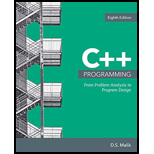
Explanation of Solution
The program has been explained in the in-lined comments:
#include <iostream>
using namespace std;
int main()
{
//delcare a list and initialize it
//with an array of 6 elements
int numList[6] = {25, 37, 62, 78, 92, 13};
//create a pointer to the list -
//the base address of the list is assigned
//to the pointer
int *listPtr = numList;
//a new pointer is declared and is assigned
//an address 2 int positions incremented i.e
//it points to the 3rd position in the array
//or numlist[2] which is the value 62
int *temp = listPtr + 2;
//declare an int variable
int num;
//assign value of the memory address pointed to
//by listPtr with the arithmetic expression on the
//RHS of the assignment operator
//RHS = *(listPtr + 1) - *listPtr =
//numList[1] - numList[0] = 37-25 = 12
//since listPtr is numList[0] so now the array elements
//are {12, 37, 62, 78, 92, 13}
*listPtr = *(listPtr + 1) - *listPtr;
//listPtr is incremented and now points to numList[1]
// i.e. 37
listPtr++;
//num is assigned the value pointed to by temp which is
//numList[2] i.e. 62
num = *temp;
//temp is incrementedand now points to numList[3]
//i...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
C++ Programming: From Problem Analysis To Program Design, Loose-leaf Version
- using r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardThe assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of…arrow_forwardI need help to solve the following case, thank youarrow_forward
- You will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forwardConstruct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
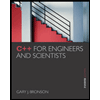
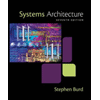
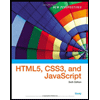
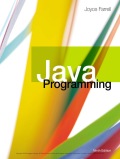
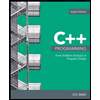