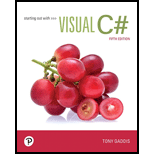
The variables used in the program are given below:
- connectionStringBuilder: an instance of the SqlConnectionStringBuilder class used to create a connection string to the productDB
database . - connectionString: a string that holds the connection string to the database.
- connection: an instance of the SqlConnection class used to connect to the database.
- query: a LINQ query that selects all the products in the database and sorts them by units on hand in ascending order.
- minUnitsOnHand: an integer variable that holds the minimum units on hand specified by the user.
- hasMin: a boolean variable that indicates whether a minimum number of units on hand was specified by the user.
- maxUnitsOnHand: an integer variable that holds the maximum units on hand specified by the user.
- hasMax: a boolean variable that indicates whether a maximum number of units on hand was specified by the user.
The methods used in the program are as follows:
- The SqlConnectionStringBuilder constructor is used to create a connection string to the database.
- The SqlConnection constructor, which creates a new SqlConnection object that can be used to connect to the database.
- The SqlCommand constructor creates a new SqlCommand object that can be used to execute a query against the database.
- The ExecuteReader method of the SqlCommand class is used to execute the query and return a SqlDataReader object that can be used to read the results.
- The Where method of the IQueryable interface is used to filter the data based on a specified condition.
- The TryParse method of the int class is used to convert user input from a string to an integer.
- The Console.WriteLine method, which is used to display information to the user in the console window.
- The Console.ReadLine method, which is used to read user input from the console window.
Program Description:
To write an application that connects to productDB database.
Steps to create an application that connects to a database, sorts, and searches data using LINQ and SQL:
- Create a new C# console application project in Visual Studio.
- Add a reference to the System.Data.SqlClient and System.Linq namespaces.
- Create a connection string to the productDB database using SqlConnectionStringBuilder.
- Create a SqlConnection object and open the connection to the database.
- Create a LINQ query to select all the products in the database and sort them by units on hand in ascending order. You can use the OrderBy method in LINQ to sort the data.
- Execute the query using the SqlCommand object and read the data using the SqlDataReader object.
- Display the data to the user.
- Prompt the user to enter a minimum and/or maximum number of units on hand.
- Modify the LINQ query to filter the products based on the user's input. You can use the Where method in LINQ to filter the data.
- Execute the modified query and display the filtered data to the user.
- Close the SqlDataReader and SqlConnection objects.
Program:
using System; using System.Data.SqlClient; using System.Linq; namespace ProductUnitsOnHandSearch { class Program { static void Main(string[] args) { // Create connection string to the productDB database var connectionStringBuilder = new SqlConnectionStringBuilder { DataSource = "your_server_name", // Replace with your actual database name InitialCatalog = "productDB", IntegratedSecurity = true }; string connectionString = connectionStringBuilder.ToString(); // Create SqlConnection object and open connection using (SqlConnection connection = new SqlConnection(connectionString)) { connection.Open(); // Create LINQ query to select all products and sort by units on hand in ascending order var query = from p in db.Products orderby p.UnitsOnHand ascending select p; // Execute query and read data using (SqlCommand command = new SqlCommand(query.ToString(), connection)) { using (SqlDataReader reader = command.ExecuteReader()) { // Display all products Console.WriteLine("All Products:"); Console.WriteLine("--------------"); while (reader.Read()) { Console.WriteLine("{0}: {1} units on hand", reader["ProductName"], reader["UnitsOnHand"]); } Console.WriteLine(); } } // Prompt user for minimum and maximum units on hand Console.Write("Enter minimum units on hand (or leave blank for no minimum): "); string minInput = Console.ReadLine(); int minUnitsOnHand; bool hasMin = int.TryParse(minInput, out minUnitsOnHand); Console.Write("Enter maximum units on hand (or leave blank for no maximum): "); string maxInput = Console.ReadLine(); int maxUnitsOnHand; bool hasMax = int.TryParse(maxInput, out maxUnitsOnHand); // Modify query to filter by units on hand based on user input if (hasMin) { query = query.Where(p => p.UnitsOnHand >= minUnitsOnHand); } if (hasMax) { query = query.Where(p => p.UnitsOnHand <= maxUnitsOnHand); } // Execute modified query and display filtered products using (SqlCommand command = new SqlCommand(query.ToString(), connection)) { using (SqlDataReader reader = command.ExecuteReader()) { Console.WriteLine("Filtered Products:"); Console.WriteLine("------------------"); while (reader.Read()) { Console.WriteLine("{0}: {1} units on hand", reader["ProductName"], reader["UnitsOnHand"]); } } } } Console.ReadLine(); } } }
Explanation:
The program is a console application that connects to a database called productDB and displays information about the products in the database. The program uses LINQ and SQL to interact with the database and allows the user to search for products with more units on hand than a specified amount or fewer units on hand than a specified amount.
