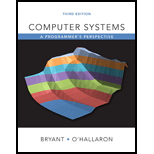
Concept explainers
Explanation of Solution
Implementation of a concurrent version of the TINY web server on I/O threads:
Modified “main.c” code:
#include "csapp.h"
//Function declaration
void doit(int fd);
void read_requesthdrs(rio_t *rp);
int parse_uri(char *uri, char *filename, char *cgiargs);
void serve_static(int fd, char *filename, int filesize);
void get_filetype(char *filename, char *filetype);
void serve_dynamic(int fd, char *filename, char *cgiargs);
void clienterror(int fd, char *cause, char *errnum,char *shortmsg, char *longmsg);
void *thread(void *vargp);
//Main function
int main(int argc, char **argv)
{
//Declare variable
int listenfd, connfd;
int *connfdp;
pthread_t tid;
char hostname[MAXLINE], port[MAXLINE];
socklen_t clientlen;
struct sockaddr_storage clientaddr;
/* Check the command line argument. if the argument does not satify, then */
if (argc != 2)
{
//Display the below error message
fprintf(stderr, "usage: %s <port>\n", argv[0]);
fprintf(stderr, "use default port 5000\n");
listenfd = Open_listenfd("5000");
}
//Otherwise,
else
{
//Call the Open_listenfd method
listenfd = Open_listenfd(argv[1]);
}
//Check condition
while (1)
{
//Get the length the client address
clientlen = sizeof(clientaddr);
//For connection accept
connfd = Accept(listenfd, (SA *)&clientaddr, &clientlen);
//Call the method
Getnameinfo((SA *) &clientaddr, clientlen, hostname, MAXLINE,
port, MAXLINE, 0);
//Display the accepted connection
printf("Accepted connection from (%s, %s)\n", hostname, port);
connfdp = (int*)Malloc(sizeof(int));
*connfdp = connfd;
//Call Pthread_create method
Pthread_create(&tid, NULL, thread, connfdp);
}
}
//Function definition for thread method
void *thread(void *vargp)
{
//Compute the connection file descriptor number
int connfd = *(int*)vargp;
//Call pthread detach method
Pthread_detach(Pthread_self());
//Free the given file
Free(vargp);
//Call doit method
doit(connfd);
//Close the connection
Close(connfd);
return NULL;
}
/* Function definition for doit method that is for handle HTTP request or response transfer */
void doit(int fd)
{
//Declare variable
int is_static;
struct stat sbuf;
char buf[MAXLINE], method[MAXLINE], uri[MAXLINE], version[MAXLINE];
char filename[MAXLINE], cgiargs[MAXLINE];
//Create object for rio function
rio_t rio;
/* Read request line and headers */
Rio_readinitb(&rio, fd);
//Check read request
if (!Rio_readlineb(&rio, buf, MAXLINE))
return;
//Display the buffer
printf("%s", buf);
//Display the parse request
sscanf(buf, "%s %s %s", method, uri, version);
//Check condition
if (strcasecmp(method, "GET"))
{
//Call client error method
clienterror(fd, method, "501", "Not Implemented","Tiny does not implement this method");
return;
}
//Call read_requestdrs method
read_requesthdrs(&rio);
/* Parse URI from GET request */
is_static = parse_uri(uri, filename, cgiargs);
//Check the static connection
if (stat(filename, &sbuf) < 0)
{
//Call client error method
clienterror(fd, filename, "404", "Not found",
"Tiny couldn't find this file");
return;
}
/* Check serve static content */
if (is_static)
{
if (!(S_ISREG(sbuf.st_mode)) || !(S_IRUSR & sbuf.st_mode))
{
//Call client error method
clienterror(fd, filename, "403", "Forbidden","Tiny couldn't read the file");
return;
}
//Call serve_static method
serve_static(fd, filename, sbuf.st_size);
}
//Otherwise check the serve dynamic content
else
{
if (!(S_ISREG(sbuf.st_mode)) || !(S_IXUSR & sbuf.st_mode))
{
//Call client error method
clienterror(fd, filename, "403", "Forbidden","Tiny couldn't run the CGI program");
return;
}
//Call serve dynamic method
serve_dynamic(fd, filename, cgiargs);
}
}
/* Function definition for read_requestdrs that is for read HTTP request headers */
void read_requesthdrs(rio_t *rp)
{
//Declare variable
char buf[MAXLINE];
Rio_readlineb(rp, buf, MAXLINE);
printf("%s", buf);
//Check condition
while(strcmp(buf, "\r\n"))
{
//Call rio readlineb function
Rio_readlineb(rp, buf, MAXLINE);
printf("%s", buf);
}
return;
}
/* Function definition for parse uri into file nane and cgi argument */
int parse_uri(char *uri, char *filename, char *cgiargs)
{
//Declare char variable
char *ptr;
//Check content type...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- This week we will be building a regression model conceptually for our discussion assignment. Consider your current workplace (or previous/future workplace if not currently working) and answer the following set of questions. Expand where needed to help others understand your thinking: What is the most important factor (variable) that needs to be predicted accurately at work? Why? Justify its selection as your dependent variable.arrow_forwardAccording to best practices, you should always make a copy of a config file and add a date to filename before editing? Explain why this should be done and what could be the pitfalls of not doing it.arrow_forwardIn completing this report, you may be required to rely heavily on principles relevant, for example, the Work System, Managerial and Functional Levels, Information and International Systems, and Security. apply Problem Solving Techniques (Think Outside The Box) when completing. should reflect relevance, clarity, and organisation based on research. Your research must be demonstrated by Details from the scenario to support your analysis, Theories from your readings, Three or more scholarly references are required from books, UWIlinc, etc, in-text or narrated citations of at least four (4) references. “Implementation of an Integrated Inventory Management System at Green Fields Manufacturing” Green Fields Manufacturing is a mid-sized company specialising in eco-friendly home and garden products. In recent years, growing demand has exposed the limitations of their fragmented processes and outdated systems. Different departments manage production schedules, raw material requirements, and…arrow_forward
- 1. Create a Book record that implements the Comparable interface, comparing the Book objects by year - title: String > - author: String - year: int Book + compareTo(other Book: Book): int + toString(): String Submit your source code on Canvas (Copy your code to text box or upload.java file) > Comparable 2. Create a main method in Book record. 1) In the main method, create an array of 2 objects of Book with your choice of title, author, and year. 2) Sort the array by year 3) Print the object. Override the toString in Book to match the example output: @Javadoc Declaration Console X Properties Book [Java Application] /Users/kuan/.p2/pool/plugins/org.eclipse.justj.openjdk.hotspo [Book: year=1901, Book: year=2010]arrow_forwardQ5-The efficiency of a 200 KVA, single phase transformer is 98% when operating at full load 0.8 lagging p.f. the iron losses in the transformer is 2000 watt. Calculate the i) Full load copper losses ii) half load copper losses and efficiency at half load. Ans: 1265.306 watt, 97.186%arrow_forward2. Consider the following pseudocode for partition: function partition (A,L,R) pivotkey = A [R] t = L for i L to R-1 inclusive: if A[i] A[i] t = t + 1 end if end for A [t] A[R] return t end function Suppose we call partition (A,0,5) on A=[10,1,9,2,8,5]. Show the state of the list at the indicated instances. Initial A After i=0 ends After 1 ends After i 2 ends After i = 3 ends After i = 4 ends After final swap 10 19 285 [12 pts]arrow_forward
- .NET Interactive Solving Sudoku using Grover's Algorithm We will now solve a simple problem using Grover's algorithm, for which we do not necessarily know the solution beforehand. Our problem is a 2x2 binary sudoku, which in our case has two simple rules: •No column may contain the same value twice •No row may contain the same value twice If we assign each square in our sudoku to a variable like so: 1 V V₁ V3 V2 we want our circuit to output a solution to this sudoku. Note that, while this approach of using Grover's algorithm to solve this problem is not practical (you can probably find the solution in your head!), the purpose of this example is to demonstrate the conversion of classical decision problems into oracles for Grover's algorithm. Turning the Problem into a Circuit We want to create an oracle that will help us solve this problem, and we will start by creating a circuit that identifies a correct solution, we simply need to create a classical function on a quantum circuit that…arrow_forwardusing r languagearrow_forward8. Cash RegisterThis exercise assumes you have created the RetailItem class for Programming Exercise 5. Create a CashRegister class that can be used with the RetailItem class. The CashRegister class should be able to internally keep a list of RetailItem objects. The class should have the following methods: A method named purchase_item that accepts a RetailItem object as an argument. Each time the purchase_item method is called, the RetailItem object that is passed as an argument should be added to the list. A method named get_total that returns the total price of all the RetailItem objects stored in the CashRegister object’s internal list. A method named show_items that displays data about the RetailItem objects stored in the CashRegister object’s internal list. A method named clear that should clear the CashRegister object’s internal list. Demonstrate the CashRegister class in a program that allows the user to select several items for purchase. When the user is ready to check out, the…arrow_forward
- 5. RetailItem ClassWrite a class named RetailItem that holds data about an item in a retail store. The class should store the following data in attributes: item description, units in inventory, and price. Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them: Description Units in Inventory PriceItem #1 Jacket 12 59.95Item #2 Designer Jeans 40 34.95Item #3 Shirt 20 24.95arrow_forwardFind the Error: class Information: def __init__(self, name, address, age, phone_number): self.__name = name self.__address = address self.__age = age self.__phone_number = phone_number def main(): my_info = Information('John Doe','111 My Street', \ '555-555-1281')arrow_forwardFind the Error: class Pet def __init__(self, name, animal_type, age) self.__name = name; self.__animal_type = animal_type self.__age = age def set_name(self, name) self.__name = name def set_animal_type(self, animal_type) self.__animal_type = animal_typearrow_forward
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningLINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.Computer ScienceISBN:9781337569798Author:ECKERTPublisher:CENGAGE LC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
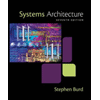
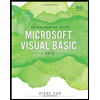
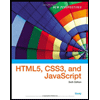
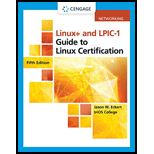
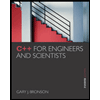