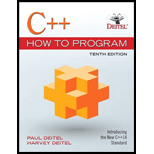
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 13, Problem 13.8E
Program Plan Intro
- In the program, we include the header files as needed.
- Declaring main() function as integer type.
- Variable declaration: test_value1 as integer with the value 15 and test_ptr as integer pointer.
- Type casting is used to print the value of pointer.
Program Description:
The program is to print the pointer value as integer.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
/2
14.
sin² 20 cos³ 20 de
10.
8 sin¹ y cos² y dy
Ln (z+1)
dz, C: |zi| = 1.4
-
17.
z 2 + 1
C
Chapter 13 Solutions
C++ How to Program (10th Edition)
Ch. 13 - (Write C ++ statements) Write a statement for each...Ch. 13 - (Inputting Decimal, Octal and Hexadecimal Values)...Ch. 13 - Prob. 13.8ECh. 13 - (Printing with field Widths) Write a program to...Ch. 13 - (Rounding) Write a program that prints the value...Ch. 13 - (Length of a String) Write a program that inputs a...Ch. 13 - (Converting Fahrenheit to Celsius) Write a program...Ch. 13 - In some programming language, string are entered...Ch. 13 - Prob. 13.14ECh. 13 - Prob. 13.15E
Knowledge Booster
Similar questions
- 15 OF 25 QUESTIONS REMAININ Consider the following code. You want to print the array values in the div as an ordered list. What statement would you use to replace the comment in the code below? Two J // what statement goes here? - لبية للالكالا const app = Vue.createApp({ data (( return ( lunch: [ 'Burrito', 'Soup', 'Pizza', 'Rice' }) app.mount ('#app6') - -arrow_forwardPlease answer JAVA OOP problem below: Assume you have three data definition classes, Person, Student and Faculty. The Student and Faculty classes extend Person. Given the code snippet below, in Java, complete the method determinePersonTypeCount to print out how many Student and Faculty objects exist within the Person array. You may assume that each object within the Person[] is either referencing a Student or Faculty object. public static void determinePersonTypeCount(Person[] people){ // Place your code here }arrow_forwardPlease answer JAVA OOP question below: Consider the following relationship diagram between the Game and VideoGame data defintion classes. Game has a constructor that takes in two parameters, title (String) and cost (double). The VideoGame constructor has an additional parameter, genre (String). In Java, efficiently write the constructors needed within the Game class and VideoGame classes. Hint: Remember to think about the appropriate validationarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
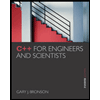
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
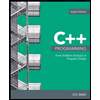
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
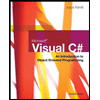
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
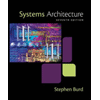
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
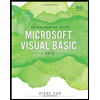
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
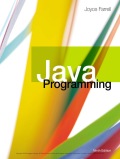
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT