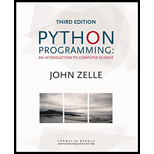
Tracking conference attendees
Program plan:
- In a file “atmmanager.py”,
- Create a class “ATMAp” that calls account information from JSON file and modifies it according to the commands given through an interface class.
- Define the function “_init_()” to create a list of users and manage the account information.
- Define the “run()” function to execute the transaction according to user input.
- Define the function “screen_1()” to return the user input.
- Define the function “screen_2()” to process the transaction according to the user input.
- Define the function “get_User()” to return the user with the given user id.
- Define the function “send_Money()” to move the amount from one user to another. There are two types of accounts are used; “Checking” and “Savings”.
- Define the function “_updateAccounts()” to update the amount in output file whenever the transaction has occurred.
- Define the function “verify()” to check whether the userid and pin given by the user is matched with the saved userid and pin.
- Create a class “User”,
- Define the function “_init_()” method to assign the initial values.
- Define the function “check_Balances()” to return the amount of balance in checking and savings account.
- Define the function “exchange_Cash()” to deposit or withdraw amount fro checking or savings account.
- Define the function “transfer_Money()” to transfer the amount between checking and savings account.
- Define the function “getID()” to return the user id.
- Define the function “getPIN()” to return the pin.
- Create a class “ATMAp” that calls account information from JSON file and modifies it according to the commands given through an interface class.
- In the file “textatm.py”,
- Create a class “TextInterface”,
- Define “_init_()” method to assign initial values.
- Define the function “get_UserInput()” to return the user inputs.
- Define the function “choose_Transaction()” to select the type of transaction.
- Define the function “display_Balanace()” to print the amount in savings and checking accounts.
- Define the function “withdraw_Cash()” to return the withdraw amount and account type.
- Define the function “getTransferInfo()” to return the information of amount transfer between checking and savings account.
- Define the function “getSendInfo()” to return the information of receiver amount transfer.
- Define the function “close()” to exit the transaction.
- Create a class “TextInterface”,
- In a file “atm.py”,
- Import necessary packages.
- Define the “main()” function,
- All the interface class “TextInterface”.
- Call the “ATMAp” class functions with the argument of file and interface.
- Call the “main()” function.

This Python program is to keep track of conference attendees.
Explanation of Solution
Program:
File name: “atmmanager.py”
#Import package
import json
#Create a class
class ATMAp:
#Define the function
def __init__(self, account_file, interface):
#Open the file
with open(account_file) as fi:
#Serialize the object as a json stream
data = json.load(fi)
#Create a list of users
self.users = []
'''Create for loop to iterate key, value pairs till the list of tuples'''
for key,value in data['users'].items():
#Assign the value
usr = User(value["userid"], value["pin"],value["checkin"],value["saving"], value["name"])
#Append to the list
self.users.append(usr)
#Assign the value
self.interface = interface
#Call the run()
self.run()
#Define the run() function
def run(self):
#Execute while loop
while not self.interface.close():
#Call the functions
self.screen_1()
self.screen_2()
#Define the function
def screen_1(self):
#Assign the values
user_id = None
pin = None
#Execute while loop
while not self.verify(user_id, pin):
#Make simultaneous assignment
user_id, pin = self.interface.get_UserInput()
#Assign the value return from get_USer()
self.user = self.get_User(user_id)
#Define the function
def screen_2(self):
#Assign the value
terminate = self.interface.close()
#Execute while loop
while not terminate:
#Make simultaneous assignment
checkin, saving = self.user.check_Balances()
#Assign the value
tran_type = self.interface.choose_Transaction()
#Call the function
self.__updateAccounts()
#Execute the condition
if tran_type[0] == "C":
#Call the function
self.interface.display_Balance(checkin, saving)
#Execute the condition
