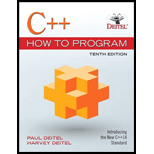
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 14, Problem 14.10E
Write a series of statements that accomplish each of the following. Assume that we've defined class Person that contains the private data members
char lastName[15];
char firstName [10]:
int age;
int id;
and public member functions
// accessor functions for id
void set Id(int);
int getId() const;
// accessor functions for lastName
void setLastName (const string&);
string getLastName() const;
// accessor functions for firstName
void setFirstName(const string&);
string getFirstName() const;
// accessor functions for age
void setAge (int);
int getAge() const;
Also assume that any random-access files have been opened properly.
- Initialize nameage.dat with 100 records that store values lastName =”unassigned”, firstName = “” and age = 0.
- Input 10 last names, first names and ages, and write them to the file,
- Update a record that already contains information. If the record does not contain information, inform the user "No info".
- Delete a record that contains information by reinitializing that particular record.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
C++
Personal Information Class:
Design a class that holds the following personal data: name, age, and phone number. Write appropriate accessor and mutator functions. Demonstrate the class by writing a program that creates three instances of it. One instance should hold your information, and the other two should hold your friend's or family member's information.
Programming Exercise 11-2
dateType.h file provided
#ifndef date_H
#define date_H
class dateType
{
public:
void setDate(int month, int day, int year);
//Function to set the date.
//The member variables dMonth, dDay, and dYear are set
//according to the parameters
//Postcondition: dMonth = month; dDay = day;
// dYear = year
int getDay() const;
//Function to return the day.
//Postcondition: The value of dDay is returned.
int getMonth() const;
//Function to return the month.
//Postcondition: The value of dMonth is returned.
int getYear() const;
//Function to return the year.
//Postcondition: The value of dYear is returned.
void printDate() const;
//Function to output the date in the form mm-dd-yyyy.
bool isLeapYear();
//Function to determine whether the year is a leap year.
dateType(int month = 1, int day = 1, int year = 1900);
//Constructor to…
Chapter 9 defined the struct studentTypeto implement the basic properties of a student. Define the class studentType with the same components as the struct studentType, and add member functions to manipulate the data members. (Note that the data members of the class studentType must be private.)
Write a program to illustrate how to use the class studentType.
Struct studentType:
struct studentType { string firstName; string lastName; char courseGrade; int testScore; int programmingScore; double GPA; };
An example of the program is shown below:
Name: Sara Spilner Grade: A Test score: 89 Programming score: 92 GPA: 3.57 ***************
Chapter 14 Solutions
C++ How to Program (10th Edition)
Ch. 14 - (Fill in the Blanks) Fill in the blanks in each of...Ch. 14 - (File Matching) Exercise 14.3 asked you to write a...Ch. 14 - (File Matching Test Data) After writing the...Ch. 14 - Prob. 14.8ECh. 14 - (File-Matching Enhancement) Its common to have...Ch. 14 - Write a series of statements that accomplish each...Ch. 14 - Prob. 14.11ECh. 14 - (Telephone-Number Word Generator) Standard...Ch. 14 - (sizeof Operator) Write a program that uses the...Ch. 14 - Prob. 14.14MAD
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Language=C++ Create an employee class. The member data should comprise an int for storing the employee number and a float for storing the employee’s compensation. Member functions should allow the user to enter this data and display it. Write a main() that allows the user to enter data for three employees and display it (Use Array of objects).arrow_forwardprogramming language :c++ make a program in c++ to store hiring date and date of birth for Managers and Employees. You need to create a Date class for this purpose. Create objects of Date class in Manager and Employee classes to store respective dates. You need to write print function in Manager and Employee classes as well to print all information of Managers and Employees. You need to perform composition to implement this task. Create objects of Manager and Employee classes in main function and call print function for both objects. Print Date class here. Print updated Manager class here. Print updated Employee class here. Print main function here.arrow_forwardin c++arrow_forward
