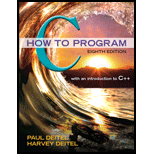
Determine whether the following
a. template < class A >
int sum(int num1, int num2, int num3)
{
return
;
}
b. void printResults (int x, int y)
{
cout « “The sum is”
return
;
}
c. template < A >
A product (A num1, A num2, A num3)
{
return num1 * num2 * num3;
}
double cube (int);
int cube (int);

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
C How to Program (8th Edition)
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (4th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting Out with C++: Early Objects
Java How To Program (Early Objects)
Absolute Java (6th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- *please help in java* Array testGrades contains NUM_VALS test scores. Write a for loop that sets sumExtra to the total extra credit received. Full credit is 100, so anything over 100 is extra credit. Ex: If testGrades = {101, 83, 107, 90}, then sumExtra = 8, because 1 + 0 + 7 + 0 is 8. import java.util.Scanner; public class SumOfExcess {public static void main (String [] args) {Scanner scnr = new Scanner(System.in);final int NUM_VALS = 4;int[] testGrades = new int[NUM_VALS];int i;int sumExtra = -9999; // Assign sumExtra with 0 before your for loop for (i = 0; i < testGrades.length; ++i) {testGrades[i] = scnr.nextInt();} // your answer goes here// System.out.println("sumExtra: " + sumExtra);}}arrow_forwardJAVA CODE ONLY AND PROVIDE OUTPUT SCREENSHOT PLEASEarrow_forwardConsider the following pseudo code, Method func() { PRINT “This is recursive function" func() } Method main( { func() } What will happen when the above snippet is executed?arrow_forward
- PLEASE CODE IN PYTHON Problem Description An anagram is a word or a phrase formed by rearranging the letters of another phrase such as “ITEM” and “TIME”. Anagrams may be several words long such as “CS AT WATERLOO” and “COOL AS WET ART”. Note that two phrases may be anagrams of each other even if each phrase has a different number of words (as in the previous example). Write a program to determine if two phrases are anagrams of each other. Input Specifications The input for the program will come from an input file in.dat, in.dat will have two lines of data, line (1) will have the first phrase and the line(2) will have the second phrase. You may assume that the input only contains upper case letters and spaces. Output Specifications The program will print out one of two statements: ”Is an anagram.” or, ”Is not an anagram.” All output will be to the screen. Sample Input CS AT WATERLOO COOL AS WET ART Output for Sample Input Is an anagram.arrow_forwardDownload the file Ackermann.cpp. Inside the file the recursive Ackermann function is implemented (described in Chapter 14 Programming Challenge 9). Do the following and answer the three questions: a) Run the program. What happens?b) Now uncomment the code that is commented out and run the program again. What happens now?c) What do you think is going on?arrow_forwardQuestion 1 Not complete Marked out of 1.00 Flag question Write a recursive function named count_non_digits (word) which takes a string as a parameter and returns the number of non-digits in the parameter string. The function should return 0 if the parameter string contains only digits. Note: you may not use loops of any kind. You must use recursion to solve this problem. You can assume that the parameter string is not empty. For example: Test Result print(count_non_digits ('123')) print(count_non_digits ('2rPTy5')) print(count_non_digits ('hello world')) 11 Precheck Check 0 4 Answer: (penalty regime: 0, 0, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 %) 1arrow_forward
- C programming language without using arraysarrow_forwardSample Output void showArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - accepts 3 int arrays and a size as arguments and displays their content. LO SHU MAGIC SQUARE Example: (arrayRow1) (arrayRow2) (arrayRow3) 135 Enter the three numbers for row 1: 1 3 2 679 824 Enter the three numbers for row' 2: 5 4 9 Enter the three numbers for row 3: 6 7 8 bool isMagicSquare(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - accepts 3 int arrays and a size as arguments and returns true if all the requirements of a magic square are met. Otherwise, it returns false. First argument corresponds to the first row of the magic square, second argument to the second row and the third argument to the third row of the magic square. 1 3 2 4 9 7 8 This is not a Lo Shu magic square. bool checkRange(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max) - accepts 3 int arrays, a size and a min and max value as arguments and returns true if the values in the…arrow_forwardWrite a recursive function that displays the number of even and odd digits in an integer using the following header: void evenAndOddCount(int value) Write a test program that prompts the user to enter an integer and displays the number of even and odd digits in it.arrow_forward
- Debugging challenge: Consider the code example below. This program is designed to compare the contents of two arrays: if they are equal, the program should display "TRUE," if the arrays are not equal, the program should display "FALSE". However, even when the arrays have the same value, the program always prints "FALSE", regardless of the values in arrays a and b. What is going on here? What about the program causes the comparison to always fail? int a[4] {1, 2, 3, 4}; int b[4] = {1, 2, 3, 4}; if (a == b) { display ("TRUE"); else { display ("FALSE");arrow_forwardQ1) Write a program to read the temperature of a particular location at a particular time of day for each day of a week. Find and display the highest and the lowest values among the temperatures recorded. Also, find the average temperature of the location and display it using the following methods: public static double findHighest(double[] array) public static double findLowest(double[] array) public static double findAverage(double[] array) Sample Input: Please enter the temperature for a week: 21 30 25.8 27.3 24 29 22.7 Sample Output: The Highest temperature is 30. The Lowest temperature is 21. The average temperature is 26.arrow_forwardPython Language Useful websites: : http://en.wikipedia.org/wiki/Radix http://www.purplemath.com/modules/numbbase.htm Special Rules: Use only Boolean/math expressions and conditional statements (if-statements). Do not use built-in functions for converting integers into a string representation. Here to start with: kthDigit(x: int, b: int, k: int) -> int .........arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
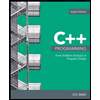
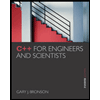
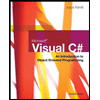