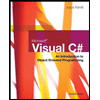
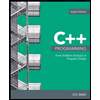
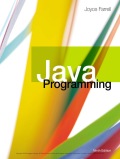
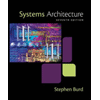
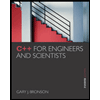