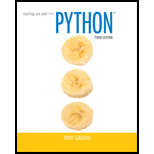
Starting Out with Python (3rd Edition)
3rd Edition
ISBN: 9780133582734
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 11, Problem 4TF
Only the _ _init_ _method can be overridden.
Expert Solution & Answer

Learn your wayIncludes step-by-step video

schedule03:14
Students have asked these similar questions
Need help making python code for this!
2.7 LAB: Smallest of two numbers
Instructor note:
Note: this section of your textbook contains activities that you will complete for points. To ensure your work is scored, please access this page from the assignment link provided in the CTU Virtual Campus. If you did not access this page via the CTU Virtual Campus, please do so now.
I help understanding this question
d'y + 4dy +3y = a, Initial Conditions: y(0) = 5 & y'(0)=0
Where a = 10
a) Find y(t) =yh(t) +yp(t) in time domainIs the system over-damped, under-damped, or critical?
b) Find y(t) using Laplace Transforms
Chapter 11 Solutions
Starting Out with Python (3rd Edition)
Ch. 11.1 - In this section, we discussed superclasses and...Ch. 11.1 - Prob. 2CPCh. 11.1 - What does a subclass inherit from its superclass?Ch. 11.1 - Look at the following code, which is the first...Ch. 11.2 - Look at the following class definitions: class...Ch. 11 - In an inheritance relationship, the ___________ is...Ch. 11 - In an inheritance relationship, the _________ is...Ch. 11 - Suppose a program uses two classes: Airplane and...Ch. 11 - This characteristic of object-oriented programming...Ch. 11 - Prob. 5MC
Ch. 11 - Polymorphism allows you to write methods in a...Ch. 11 - It is not possible to call a superclasss _ _init_...Ch. 11 - A subclass can have a method with the same name as...Ch. 11 - Only the _ _init_ _method can be overridden.Ch. 11 - You cannot use the isinstance function to...Ch. 11 - What does a subclass inherit from its superclass?Ch. 11 - Look at the following class definition. What is...Ch. 11 - Prob. 3SACh. 11 - Write the first line of the definition for a...Ch. 11 - Look at the following class definitions: class...Ch. 11 - Look at the following class definition: class...Ch. 11 - Employee and ProductionWorker Classes Write an...Ch. 11 - ShiftSupervisor Class In a particular factory, a...Ch. 11 - Person and Customer Classes The Person and...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
The ____________ is always transparent.
Web Development and Design Foundations with HTML5 (8th Edition)
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
Write a program segment that defines a file stream object named employees. The file should be opened for both i...
Starting Out with C++ from Control Structures to Objects (9th Edition)
If the Vole executes an instruction every microsecond (a millionth of a second), how long does it take to compl...
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
For the circuit shown, use the node-voltage method to find v1, v2, and i1.
How much power is delivered to the c...
Electric Circuits. (11th Edition)
Distinguish among data definition commands, data manipulation commands, and data control commands.
Modern Database Management
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Given f(t)=a sin(ßt) a = 10 & ß = 23 Find the Laplace Transform using the definition F(s) = ∫f(t)e-stdtarrow_forwardPlease do not use any AI tools to solve this question. I need a fully manual, step-by-step solution with clear explanations, as if it were done by a human tutor. No AI-generated responses, please.arrow_forwardObtain the MUX design for the function F(X,Y,Z) = (0,3,4,7) using an off-the-shelf MUX with an active low strobe input (E).arrow_forward
- I cannot program smart home automation rules from my device using a computer or phone, and I would like to know how to properly connect devices such as switches and sensors together ? Cisco Packet Tracer 1. Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardusing r language for integration theta = integral 0 to infinity (x^4)*e^(-x^2)/2 dx (1) use the density function of standard normal distribution N(0,1) f(x) = 1/sqrt(2pi) * e^(-x^2)/2 -infinity <x<infinity as importance function and obtain an estimate theta 1 for theta set m=100 for the estimate whatt is the estimate theta 1? (2)use the density function of gamma (r=5 λ=1/2)distribution f(x)=λ^r/Γ(r) x^(r-1)e^(-λx) x>=0 as importance function and obtain an estimate theta 2 for theta set m=1000 fir the estimate what is the estimate theta2? (3) use simulation (repeat 1000 times) to estimate the variance of the estimates theta1 and theta 2 which one has smaller variance?arrow_forwardusing r language A continuous random variable X has density function f(x)=1/56(3x^2+4x^3+5x^4).0<=x<=2 (1) secify the density g of the random variable Y you find for the acceptance rejection method. (2) what is the value of c you choose to use for the acceptance rejection method (3) use the acceptance rejection method to generate a random sample of size 1000 from the distribution of X .graph the density histogram of the sample and compare it with the density function f(x)arrow_forward
- using r language a continuous random variable X has density function f(x)=1/4x^3e^-(pi/2)^4,x>=0 derive the probability inverse transformation F^(-1)x where F(x) is the cdf of the random variable Xarrow_forwardusing r language in an accelerated failure test, components are operated under extreme conditions so that a substantial number will fail in a rather short time. in such a test involving two types of microships 600 chips manufactured by an existing process were tested and 125 of them failed then 800 chips manufactured by a new process were tested and 130 of them failed what is the 90%confidence interval for the difference between the proportions of failure for chips manufactured by two processes? using r languagearrow_forwardI want a picture of the tools and the pictures used Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forward
- A. What will be printed executing the code above?B. What is the simplest way to set a variable of the class Full_Date to January 26 2020?C. Are there any empty constructors in this class Full_Date?a. If there is(are) in which code line(s)?b. If there is not, how would an empty constructor be? (create the code lines for it)D. Can the command std::cout << d1.m << std::endl; be included after line 28 withoutcausing an error?a. If it can, what will be printed?b. If it cannot, how could this command be fixed?arrow_forwardCisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardTransform the TM below that accepts words over the alphabet Σ= {a, b} with an even number of a's and b's in order that the output tape head is positioned over the first letter of the input, if the word is accepted, and all letters a should be replaced by the letter x. For example, for the input aabbaa the tape and head at the end should be: [x]xbbxx z/z,R b/b,R F ① a/a,R b/b,R a/a, R a/a,R b/b.R K a/a,R L b/b,Rarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
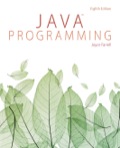
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
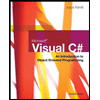
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
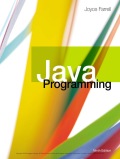
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
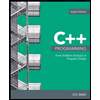
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
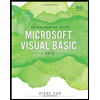
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY