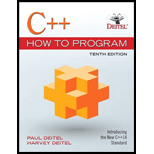
Fill in the blanks in each of the following statements:
a) The three styles of container classes are first-class containers, _______ and near containers.
b) Containers are divided into four major categories—sequence containers, ordered associative containers, _____ and container adapters.
c) The Standard Library container adapter most closely associated with the first-in, first- out (FIFO) insertion-and-removal discipline is the ______.
d) Built-in arrays, bitsets and valarrays are all _________ containers.
e) A(n) ______ constructor (C++11) moves the contents of an existing container of the same type into a new container, without the overhead of copying each element of the argument container.
f) The _______ container member function returns the number of elements currently in the container.
g) The _______ container member function returns true if the contents of the first container are not equal to the contents of the second; otherwise, returns false.
h) We use iterators with sequences—these be input sequences or output sequences, or they can be _________.
i) The Standard Library
j) Applications with frequent insertions and deletions in the middle and/or at the extremes of a container normally use a(n) ________.
k) Function __________ is available in every first-class container (except forward_list) and
it returns the number of elements currently stored in the container.
l) It can be wasteful to double a
m) As of C++11, you can ask a vector or deque to return unneeded memory to the system by calling member function ____.
n) The associative containers provide direct access to store and retrieve elements via keys (often called search keys). The ordered associative containers are multi set, set, ___ and ___.
o) Classes ____ and ____ provide operations for manipulating sets of values where the values are the keys—there is not a separate value associated with each key.
p) We use C++11’s auto keyword to ____.
q) A multimap is implemented to efficiently locate all values paired with a given ____.
r) The Standard Library container adapters are stack, queue and _____.

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
C++ How to Program (10th Edition)
- using r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardThe assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of…arrow_forwardI need help to solve the following case, thank youarrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
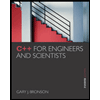
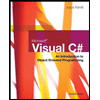
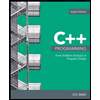
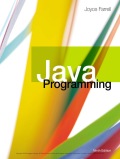
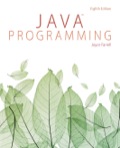