When the program starts, it creates a connection string to the productDB database and opens a connection to the database using the SqlConnection and SqlCommand classes. The program then executes a LINQ query to select all the products in the database and sorts them by units on hand in ascending order.
The program then prompts the user to enter a minimum unit on hand and a maximum unit on hand. If the user enters a valid integer for the minimum or maximum units on hand, the program filters the results using the Where method of the IQueryable interface. If the user does not enter a value for the minimum or maximum units on hand, the program skips that filter.
Finally, the program loops through the results and displays the product ID, name, and units on hand for each product that matches the user's search criteria. If no products match the criteria, the program displays a message indicating that no results were found. The user can then exit the program or search for products again.
SAMPLE OUTPUT:
Enter minimum units on hand (press enter to skip): 50 Enter maximum units on hand (press enter to skip): 100 Products with units on hand between 50 and 100: ---------------------------------------------- Product ID: 2 Name: Rechargeable Lamp Units on hand: 75 Product ID: 4 Name: Smart watch Units on hand: 80 Do you want to search again? (Y/N) y Enter minimum units on hand (press enter to skip): Enter maximum units on hand (press enter to skip): 200 Products with units on hand between 0 and 200: ---------------------------------------------- Product ID: 1 Name: Wireless Earbuds Units on hand: 120 Product ID: 3 Name:Head phones Units on hand: 150 Do you want to search again? (Y/N) n Press any key to exit...

Want to see the full answer?
Check out a sample textbook solution
Chapter 14 Solutions
Pearson eText for Starting out with Visual C# -- Instant Access (Pearson+)
- Please answer Java OOP Questions.arrow_forward.NET Interactive Solving Sudoku using Grover's Algorithm We will now solve a simple problem using Grover's algorithm, for which we do not necessarily know the solution beforehand. Our problem is a 2x2 binary sudoku, which in our case has two simple rules: •No column may contain the same value twice •No row may contain the same value twice If we assign each square in our sudoku to a variable like so: 1 V V₁ V3 V2 we want our circuit to output a solution to this sudoku. Note that, while this approach of using Grover's algorithm to solve this problem is not practical (you can probably find the solution in your head!), the purpose of this example is to demonstrate the conversion of classical decision problems into oracles for Grover's algorithm. Turning the Problem into a Circuit We want to create an oracle that will help us solve this problem, and we will start by creating a circuit that identifies a correct solution, we simply need to create a classical function on a quantum circuit that…arrow_forwardNeed help with this in python!arrow_forward
- If you created a main document based on an existing document entitled "Confirmation Letter," what default filename would Word give the main document? Question 14Select one: a. Confirmation Letter-1 b. Confirmation Letter-merge c. Document1 d. MergedDocument1arrow_forwardClick the ____ option button in the Mail Merge task pane to use an Outlook contact list as a data source for a merge. Question 11Select one: a. Use Outlook contacts list b. Select from Outlook contacts c. Select Contacts d. Mail Merge Recipientsarrow_forwardA(n) ____ cannot be selected as the document type in the Mail Merge task pane. Question 9Select one: a. Letter b. Directory c. Fax d. E-mail messagearrow_forward
- Consider a Superstore Database which consists of 3 tables, Orders, Returns, and Managers. The CSV files have been provided along with this DOC file in the Midterm 2 Link in the Moodle. Answer the questions as below: Use the created table as in the provided SQL query file, solve the problems as mentioned below. You will have to import the respective CSV files of the above created tables as without them, it is impossible to solve the questions below. If you are not able to upload the files successfully, do not leave the query questions. Just write the query to the best of your knowledge. Do not copy. To be graded for the screenshot answer, you must upload the CSV properly and paste the resulting screenshot of the queries as asked. Write Query to Find out which Product Sub-Category has a sum of Shipping Cost to sum of Sales ratio > 0.03.arrow_forwardI need to render an image of a car continuously for a smooth visual experience in C# WinForms. It gets the location array (that has all the x,y of the tiles it should visit) from another function - assume it is already written.arrow_forwardwrite c program with features: Register a Bunny: Store the bunny's name, poem, and initialize the egg count to 0. Modify an Entry: Change the bunny's poem or update the egg count. Delete a Bunny: Remove a registered bunny from the list. List All Bunnies: Display all registered bunnies and their details. Save & Load Data: Store bunny data in a file to persist between runs. Use a struct to represent a bunny contestant. Store data in a binary file (bunnies.dat) for persistence. Use file I/O functions (fopen, fwrite, fread, etc.) to manage data. Implement a menu-driven interface for user interaction.arrow_forward
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
- Enhanced Discovering Computers 2017 (Shelly Cashm...Computer ScienceISBN:9781305657458Author:Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. CampbellPublisher:Cengage LearningFundamentals of Information SystemsComputer ScienceISBN:9781305082168Author:Ralph Stair, George ReynoldsPublisher:Cengage LearningNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
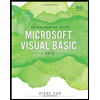
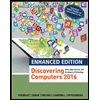
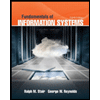