elif tran_type[0] == "W":
#Make simultaneous assignment
value, account_type = self.interface.withdraw_Cash()
#Call the function
self.user.exchange_Cash(value, account_type)
#Execute the condition
elif tran_type[0] == "T":
#Make simultaneous assignment
inaccount, outaccount, value = self.interface.getTransferInfo()
#Call the function
self.user.transfer_Money(inaccount, outaccount, value)
#Execute the condition
elif tran_type[0] == "S":
#Make the assignment
recipient, type_sender, type_recipient, value = self.interface.getSendInfo()
#Assign the value return from get_User()
recipient = self.get_User(recipient)
#Call the function
self.send_Money(self.user, recipient, value, type_sender, type_recipient)
#Execute the condition
elif tran_type[0] == "Q":
#Call the function
self.user.exchangeCash(-100, "Checking")
#Execute the condition
elif tran_type[0] == "E":
#Call the unction
self.__updateAccounts()
#Assign the boolean value
terminate = True
#Define the function
def get_User(self, userid):
#Create for loop
for user in self.users:
#Check the condition
if userid == user.getID():
#Return the user object for the given userid
return user
#Define the function
def send_Money(self, sender, recipient, value, type_sender, type_recipient):
#Assign the values return from check_Balances()
checking, savings = sender.check_Balances()
checking, savings = recipient.check_Balances()
#Call the functions
sender.exchange_Cash(-value, type_sender)
recipient.exchange_Cash(value, type_recipient)
#Define the function
def __updateAccounts(self):
#Create dictionary
data = {}
#Assign empty dictionary
data['users'] = {}
#Create for loop
for usr in self.users:
#Assign the values
json_str = (vars(usr))
user_id = usr.getID()
#Assign the value
data['users'][user_id] = json_str
#Open the output file in write mode
with open('new_atmusers.json', 'w') as f:
#Serialize the objecct as a json stream
json.dump(data, f, indent=4)
#Define the function
def verify(self, user_id, pin):
#Create for loop
for usr in self.users:
#Execute the condition
if (usr.getID() == user_id) and (usr.getPIN() == pin):
#Return boolean value
return True
#Otherwise
else:
#Return boolean value
return False
#Create a class
class User:
#Define _init_() method
def __init__(self, userid, pin, checkin, saving, name):
#Assign initial values
self.userid = userid
self.pin = pin
self.checkin = float(checkin)
self.saving = float(saving)
self.name = name
#Define the function
def check_Balances(self):
#Return two values
return self.checkin, self.saving
#Define the function
def exchange_Cash(self, value, inaccount):
#Check the condition
if inaccount[0] == "C":
#Assign the value
self.checkin = round(self.checkin + value, 2)
else:
#Assign the value
self.saving = round(self.saving + value, 2)
#Define the function
def transfer_Money(self, inaccount, outaccount, value):
#Check the condition
if inaccount[0] == "C":
#Assign the values
self.checkin = self.checkin + value
self.saving = self.saving - value
#Otherwise
else:
#Assign the values
self.checkin = self.checkin - value
self.saving = self.saving + value
#Define the function
def getID(self):
"""Returns userid"""
#Return the value
return self.userid
#Define the function
def getPIN(self):
#Return the value
return self.pin
File name: “textatm.py”
#Create a class
class TextInterface:
#Define the function
def __init__(self):
#Assign boolean value
self.active = True
#Print the string
print("Welcome to the ATM")
#Define the function
def get_UserInput(self):
#Get user inputs
a = input("Username >> ")
b = input("Pin >> ")
#Return the values
return a, b
#Define the function
def choose_Transaction(self):
#Display user balance
#Assign the value
tran = "xxx"
#Execute the loop
while tran[0] != ("C" or "W" or "T" or "S" or "E" or "Q"):
#Get the choice
tran = input('Please select a transaction from the approved list. "Check Balance", \n "Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> ')
#Check the condition
if tran[0] != "E":
#Return the choice
return tran
else:
#Assign boolean value
self.active = False
#Return the choice
return tran
#Define the function
def display_Balance(self, checking, savings):
#Print savings and checking
print("Checking: {0} Savings: {1}".format(checking, savings))
#Define the function
def withdraw_Cash(self):
#Print the string
print("This function will withdraw or deposit cash.")
#Get the values
value = eval(input("Enter the amount to deposit/withdraw? Enter negative value to withdraw. >> "))
account = input("Checking or Savings account? >> ")
#Print the string
print("Transaction is complete.\n")
#Retuen the values
return value, account
#Define the function
def getTransferInfo(self):
#Get the inputs
outaccount = input("Select account to withdraw money from: >> ")
inaccount = input("Select account to deposit money in: >> ")
value = eval(input("How much would you like to move?"))
#Print the string
print("Transaction is complete.\n")
#Return the values
return inaccount, outaccount, value
#Define the function
def getSendInfo(self):
#Get the inputs
recipient = input("Recipient userid")
type_sender = input("Sender: Checking or Savings? >> ")
type_recipient = input("Recipient: Checking or Savings? >> ")
value = eval(input("How much to send? >> "))
#Print the string
print("Transaction is complete.\n")
#Return the values
return recipient, type_sender, type_recipient, value
#Define the function
def close(self):
#Execute the condition
if self.active == False:
#Print the string
print("\nPlease come again.")
#Return boolean value
return True
#Otherwise
else:
#Return boolean value
return False
File name: “atm.py”
#Import packages
from atmmanager import ATMAp, User
from textatm import TextInterface
#Define the function
def main():
#Assign the value return from TextInterface()
interface = TextInterface()
#Assign the value return from ATMApp class
app = ATMAp("new_atmusers.json", interface)
#Call the function
main()
Content of “new_atmusers.json”:
{
"users": {
"xxxx": {
"userid": "xxxx",
"pin": "1111",
"checkin": 2000.0,
"saving": 11000.0,
"name": "Christina"
},
"ricanontherun": {
"userid": "ricanontherun",
"pin": "2112",
"checkin": 8755.0,
"saving": 7030.0,
"name": "Christian"
},
"theintimidator": {
"userid": "theintimidator",
"pin": "3113",
"checkin": 1845.0,
"saving": 112.0,
"name": "Sean"
},
"jazzplusjazzequalsjazz": {
"userid": "jazzplusjazzequalsjazz",
"pin": "4114",
"checkin": 4489.0,
"saving": 24000.0,
"name": "Dan"
}
}
}
Output:
Welcome to the ATM
Username >> xxxx
Pin >> 1111
Please select a transaction from the approved list. "Check Balance",
"Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> Check Balance
Checking: 2000.0 Savings: 11000.0
Please select a transaction from the approved list. "Check Balance",
"Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> Exit
Please come again.
>>>
Additional output:
Welcome to the ATM
Username >> xxxx
Pin >> 1111
Please select a transaction from the approved list. "Check Balance",
"Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> Withdraw/Deposit Cash
This function will withdraw or deposit cash.
Enter the amount to deposit/withdraw? Enter negative value to withdraw. >> 100
Checking or Savings account? >> Checking
Transaction is complete.
Please select a transaction from the approved list. "Check Balance",
"Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> Exit
Please come again.
>>>
Want to see more full solutions like this?
Chapter 12 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- This week we will be building a regression model conceptually for our discussion assignment. Consider your current workplace (or previous/future workplace if not currently working) and answer the following set of questions. Expand where needed to help others understand your thinking: What is the most important factor (variable) that needs to be predicted accurately at work? Why? Justify its selection as your dependent variable.arrow_forwardAccording to best practices, you should always make a copy of a config file and add a date to filename before editing? Explain why this should be done and what could be the pitfalls of not doing it.arrow_forwardIn completing this report, you may be required to rely heavily on principles relevant, for example, the Work System, Managerial and Functional Levels, Information and International Systems, and Security. apply Problem Solving Techniques (Think Outside The Box) when completing. should reflect relevance, clarity, and organisation based on research. Your research must be demonstrated by Details from the scenario to support your analysis, Theories from your readings, Three or more scholarly references are required from books, UWIlinc, etc, in-text or narrated citations of at least four (4) references. “Implementation of an Integrated Inventory Management System at Green Fields Manufacturing” Green Fields Manufacturing is a mid-sized company specialising in eco-friendly home and garden products. In recent years, growing demand has exposed the limitations of their fragmented processes and outdated systems. Different departments manage production schedules, raw material requirements, and…arrow_forward
- 1. Create a Book record that implements the Comparable interface, comparing the Book objects by year - title: String > - author: String - year: int Book + compareTo(other Book: Book): int + toString(): String Submit your source code on Canvas (Copy your code to text box or upload.java file) > Comparable 2. Create a main method in Book record. 1) In the main method, create an array of 2 objects of Book with your choice of title, author, and year. 2) Sort the array by year 3) Print the object. Override the toString in Book to match the example output: @Javadoc Declaration Console X Properties Book [Java Application] /Users/kuan/.p2/pool/plugins/org.eclipse.justj.openjdk.hotspo [Book: year=1901, Book: year=2010]arrow_forwardQ5-The efficiency of a 200 KVA, single phase transformer is 98% when operating at full load 0.8 lagging p.f. the iron losses in the transformer is 2000 watt. Calculate the i) Full load copper losses ii) half load copper losses and efficiency at half load. Ans: 1265.306 watt, 97.186%arrow_forward2. Consider the following pseudocode for partition: function partition (A,L,R) pivotkey = A [R] t = L for i L to R-1 inclusive: if A[i] A[i] t = t + 1 end if end for A [t] A[R] return t end function Suppose we call partition (A,0,5) on A=[10,1,9,2,8,5]. Show the state of the list at the indicated instances. Initial A After i=0 ends After 1 ends After i 2 ends After i = 3 ends After i = 4 ends After final swap 10 19 285 [12 pts]arrow_forward
- .NET Interactive Solving Sudoku using Grover's Algorithm We will now solve a simple problem using Grover's algorithm, for which we do not necessarily know the solution beforehand. Our problem is a 2x2 binary sudoku, which in our case has two simple rules: •No column may contain the same value twice •No row may contain the same value twice If we assign each square in our sudoku to a variable like so: 1 V V₁ V3 V2 we want our circuit to output a solution to this sudoku. Note that, while this approach of using Grover's algorithm to solve this problem is not practical (you can probably find the solution in your head!), the purpose of this example is to demonstrate the conversion of classical decision problems into oracles for Grover's algorithm. Turning the Problem into a Circuit We want to create an oracle that will help us solve this problem, and we will start by creating a circuit that identifies a correct solution, we simply need to create a classical function on a quantum circuit that…arrow_forwardusing r languagearrow_forward8. Cash RegisterThis exercise assumes you have created the RetailItem class for Programming Exercise 5. Create a CashRegister class that can be used with the RetailItem class. The CashRegister class should be able to internally keep a list of RetailItem objects. The class should have the following methods: A method named purchase_item that accepts a RetailItem object as an argument. Each time the purchase_item method is called, the RetailItem object that is passed as an argument should be added to the list. A method named get_total that returns the total price of all the RetailItem objects stored in the CashRegister object’s internal list. A method named show_items that displays data about the RetailItem objects stored in the CashRegister object’s internal list. A method named clear that should clear the CashRegister object’s internal list. Demonstrate the CashRegister class in a program that allows the user to select several items for purchase. When the user is ready to check out, the…arrow_forward
- 5. RetailItem ClassWrite a class named RetailItem that holds data about an item in a retail store. The class should store the following data in attributes: item description, units in inventory, and price. Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them: Description Units in Inventory PriceItem #1 Jacket 12 59.95Item #2 Designer Jeans 40 34.95Item #3 Shirt 20 24.95arrow_forwardFind the Error: class Information: def __init__(self, name, address, age, phone_number): self.__name = name self.__address = address self.__age = age self.__phone_number = phone_number def main(): my_info = Information('John Doe','111 My Street', \ '555-555-1281')arrow_forwardFind the Error: class Pet def __init__(self, name, animal_type, age) self.__name = name; self.__animal_type = animal_type self.__age = age def set_name(self, name) self.__name = name def set_animal_type(self, animal_type) self.__animal_type = animal_typearrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
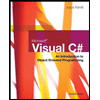
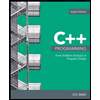
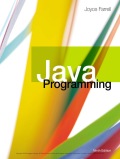
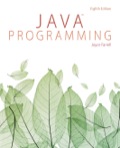
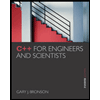