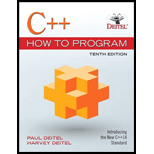
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 13, Problem 13.14E
Program Plan Intro
Program Plan:
- Insert required header Files
- Create new file PhoneNumber.h.
- Make a class PhoneNumber and declare extraction and insertion operators as friend to class.
- Declare three string variable “ areacode ”, “ exchange ”, “ line ” and one character array “ phno ” to get phone number in an array.
- End header class.
- Close and save header file.
- Create new file Phonenumber.cpp. This will contain definitions and modification required in extraction operator ">>".
- Include header files, must include PhoneNumber.h
- Overload the insertion operator to display areacode, exchange and line.
- Make changes the extraction operator definition.
- use "getline()" function to get input into "phno". The "getline()" function is used to identify psaces also.
- Use if construct to check various conditions
- Check for length of input entered which should be 14, use input.clear(ios::failbit) to set failbit.
- Check that areacode does not start with either 0 or 1, if it does then use input.clear(ios::failbit) to set failbit.
- Check that exchange does not start with either 0 or 1, if it does then use input.clear(ios::failbit) to set failbit.
- Check that middle digit of areacode should be either 0 or 1, if not then use input.clear(ios::failbit) to set failbit.
- If all the conditions are false, this means that input is correct, thus put them into areacode, exchange and line of number object passed through the function.
- return input
- end function
- Make new C++ file for main() function.
- Include header files, must include PhoneNumber.h.
- Start main function.
- Declare an object of PhoneNumber class phone.
- Display message to enter phone number.
- Use cin>>phone which will call overloaded extraction operator.
- Check whether state returned is goodbit(incase failbit is not set in the function) then display phone number else display error.
- Return and exit.
Program Description:
Program to get correct input by overloading extraction operator.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
I want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.To elaborate, what…
Male comedians were typically the main/dominant star of television sitcoms made during the FCC licensing freeze.
Question 19 options:
True
False
In the episode of The Honeymooners that you watched this week, why did Alice decide to get a job outside of the home?
Question 1 options:
to earn enough money to buy a mink coat
to have something to do while the kids were at school
to pay the bills after her husband got laid off
After the FCC licensing freeze was lifted, sitcoms featuring urban settings and working class characters became far less common.
Question 14 options:
True
False
Chapter 13 Solutions
C++ How to Program (10th Edition)
Ch. 13 - (Write C ++ statements) Write a statement for each...Ch. 13 - (Inputting Decimal, Octal and Hexadecimal Values)...Ch. 13 - Prob. 13.8ECh. 13 - (Printing with field Widths) Write a program to...Ch. 13 - (Rounding) Write a program that prints the value...Ch. 13 - (Length of a String) Write a program that inputs a...Ch. 13 - (Converting Fahrenheit to Celsius) Write a program...Ch. 13 - In some programming language, string are entered...Ch. 13 - Prob. 13.14ECh. 13 - Prob. 13.15E
Knowledge Booster
Similar questions
- solve this questions for me .arrow_forwarda) first player is the minimizing player. What move should be chosen?b) What nodes would not need to be examined using the alpha-beta pruning procedure?arrow_forwardConsider the problem of finding a path in the grid shown below from the position S to theposition G. The agent can move on the grid horizontally and vertically, one square at atime (each step has a cost of one). No step may be made into a forbidden crossed area. Inthe case of ties, break it using up, left, right, and down.(a) Draw the search tree in a greedy search. Manhattan distance should be used as theheuristic function. That is, h(n) for any node n is the Manhattan distance from nto G. The Manhattan distance between two points is the distance in the x-directionplus the distance in the y-direction. It corresponds to the distance traveled along citystreets arranged in a grid. For example, the Manhattan distance between G and S is4. What is the path that is found by the greedy search?(b) Draw the search tree in an A∗search. Manhattan distance should be used as thearrow_forward
- whats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forwardWhat is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.arrow_forward
- Consider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forwardassume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forward
- Consider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forwardNo aiarrow_forwardGiven the dependency diagram of attributes {C1,C2,C3,C4,C5) in a table shown in the following figure, (the primary key attributes are underlined)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
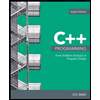
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
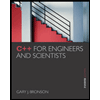
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
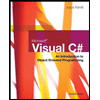
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
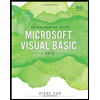
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning