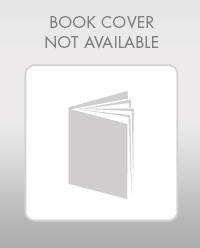
Concept explainers
What will the following
#include <iostream>
#include <memory>
using namespace std;
class Base
{
public:
Base () { cout << "Entering the base .\n"; }
virtual ~Base () { cout << "Leaving the base.\n"; }
} ;
class Camp : public Base
{
public :
Camp() { cout << "Entering the camp.\n"; }
virtual ~Camp() { cout << "Leaving the camp.\n"; }
};
int main()
{
shared _ptr<Camp> outpost = make_shared<Camp> ();
return 0;
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
Starting Out With C++: Early Objects (10th Edition)
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Modern Database Management
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Introduction To Programming Using Visual Basic (11th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
- #include <iostream> using namespace std; class Creature { public: Creature(); void run() const; protected: int distance;}; Creature::Creature(): distance(10){} void Creature::run() const{ cout << "running " << distance << " meters!\n";} class Wizard : public Creature { public: Wizard(); void hover() const; private: int distFactor;}; Wizard::Wizard() : distFactor(3){} void Wizard::hover() const{ cout << "hovering " << (distFactor * distance) << " meters!\n";} int main(){ cout << "Creating an Creature.\n"; Creature c; c.run(); cout << "\nCreating a Wizard.\n"; Wizard w; w.run(); w.hover(); return 0;}arrow_forward#include <iostream> using namespace std; class Rectangle { // define class public: Rectangle() { length = 0; width = 0; } // constructor float getlength() { return length; } // return length float getWidth() { return width; } // return width float area() { return length* width; } // return area float perimeter() { return 2 * length + 2 * width; } void setValues(float, float); private: float length, width; }; void Rectangle::setValues(float len, float wid) { if (len <= 0 || len >= 20) { cout << "Enter new value for length "; cin >> len; } length = len; if (wid <= 0 || wid >= 20) { cout << "Enter new value for width "; cin >> wid; } width = wid; } int main() { float length, width; Rectangle r; cout << "Enter a value for length and width between 0 and…arrow_forward#include #include using namespace std; class Staff{ public: int code; string name; public: Staff(){} Staff(int code, string name){ this->code = code; this->name = name; } Staff(const Staff &s){ this->code=s.code; this->name = s.name; } int getCode(){ return code; } void setCode(int c){ code = c; } string getName(){ return name; } void setName(string n){ name = n; }; class Faculty: public Staff{ public: int department; string subjectTaken; string researchArea; Faculty(int code,string name,int department, string subjectTaken, string researchArea):Staff(code,name) { this->department = department; this->subjectTaken = subjectTaken; this->researchArea = researchArea; } Faculty() { } Faculty(const Faculty &f){ code = f.code; name = f.name; department = f.department; subjectTaken = f.subjectTaken; researchArea = f.researchArea; } int getDepartment() { return department; } void setDepartment(int department) {…arrow_forward
- Nonearrow_forwardC++arrow_forwardclass Currency { protected: int whole; int fraction; virtual std::string get_name() = 0; public: Currency() { whole = 0; fraction = 0; } Currency(double value) { if (value < 0) throw "Invalid value"; whole = int(value); fraction = std::round(100 * (value - whole)); } Currency(const Currency& curr) { whole = curr.whole; fraction = curr.fraction; } /* This algorithm gets the whole part or fractional part of the currency Pre: whole, fraction - integer numbers Post: Return: whole or fraction */ int get_whole() { return whole; } int get_fraction() { return fraction; } /* This algorithm adds an object to the same currency Pre: object (same currency) Post: Return: */ void set_whole(int w) { if (w >= 0) whole = w; } void set_fraction(int f) { if (f >= 0 && f <…arrow_forward
- 1. Assume the following: class Complex{public:Complex(int, int); //initializes the private data members to the passed in values: // real = value of the first argument // imag = value of the second argumentComplex add( Complex ); //returns a Complex object that holds the sum of two //Complex objectsvoid print(); //prints the values of the private data members in the format: // (real, imag)private:int real, imag;}; Will these two lines work below in a driver program for the class? Complex c1(40,50); //create object (line 1) c1.real=55; //move in value of 55 (line 2) a. yes it will work fine b. no, you will get a compiler error c. need more information on the object d. line 1 will cause an error line 2 will work finearrow_forwardClass Implementation Exercises EX1: Write a complete C++ program to implement a car class. The class has three attributes: Id, speed and color. Also, the class include the following methods: (setter) function, (getter) function, (default and parameterized constructor), and( print) to print the attributes values ➜ Create three objects in the main function then call the method. EX2: Write a complete C++ program to implement a student class. ➜ The class has three attributes:std-Id, Name and marks [3]. Also, the class include the following methods: (setter) function,(getter) functions (default and parameterized constructor), (average) and(print) to print the attributes values ➜ Create array of objects in the main function then call the method.arrow_forwardProgramming Exercise 11-2 dateType.h file provided #ifndef date_H #define date_H class dateType { public: void setDate(int month, int day, int year); //Function to set the date. //The member variables dMonth, dDay, and dYear are set //according to the parameters //Postcondition: dMonth = month; dDay = day; // dYear = year int getDay() const; //Function to return the day. //Postcondition: The value of dDay is returned. int getMonth() const; //Function to return the month. //Postcondition: The value of dMonth is returned. int getYear() const; //Function to return the year. //Postcondition: The value of dYear is returned. void printDate() const; //Function to output the date in the form mm-dd-yyyy. bool isLeapYear(); //Function to determine whether the year is a leap year. dateType(int month = 1, int day = 1, int year = 1900); //Constructor to…arrow_forward
- Make Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forwardMake Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forwardclass Base { public: int x, y: public: Base(int i, int j) { x = i; y = j; }}; class Derived : public Base public: Derived (int i, int j) { x = i; y = j; void display() {cout « x <<" "<< y; }}; int main(void) { Derived d(5, 5); d.display(); return e; Error O X=5,Y=5 X-0,Y=0 None of the above Other:arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
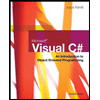