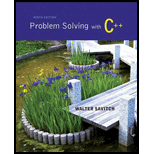
The following
#include <iostream>
#include <string>
using namespace std;
struct Node
{
string name;
Node *link;
};
typedef Node* NodePtr;
int main()
{
NodePtr listPtr, tempPtr;
listPtr = new Node;
listPtr–>name = “Emily”;
tempPtr = new Node;
tempPtr–>name = “James”;
listPtr–>link = tempPtr;
tempPtr–>link = new Node;
tempPtr = tempPtr–>link;
tempPtr–>name = “Joules”;
tempPtr–>link = NULL;
return 0;
}
Add code to the main function that:
- a. Outputs in order all names in the list.
- b. Inserts the name “Joshua” in the list after “James” then outputs the modified list.
- c. Deletes the node with “Joules” then outputs the modified list,
- d. Deletes all nodes in the list.

Creation of program to produce a linked list and perform operations
Program Plan:
- Define a structure “Node” with member variable and link to another node.
- Define a method “displayList()” to display nodes present in the list.
- Perform a loop operation until list reaches null.
- Display name of node and move to next node.
- Continue this process until list reaches null.
- Define a method “insertNameAfter()” to insert a name after a particular name.
- Perform a loop operation until list reaches null.
- Compare each word with given name.
- If required name is reached, insert new name after the given name.
- Update the next node pointer.
- Define a method “deleteNameList()” to delete a particular name in list.
- Perform a loop operation until list reaches null.
- Compare each word with given name.
- If required name is reached, delete name.
- Update the next node pointer.
- Define a method “deleteAllNodes()” to delete all nodes in list.
- Declare variables that are required for program.
- Perform a loop operation until list reaches null.
- Assign “head” to a temporary variable.
- Move to next node.
- Delete the “head” of linked list.
- Define a main method to perform operations on list.
- Declare variables that are required for program.
- Define nodes and assign values.
- Call method “displayList()” to display nodes in list.
- Call method “insertNameAfter()” to insert a name after a particular name.
- Call method “deleteNameList()” to delete a particular name in list.
- Call method “deleteAllNodes()” to delete all nodes in list.
Program Description:
The following C++ program describes about creation of program to create a linked list and perform operations on list.
Explanation of Solution
Program:
//Include libraries
#include <iostream>
#include <string>
//Use namespace
using namespace std;
//Define a structure
struct Node
{
//Declare member variable
string name;
//Declare link
Node *link;
};
//Define instance
typedef Node* NodePtr;
//Define a method displayList()
void displayList(NodePtr head)
{
//Loop until empty
while(head!=NULL)
{
//Display value
cout<<head->name<<" ";
//Move to next node
head=head->link;
}
//New line
cout<<endl;
}
//Define method insertNameAfter()
void insertNameAfter(NodePtr head,string sName, string newName)
{
//Declare variable
NodePtr temp;
//Create instance of node
temp=new Node;
//Assign value
temp->name=newName;
//Assign null value
temp->link=NULL;
//Loop until it reaches null
while(head!=NULL)
{
//If condition satisfies
if(head->name.compare(sName)==0)
{
//Assign value
temp->link=head->link;
//Assign value
head->link=temp;
//Break
break;
}
//Assign value
head=head->link;
}
}
//Define method deleteNameList()
void deleteNameList(NodePtr head,string sName)
{
//Declare variable
NodePtr prev=NULL;
//Loop until it reaches null
while(head!=NULL)
{
//If condition satisfies
if(head->name.compare(sName)==0)
{
//Assign value
prev->link=head->link;
//Delete
delete head;
//Break
break;
}
//Assign value
prev=head;
//Assign value
head=head->link;
}
}
//Define method deleteAllNodes()
void deleteAllNodes(NodePtr head)
{
//Declare variable
NodePtr temp;
//Loop
while(head)
{
//Assign value
temp=head;
//Move to next value
head=head->link;
//Delete node
delete temp;
}
}
//Define main method
int main()
{
//Declare variables
NodePtr listPtr,tempPtr;
//Create new instance
listPtr =new Node;
//Assign value
listPtr->name="Emily";
//Create new node
tempPtr=new Node;
//Assign value
tempPtr->name="James";
//Assign value
listPtr->link=tempPtr;
//Create new node
tempPtr->link=new Node;
//Move to next value
tempPtr=tempPtr->link;
//Assign value
tempPtr->name="Joules";
//Assign null value
tempPtr->link=NULL;
//Display message
cout<<"All Names in the list are: "<<endl;
//Call method displayList()
displayList(listPtr);
//Call method insertNameAfter()
insertNameAfter(listPtr,"James","Joshua");
//Display message
cout<<"Output modified list after Insert Joshua are:"<<endl;
//Call method displayList()
displayList(listPtr);
//Call method deleteNameList()
deleteNameList(listPtr,"Joules");
//Display message
cout<<"Output modified list after deleting Joshua are:"<<endl;
//Call method displayList()
displayList(listPtr);
//Call method deleteAllNodes()
deleteAllNodes(listPtr);
//Pause console window
system("pause");
//Return
return 0;
}
All Names in the list are:
Emily James Joules
Output modified list after Insert Joshua are:
Emily James Joshua Joules
Output modified list after deleting Joshua are:
Emily James Joshua
Press any key to continue . . .
Want to see more full solutions like this?
Chapter 13 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Web Development and Design Foundations with HTML5 (8th Edition)
Mechanics of Materials (10th Edition)
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Introduction To Programming Using Visual Basic (11th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Modern Database Management
- Exercise docID document text docID document text 1 hot chocolate cocoa beans 7 sweet sugar 2345 9 cocoa ghana africa 8 sugar cane brazil beans harvest ghana 9 sweet sugar beet cocoa butter butter truffles sweet chocolate 10 sweet cake icing 11 cake black forest Clustering by k-means, with preprocessing tokenization, term weighting TFIDF. Manhattan Distance. Number of cluster is 2. Centroid docID 2 and docID 9.arrow_forwardChange the following code so that there is always at least one way to get from the left corner to the top right, but the labyrinth is still randomized. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. Take care that the player and the dragon cannot start off on walls. Also the dragon starts off from a randomly chosen position public class Labyrinth { private final int size; private final Cell[][] grid; public Labyrinth(int size) { this.size = size; this.grid = new Cell[size][size]; generateLabyrinth(); } private void generateLabyrinth() { Random rand = new Random(); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { // Randomly create walls and paths grid[i][j] = new Cell(rand.nextBoolean()); } } // Ensure start and end are…arrow_forwardChange the following code so that it checks the following 3 conditions: 1. there is no space between each cells (imgs) 2. even if it is resized, the components wouldn't disappear 3. The GameGUI JPanel takes all the JFrame space, so that there shouldn't be extra space appearing in the frame other than the game. Main(): Labyrinth labyrinth = new Labyrinth(10); Player player = new Player(9, 0); Dragon dragon = new Dragon(9, 9); JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon); frame.add(gui); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; //labyrinth, player, dragon are just public classes private final ImageIcon playerIcon = new ImageIcon("data/images/player.png");…arrow_forward
- Make the following game user friendly with GUI, with some simple graphics. The GUI should be in another seperate class, with some ImageIcon, and Game class should be added into the pane. The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return…arrow_forwardDiscuss the negative and positive impacts or information technology in the context of your society. Provide two references along with with your answerarrow_forwardA cylinder of diameter 10 cm rotates concentrically inside another hollow cylinder of inner diameter 10.1 cm. Both cylinders are 20 cm long and stand with their axis vertical. The annular space is filled with oil. If a torque of 100 kg cm is required to rotate the inner cylinder at 100 rpm, determine the viscosity of oil. Ans. μ= 29.82poisearrow_forward
- Make the following game user friendly with GUI, with some simple graphics The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return isWall; } public void setWall(boolean isWall) { this.isWall = isWall; } @Override public String toString() {…arrow_forwardPlease original work What are four of the goals of information lifecycle management think they are most important to data warehousing, Why do you feel this way, how dashboards can be used in the process, and provide a real life example for each. Please cite in text references and add weblinksarrow_forwardThe following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forward
- In this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forwardHow do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forwardYou are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
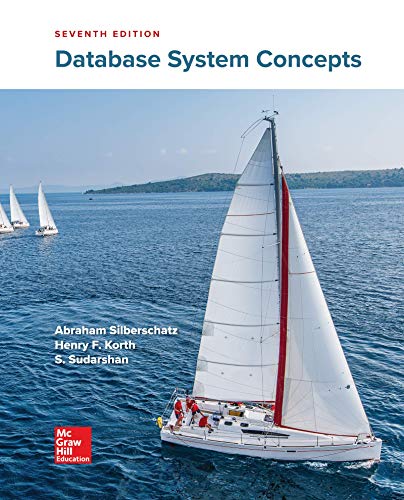
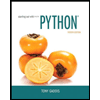
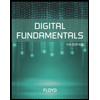
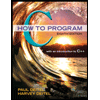
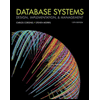
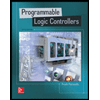