- In C++ Q1.) Write a Review class that has: • These private data members: • string user: ID of the user • string item: ID of the item • double paid: the price of the item that the user buys • double rating: the rating that the user gives to the item • string review: the review content that the user gives to the item • These public member functions: • A default constructor to set all instance variables to a default value • A parameterized constructor (i.e., a constructor with arguments) to set all instance variables • A method for getting/setting the instance variables. • A method for printing the Review's information.arrow_forwardCreate a class: Doctor, with private data members: id, name, specialization and salary with public member function: get_data () to take input for all data members, show_data () to display the values of all data members and get_specialization () to return specialization. Write a program to write 10 records of doctors and allocating the memory at run time write the code in C++arrow_forwardObject oriented programming C++ Use Classes Write a C++ program in which each flight is required to have a date of departure, a time of departure, a date of arrival and a time of arrival. Furthermore, each flight has a unique flight ID and the information whether the flight is direct or not.Create a C++ class to model a flight. (Note: Make separate classes for Date and Time and use composition).add two integer data members to the Flight class, one to store the total number of seats in the flight and the other to store the number of seats that have been reserved. Provide all standard functions in each of the Date, Time and Flight classes (Constructors, set/get methods and display methods etc.).Add a member function delayFlight(int) in the Flight class to delay the flight by the number of minutes pass as input to this function. (For simplicity, assume that delaying a flight will not change the Date). Add a member function reserveSeat(int) which reserves the number of seats passed as…arrow_forward
- Create a class: Doctor, with private data members: id, name, specialization and salary with public member function: get_data () to take input for all data members, show_data () to display the values of all data members and get_specialization () to return specialization. Write a program to write 10 records of doctors and allocating the memory at run time only. Programming language: C++arrow_forwardC++arrow_forwardNote:C++ programming Create a class: “Palindrome” with private data members: upper_limit (int), lower_limit (int) and parameterized constructor, which initializes the values of both data members. Apart from initialization, this constructor also displays the count of palindrome numbers from upper_limit to lower_limit. [Example: upper_limit:50, lower_limit:10, count of palindrome numbers is:4(11 , 22, 33 and 44), also make sure the value of upper_limit should be greater than lower_limit].arrow_forward
- The code is in C++arrow_forwardC++ You may work on a single class. The parameter and return type s of each function and class member function should be decided in advance. The program will be best implemented as a multi-file program, (header file, the main program,..) Design a generic class to hold the following information about a bank account: Balance Number of deposits this month Number of withdrawals Annual Interest Rate Monthly service charges The class should have the following member function: Constructors Accepts arguments for the balance and annual interest rate. deposit a virtual function that accepts an argument for the amount of the deposit. The function should add the argument to the account balance. It should also increment the variable holding the number of deposits. withdraw a virtual function that accepts an argument for the amount of the withdrawal. The function should subtract the argument from the account balance. It should also increment the variable…arrow_forwardB. Pet Class Using Python Program, Write a class named Pet, which should have the following data attributes: • _ _name (for the name of a pet) •__animal_type (for the type of animal that a pet is. Example values are 'Dog', 'Cat', and `Bird')' __age (for the pet's age) init The Pet class should have an method that creates these attributes. It should also have the following methods: set_name() This method assigns a value to the __name field. set_animal_type() This method assigns a value to the __animal_type field. • set_age() This method assigns a value to the • get_name() This method returns the value of the get_animal_type() This method returns the value of the __animal_type field. • get_age() This method returns the value of the _age field. Once you have written the class, write a program that creates an object of the class and prompts the user to enter the name, type, and age of his or her pet. This data should be stored as the object's attributes. Use the object's accessor methods…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
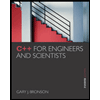
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
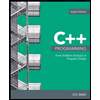
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
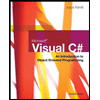
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
What is Abstract Data Types(ADT) in Data Structures ? | with Example; Author: Simple Snippets;https://www.youtube.com/watch?v=n0e27Cpc88E;License: Standard YouTube License, CC-BY