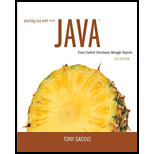
Starting Out with Java: From Control Structures through Objects (6th Edition)
6th Edition
ISBN: 9780133957051
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 11, Problem 4AW
Program Plan Intro
Exception:
An exception is an event which takes place during the execution of a
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Which tool takes the 2 provided input datasets and produces the following output dataset?
Input 1:
Record
First
Last
Output:
1 Enzo
Cordova
Record
2 Maggie
Freelund
Input 2:
Record
Frist
Last
MI
?
First
1 Enzo
Last
MI
Cordova
[Null]
2 Maggie
Freelund
[Null]
3 Jason
Wayans
T.
4 Ruby
Landry
[Null]
1 Jason
Wayans
T.
5 Devonn
Unger
[Null]
2 Ruby
Landry
[Null]
6 Bradley
Freelund
[Null]
3 Devonn
Unger
[Null]
4 Bradley
Freelund
[Null]
OA. Append Fields
O B. Union
OC. Join
OD. Find Replace
Clear selection
What are the similarities and differences between massively parallel processing systems and grid computing. with references
Modular Program Structure. Analysis of Structured Programming Examples. Ways to Reduce Coupling.
Based on the given problem, create an algorithm and a block diagram, and write the program code:
Function: y=xsinx
Interval: [0,π]
Requirements:
Create a graph of the function.
Show the coordinates (x and y).
Choose your own scale and show it in the block diagram.
Create a block diagram based on the algorithm.
Write the program code in Python.
Requirements:
Each step in the block diagram must be clearly shown.
The graph of the function must be drawn and saved (in PNG format).
Write the code in a modular way (functions and the main part should be separate).
Please explain and describe the results in detail.
Chapter 11 Solutions
Starting Out with Java: From Control Structures through Objects (6th Edition)
Ch. 11.1 - Prob. 11.1CPCh. 11.1 - Prob. 11.2CPCh. 11.1 - Prob. 11.3CPCh. 11.1 - Prob. 11.4CPCh. 11.1 - Prob. 11.5CPCh. 11.1 - Prob. 11.6CPCh. 11.1 - Prob. 11.7CPCh. 11.1 - Prob. 11.8CPCh. 11.1 - Prob. 11.9CPCh. 11.1 - When does the code in a finally block execute?
Ch. 11.1 - What is the call stack? What is a stack trace?Ch. 11.1 - Prob. 11.12CPCh. 11.1 - Prob. 11.13CPCh. 11.1 - Prob. 11.14CPCh. 11.2 - What does the throw statement do?Ch. 11.2 - Prob. 11.16CPCh. 11.2 - Prob. 11.17CPCh. 11.2 - Prob. 11.18CPCh. 11.2 - Prob. 11.19CPCh. 11.3 - What is the difference between a text file and a...Ch. 11.3 - What classes do you use to write output to a...Ch. 11.3 - Prob. 11.22CPCh. 11.3 - What class do you use to work with random access...Ch. 11.3 - What are the two modes that a random access file...Ch. 11.3 - Prob. 11.25CPCh. 11 - Prob. 1MCCh. 11 - Prob. 2MCCh. 11 - Prob. 3MCCh. 11 - Prob. 4MCCh. 11 - FileNotFoundException inherits from __________. a....Ch. 11 - Prob. 6MCCh. 11 - Prob. 7MCCh. 11 - Prob. 8MCCh. 11 - Prob. 9MCCh. 11 - Prob. 10MCCh. 11 - Prob. 11MCCh. 11 - Prob. 12MCCh. 11 - Prob. 13MCCh. 11 - Prob. 14MCCh. 11 - Prob. 15MCCh. 11 - This is the process of converting an object to a...Ch. 11 - Prob. 17TFCh. 11 - Prob. 18TFCh. 11 - Prob. 19TFCh. 11 - True or False: You cannot have more than one catch...Ch. 11 - Prob. 21TFCh. 11 - Prob. 22TFCh. 11 - Prob. 23TFCh. 11 - Prob. 24TFCh. 11 - Find the error in each of the following code...Ch. 11 - // Assume inputFile references a Scanner object,...Ch. 11 - Prob. 3FTECh. 11 - Prob. 1AWCh. 11 - Prob. 2AWCh. 11 - Prob. 3AWCh. 11 - Prob. 4AWCh. 11 - Prob. 5AWCh. 11 - Prob. 6AWCh. 11 - The method getValueFromFile is public and returns...Ch. 11 - Prob. 8AWCh. 11 - Write a statement that creates an object that can...Ch. 11 - Write a statement that opens the file...Ch. 11 - Assume that the reference variable r refers to a...Ch. 11 - Prob. 1SACh. 11 - Prob. 2SACh. 11 - Prob. 3SACh. 11 - Prob. 4SACh. 11 - Prob. 5SACh. 11 - Prob. 6SACh. 11 - What types of objects can be thrown?Ch. 11 - Prob. 8SACh. 11 - Prob. 9SACh. 11 - Prob. 10SACh. 11 - What is the difference between a text file and a...Ch. 11 - What is the difference between a sequential access...Ch. 11 - What happens when you serialize an object? What...Ch. 11 - TestScores Class Write a class named TestScores....Ch. 11 - Prob. 2PCCh. 11 - Prob. 3PCCh. 11 - Prob. 4PCCh. 11 - Prob. 5PCCh. 11 - FileArray Class Design a class that has a static...Ch. 11 - File Encryption Filter File encryption is the...Ch. 11 - File Decryption Filter Write a program that...Ch. 11 - TestScores Modification for Serialization Modify...Ch. 11 - Prob. 10PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forwardBased on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forwardQuestion: Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forward
- 23:12 Chegg content://org.teleg + 5G 5G 80% New question A feed of 60 mol% methanol in water at 1 atm is to be separated by dislation into a liquid distilate containing 98 mol% methanol and a bottom containing 96 mol% water. Enthalpy and equilibrium data for the mixture at 1 atm are given in Table Q2 below. Ask an expert (a) Devise a procedure, using the enthalpy-concentration diagram, to determine the minimum number of equilibrium trays for the condition of total reflux and the required separation. Show individual equilibrium trays using the the lines. Comment on why the value is Independent of the food condition. Recent My stuff Mol% MeOH, Saturated vapour Table Q2 Methanol-water vapour liquid equilibrium and enthalpy data for 1 atm Enthalpy above C˚C Equilibrium dala Mol% MeOH in Saturated liquid TC kJ mol T. "Chk kot) Liquid T, "C 0.0 100.0 48.195 100.0 7.536 0.0 0.0 100.0 5.0 90.9 47,730 928 7,141 2.0 13.4 96.4 Perks 10.0 97.7 47,311 87.7 8,862 4.0 23.0 93.5 16.0 96.2 46,892 84.4…arrow_forwardYou are working with a database table that contains customer data. The table includes columns about customer location such as city, state, and country. You want to retrieve the first 3 letters of each country name. You decide to use the SUBSTR function to retrieve the first 3 letters of each country name, and use the AS command to store the result in a new column called new_country. You write the SQL query below. Add a statement to your SQL query that will retrieve the first 3 letters of each country name and store the result in a new column as new_country.arrow_forwardWe are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7). A code breaker manages to factories 247 = 13 x 19 Determine Alice's secret key. To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.arrow_forward
- Consider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.arrow_forwardPllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forwardPllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forward
- Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forwardPllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forward4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
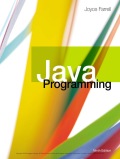
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
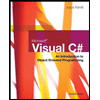
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
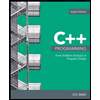
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
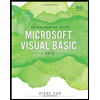
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
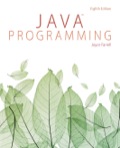
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT