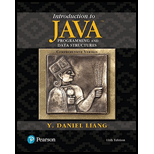
(Triangle class) Design a new Triangle class that extends the abstract GeometricObject class. Draw the UML diagram for the classes Triangle and GeometricObject then implement the Triangle class. Write a test

Program to create Triangle class
Program Plan:
- Include a class name named “Exercise_13_01”.
- Include the scanner package.
- Declare the main() function.
- Create a scanner object.
- Prompt the user to enter three sides of the triangle.
- Prompt the user to enter a color.
- Prompt the user to enter a Boolean value on whether the triangle is filled.
- Create triangle object with the entered user input.
- Set color and set filled in the triangle.
- Print the output.
- Close the main() function.
- Close the class “Exercise_13_01”.
- Include an abstract class “GeometricObject”.
- Declare all the data types of the variables.
- Construct a default geometric object.
- Construct a geometric object with color and filled value.
- Assign color and fill to the object.
- Set a new color.
- Define an isFilled method returns filled and since filled is Boolean; the get method is named isFilled.
- Set a new filled and getDateCreated method.
- Override toString function to return output.
- Define Abstract method getArea.
- Define Abstract method getPerimeter.
- Close class.
- Include a class “Triangle” that extends the class GeometricObject.
- Declare the three sides of the triangle.
- Include a triangle constructor.
- Assign values to all three sides of the triangle.
- Assign triangle values to all attributes.
- Return side1 and Set side1 to a new length.
- Return side2 and Set side2 to a new length.
- Return side3 and Set side3 to a new length.
- Create a Function to return area of the Triangle.
- Create a function to return a string description of the object.
- Close class.
The java code to design a new Triangle class that extends the abstract GeometricObject class and to design a test program that prompts the user to enter three sides of the triangle, a color, and a Boolean value to indicate whether the triangle is filled or not.
Explanation of Solution
Program:
File name: Exercise_13_01.java
//package to have user input
import java.util.Scanner;
//class Definition
public class Exercise_13_01
{
// Main method
public static void main(String[] args)
{
// Create a Scanner object
Scanner input = new Scanner(System.in);
/* Prompt the user to enter three sides of the triangle */
System.out.print("Enter three side of the triangle: ");
double side1 = input.nextDouble();
double side2 = input.nextDouble();
double side3 = input.nextDouble();
// Prompt the user to enter a color
System.out.print("Enter a color: ");
String color = input.next();
/*Prompt the user to enter a boolean value on whether the triangle is filled*/
System.out.print("Is the triangle filled (true / false)? ");
boolean filled = input.nextBoolean();
/* Create triangle object with the entered user input */
Triangle triangle = new Triangle(side1, side2, side3);
// set color
triangle.setColor(color);
// set filled
triangle.setFilled(filled);
// print the output
System.out.println(triangle.toString());
System.out.println("Area: " + triangle.getArea());
System.out.println("Perimeter: " + triangle.getPerimeter());
System.out.println("Color: " + triangle.getColor());
System.out.println("Triangle is" + (triangle.isFilled() ? "" : " not ")
+ "filled");
}
}
Filename: GeometricObject.java
//abstract class GeometricObject definition
public abstract class GeometricObject
{
//data type declaration
private String color = "while";
private boolean filled;
private java.util.Date dateCreated;
// Construct a default geometric object
protected GeometricObject()
{
dateCreated = new java.util.Date();
}
/* Construct a geometric object with color and filled value */
protected GeometricObject(String color, boolean filled)
{
dateCreated = new java.util.Date();
//assign color and fill
this.color = color;
this.filled = filled;
}
// Return color
public String getColor()
{
return color;
}
// Set a new color
public void setColor(String color)
{
this.color = color;
}
/* isFilled method returns filled and Since filled is boolean,the get method is named isFilled */
public boolean isFilled()
{
return filled;
}
// Set a new filled
public void setFilled(boolean filled)
{
this.filled = filled;
}
// Get dateCreated
public java.util.Date getDateCreated()
{
return dateCreated;
}
//override toString function to return output
@Override
public String toString()
{
return "created on " + dateCreated + "\ncolor: " + color +
" and filled: " + filled;
}
// define Abstract method getArea
public abstract double getArea();
//Define Abstract method getPerimeter
public abstract double getPerimeter();
}
Filename: Triangle.Java
/*Class definition of Triangle that extends the class GeometricObject */
public class Triangle extends GeometricObject
{
//declare the three sides of the triangle
private double side1;
private double side2;
private double side3;
//triangle constructor
public Triangle()
{
}
//assign values to all three sides of the triangle
public Triangle(double side1, double side2, double side3)
{
this.side1 = side1;
this.side2 = side2;
this.side3 = side3;
}
//assign triangle values to all attributes
public Triangle(double side1, double side2, double side3, String color, boolean filled)
{
this(side1, side2, side3);
setColor(color);
setFilled(filled);
}
// Return side1
public double getSide1()
{
return side1;
}
// Set side1 to a new length
public void setSide1(double side1)
{
this.side1 = side1;
}
// Return side2
public double getSide2()
{
return side2;
}
// Set side2 to a new length
public void setSide2(double side2)
{
this.side2 = side2;
}
// Return side3
public double getSide3()
{
return side3;
}
// Set side3 to a new length
public void setSide3(double side3)
{
this.side3 = side3;
}
// Function to Return area of the Triangle
@Override
public double getArea()
{
double s = (side1 + side2 + side3) / 2;
return Math.sqrt(s * (s - side1) * (s - side2) * (s - side3));
}
/* override function to Return perimeter of the triangle */
@Override
public double getPerimeter()
{
return side1 + side2 + side3;
}
/*override function to Return a string description of the object*/
@Override
public String toString()
{
return super.toString() + "\nArea: " + getArea() + "\nPerimeter: " + getPerimeter();
}
}
UML Diagram:
Explanation:
- Here, GeometricObject is an abstract class that extends the triangle class which is represented using an arrow. The triangle class then contains a rectangle that represents the objects used to input the three sides of the triangle.
- The remaining part is used to represent all the methods and functions used in the triangle class.
- The Triangle() method creates a triangle with default sides. The method Triangle(side1:double, side2; double,side3;double) creates a triangle of the specified sides.
- Then the respective sides of the triangles are returned using getSide() methods.
- Then the method getArea() returns the area of the triangle and the method getPerimeter() returns the perimeter of the triangle and the function toString() returns a string description of the object.
Enter three side of the triangle: 3
4
5
Enter a color: red
Is the triangle filled (true / false)? false
created on Sun Jul 08 13:24:03 IST 2018
color: red and filled: false
Area: 6.0
Perimeter: 12.0
Area: 6.0
Perimeter: 12.0
Color: red
Triangle is not filled
Want to see more full solutions like this?
Chapter 13 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Modern Database Management
SURVEY OF OPERATING SYSTEMS
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting Out with C++ from Control Structures to Objects (9th Edition)
- A controller is required for a home security alarm, providing the followingfunctionality. The alarm does nothing while it is disarmed (‘switched off’). It canbe armed (‘switched on’) by entering a PIN on the keypad. Whenever thealarm is armed, it can be disarmed by entering the PIN on the keypad.If motion is detected while the alarm is armed, the siren should sound AND asingle SMS message sent to the police to notify them. Further motion shouldnot result in more messages being sent. If the siren is sounding, it can only bedisarmed by entering the PIN on the keypad. Once the alarm is disarmed, asingle SMS should be sent to the police to notify them.Two (active-high) input signals are provided to the controller:MOTION: Asserted while motion is detected inside the home.PIN: Asserted for a single clock cycle whenever the PIN has beencorrectly entered on the keypad.The controller must provide two (active-high) outputs:SIREN: The siren sounds while this output is asserted.POLICE: One SMS…arrow_forward4G+ Vo) % 1.1. LTE1 : Q B NIS شوز طبي ۱:۱۷ کا A X حاز هذا على إعجاب Mohamed Bashar. MEDICAL SHOE شوز طبي ممول . اقوى عرض بالعراق بلاش سعر القطعة ١٥ الف سعر القطعتين ٢٥ الف سعر 3 قطع ٣٥ الف القياسات : 40-41-42-43-44- افحص وكدر ثم ادفع خدمة التوصيل 5 الف لكافة محافظات العراق ופרסם BNI SH ופרסם DON JU WORLD DON JU MORISO DON JU إرسال رسالة III Messenger التواصل مع شوز طبي تعليق باسم اواب حمیدarrow_forwardA manipulator is identified by the following table of parameters and variables:a. Obtain the transformation matrices between adjacent coordinate frames and calculate the global transformation matrix.arrow_forward
- Which tool takes the 2 provided input datasets and produces the following output dataset? Input 1: Record First Last Output: 1 Enzo Cordova Record 2 Maggie Freelund Input 2: Record Frist Last MI ? First 1 Enzo Last MI Cordova [Null] 2 Maggie Freelund [Null] 3 Jason Wayans T. 4 Ruby Landry [Null] 1 Jason Wayans T. 5 Devonn Unger [Null] 2 Ruby Landry [Null] 6 Bradley Freelund [Null] 3 Devonn Unger [Null] 4 Bradley Freelund [Null] OA. Append Fields O B. Union OC. Join OD. Find Replace Clear selectionarrow_forwardWhat are the similarities and differences between massively parallel processing systems and grid computing. with referencesarrow_forwardModular Program Structure. Analysis of Structured Programming Examples. Ways to Reduce Coupling. Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forward
- Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forwardBased on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forwardQuestion: Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forward
- 23:12 Chegg content://org.teleg + 5G 5G 80% New question A feed of 60 mol% methanol in water at 1 atm is to be separated by dislation into a liquid distilate containing 98 mol% methanol and a bottom containing 96 mol% water. Enthalpy and equilibrium data for the mixture at 1 atm are given in Table Q2 below. Ask an expert (a) Devise a procedure, using the enthalpy-concentration diagram, to determine the minimum number of equilibrium trays for the condition of total reflux and the required separation. Show individual equilibrium trays using the the lines. Comment on why the value is Independent of the food condition. Recent My stuff Mol% MeOH, Saturated vapour Table Q2 Methanol-water vapour liquid equilibrium and enthalpy data for 1 atm Enthalpy above C˚C Equilibrium dala Mol% MeOH in Saturated liquid TC kJ mol T. "Chk kot) Liquid T, "C 0.0 100.0 48.195 100.0 7.536 0.0 0.0 100.0 5.0 90.9 47,730 928 7,141 2.0 13.4 96.4 Perks 10.0 97.7 47,311 87.7 8,862 4.0 23.0 93.5 16.0 96.2 46,892 84.4…arrow_forwardYou are working with a database table that contains customer data. The table includes columns about customer location such as city, state, and country. You want to retrieve the first 3 letters of each country name. You decide to use the SUBSTR function to retrieve the first 3 letters of each country name, and use the AS command to store the result in a new column called new_country. You write the SQL query below. Add a statement to your SQL query that will retrieve the first 3 letters of each country name and store the result in a new column as new_country.arrow_forwardWe are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7). A code breaker manages to factories 247 = 13 x 19 Determine Alice's secret key. To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
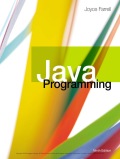
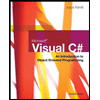
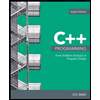
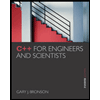
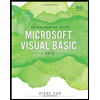