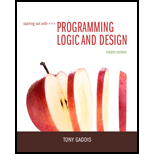
Starting Out with Programming Logic and Design (4th Edition)
4th Edition
ISBN: 9780133985078
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 10, Problem 7AW
Program Plan Intro
The following algorithm is used to delete a record from file containing the student name of “John Perz”.
- Import a package named “os” to use remove and rename functions for files.
- Define “main()” function,
- Declare a variable named “found” and initialize it to be “False”.
- Open an input file named “students.txt” that is available on disk using “open()” function with “r” mode and initialize it into the “fileObject”.
- Open an output file named “temp.txt” using “open()” function with “w” mode and initialize it into the “temp_file”.
- Define a “while” loop to check the file until the value of “name” will be empty.
- Read the line from file using “readline()” method and store the score value into “score”.
- Strip the new line from the value of “name” variable.
- Using “if...else” condition check the name of “John Perz” is in file or not.
- If the name not presented in file copy all contents into temporary file.
- If the name presented in file and assign the value of “found” will be “True”.
- Read next line from file using “readline()” method.
- Close the file “students.txt” with “fileObject”.
- Close the file “temp.txt” with “temp_file”.
- Remove “students.txt” using “os.remove()” method.
- Rename “temp.txt” as “students.txt” using “os.rename()” method.
- Using “if...else” condition display the intimation message on the screen.
- Call the “main()” method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
A temperature file consists of five records, each containing a temperature in degrees Fahrenheit. A program is to be written that will read the input temperature, convert it from degrees Fahrenheit to degrees Celsius and print both temperatures in two columns on a report. Column headings, which read ‘Degrees F’ and ‘Degrees C’, are to be printed at the top of the page. What ist he best solution algorithm to successfully implement the above question?
The names and student numbers of students are save in a text file called stnumbers.txt. Example of the content of the text file:
Peterson
20570856
Johnson
12345678
Suku
87654321
Westley
12345678
Venter
87654321
Mokoena
79012400
Makubela
29813360
Botha
30489059
Bradley
30350069
Manana
30530679
Shabalala
28863496
Smith
87873909
Nilsson
30989698
Makwela
30256607
Govender
30048117
Ntumba
30598303
Ramsamy
29952239
Skosana
29982995
Jameson
30228484
Xulu
29092248
Wasserman
27469352
Bester
28615425
Babane
27154033
Maboya
29897890
Mahlangu
30031338
Majavu
30165970
Myene
30954177
Motaung
30907276
Ramaroka
30804507
Radebe
30007674
Sekake
30017416
Zwane
30038227
Shuro
30238072
Viljoen
28881389
Sithole
45688555
Write a function called displayData() to receive the array and number of elements as parameters and display the names and student numbers of the students with a heading and neatly spaced.
Write a function, isValid(), which receives a number as parameter and determines whether the number…
The Apgar Medical group keeps a patient file for each doctor in the office. Each record contains the patient's first and last name, home address, and birth year. The records are sorted in ascending birth year order. Write a program so that display a count of the number of patients born each year
John Hanson, 23 Elm, 1927Mary Locust, 476 Maple, 1950Susan Monroe, 512 Peachtree, 1957Carol Fortune, 2819 Locust, 1960James Fortune, 2819 Locust, 1963Lawrence Fish, 12 Elm, 1968Janice Weiss, 234 Birch, 1971Henry Garza, 199 Second, 1973Kimberly Swanson, 310 Appletree, 1980Louis Claude, 2716 Third, 1981Jill Fox, 12 Oak, 1985Opal Reynolds, 78 County Line, 1987Francis Dumas, 67 Fourth, 1992Madison Conroy, 23 Fifth, 1996Daniel Moy, 100 Sunset, 1987
Chapter 10 Solutions
Starting Out with Programming Logic and Design (4th Edition)
Ch. 10.1 - Where are files normally stored?Ch. 10.1 - What is an output file?Ch. 10.1 - What is an input file?Ch. 10.1 - What three steps must be taken by a program when...Ch. 10.1 - Prob. 10.5CPCh. 10.1 - Prob. 10.6CPCh. 10.1 - When writing a program that performs an operation...Ch. 10.1 - In most programming languages, if a file already...Ch. 10.1 - What is the purpose of opening a file?Ch. 10.1 - What is the purpose of closing a file?
Ch. 10.1 - Prob. 10.11CPCh. 10.1 - Prob. 10.12CPCh. 10.1 - What is a files read position? Initially, where is...Ch. 10.1 - In what mode do you open a file if you want to...Ch. 10.2 - Prob. 10.15CPCh. 10.2 - What is the purpose of the eof function?Ch. 10.2 - Is it acceptable for a program to attempt to read...Ch. 10.2 - Prob. 10.18CPCh. 10.2 - Which of the following loops would you use to read...Ch. 10.4 - Prob. 10.20CPCh. 10.4 - Prob. 10.21CPCh. 10.4 - Prob. 10.22CPCh. 10 - A file that data is written to is known as a(n) a....Ch. 10 - A file that data is read from is known as a(n) a....Ch. 10 - Before a file can be used by a program, it must be...Ch. 10 - When a program is finished using a file, it should...Ch. 10 - The contents of this type of file can be viewed in...Ch. 10 - This type of file contains data that has not been...Ch. 10 - When working with this type of file, you access...Ch. 10 - When working with this type of file, you can jump...Ch. 10 - This is a small holding section in memory that...Ch. 10 - Prob. 10MCCh. 10 - This is a character or set of characters that...Ch. 10 - This marks the location of the next item that will...Ch. 10 - When a file is opened in this mode, data will be...Ch. 10 - The expression NOT eof (myFi1e) is equivalent to...Ch. 10 - This is a single piece of data within a record. a....Ch. 10 - When working with a sequential access file, you...Ch. 10 - In most languages, when you open an output file...Ch. 10 - The process of opening a file is only necessary...Ch. 10 - Prob. 4TFCh. 10 - Prob. 5TFCh. 10 - When a file that already exists is opened in...Ch. 10 - In control break logic, the program performs some...Ch. 10 - Describe the three steps that must be taken when a...Ch. 10 - Why should a program close a file when its...Ch. 10 - What is a files read position? Where is the read...Ch. 10 - If an existing file is opened in append mode, what...Ch. 10 - In most languages, if a file does not exist and a...Ch. 10 - What is the purpose of the eof function that was...Ch. 10 - What is control break logic?Ch. 10 - Design a program that opens an output file with...Ch. 10 - Design a program that opens the my_name.dat file...Ch. 10 - Prob. 3AWCh. 10 - Design an algorithm that does the following: opens...Ch. 10 - Modify the algorithm that you designed in question...Ch. 10 - Write pseudocode that opens an output file with...Ch. 10 - Prob. 7AWCh. 10 - A file exists on the disk named students.dat. The...Ch. 10 - Why doesn't the following pseudocode module work...Ch. 10 - File Display Assume that a file containing a...Ch. 10 - Item Counter Assume that a file containing a...Ch. 10 - Sum of Numbers Assume that a file containing a...Ch. 10 - Average of Numbers Assume that a file containing a...Ch. 10 - Largest Number Assume that a file containing a...Ch. 10 - Golf Scores The Springfork Amateur Golf Club has a...Ch. 10 - Sales Report Brewster's Used Cars, Inc. employs...
Knowledge Booster
Similar questions
- Finish this program from the code posted below! Note: There should be two files Main.py and Contact.py You will implement the edit_contact function. In the function, do the following: Ask the user to enter the name of the contact they want to edit. If the contact exists, in a loop, give them the following choices Remove one of the phone numbers from that Contact. Add a phone number to that Contact. Change that Contact's email address. Change that Contact's name (if they do this, you will have to remove the key/value pair from the dictionary and re-add it, since the key is the contact’s name. Use the dictionary's pop method for this!) Stop editing the Contact Once the user is finished making changes to the Contact, the function should return. Code:from Contact import Contactimport pickledef load_contacts():""" Unpickle the data on mydata.dat and save it to a dictionaryReturn an empty dictionary if the file doesn't exist """try:with open("mydata.dat", 'rb') as file:return…arrow_forwardCODE IN PYTHON PLEASE The objective is to create a code in Python that can extract the columns highlighted in blue (Column T and AB3) and output them to a .txt file. The code should be able to generate the text file with the columns that are highlighted in blue and store them on a separate folder. Below shows how the Test.xlsm file looks like as well as how the text file should look like when the code extracts and outputs it. Google drive to access Test.xlsm file: https://drive.google.com/drive/folders/16utzb5_h7yMCN8_13E_JqasfcpykZOYr?usp=sharing What my code outputs is shown in the picture (O1.png) What I would like for my code to output is the columns next to each other (Right Example.png) The current code is able to output Columns T and AB3 but I am not able to output them next to each other as shown above. We would also like for the code to be capable of storing the text file to a separate folder. Below is the code I have been working on. #package to read xlsm file import openpyxl…arrow_forwardA file has r = 20, 000 STUDENT records of fixed length. Each record has the following fields: NAME (30 bytes), SSN (9 bytes), ADRESS(40 bytes), PHONE(9 bytes), BIRTHDATE (8 bytes), SEX(1 byte), CLASSCODE( 4 bytes, integer) MAJORDEPTCODE(4 bytes), MINORDEPTCODE(4 bytes), and DEGREEPROGRAM( 3 bytes). An additional byte is used as a deletion marker. Block size B = 512 bytes. a) Calculate the blocking factor bfr (=floor(B/R), where R is the record size) and number of file blocks b, assuming unspanned organization (a record can’t be split across blocks). b) Suppose only 80% of the STUDENT records have a value for PHONE, 85% for MAJORDEPTCODE, 15% for MINORDEPTCODE, and 90% for DEGREEPROGRAM. We use a variable-length record file. Each record has a 1-byte field type for each field in the record, plus the 1-byte deletion marker and a 1-byte end-of-record marker. Suppose that we use a spanned record organization, where each block has a 5-byte pointer to the next block (this space is not used…arrow_forward
- Should not be case sensitive when getting the column name. Use python language for a program that modifies and sorts the content of a specific csv file based on the inputted column name. The program should ask Enter filename: Enter column name to be sorted: Additionally, the csv file must be modified and no additional csv files must be created. The application will catch and display an error message "the file does not exist" if the csv file does not exist.arrow_forwardYou are given a file called detention.txt with a list of students names and ID numbers. The data will be stored as shown below: Erin Shaw,36425109 Aldo Montes,8736542 Jessica,587963 Logan,22587 Leo Alvera,6544836 etc... Note that not all the students will have their last names in the detention.txt (names will not repeat in the file) Your job is to create a c++ program for teachers to look up a students name and display their student ID. Example output. (Input is highlighted) Welcome Staff! Please enter a student's name: Leo Alvera Searching for Leo Alvera... We found the Student ID: 6544836 for Leo Alvera Please enter a student's name: Jessica Alvera Searching for Jessica Alvera... Jessica Alvera not in detention.txt system Please enter a student's name: Please give proper explanation and typed answer only.arrow_forwardPython The program should open a specified text file, read its contents, then use the dictionary to writean encrypted version of the file’s contents to a second file. Each character in the second file should contain the code for the corresponding character in the first file. Your program contains both encryption AND decryption functions. Please do not put them in separate programs. Your program provides a MENU that offers the user a choice to encrypt or decrypt the file and call the respective function. Your encoder function will use the Caesar Ciphe to translate the file Your encoder function will first convert all input characters to upper case. This means that the output of encrypted text should be in CAPITALS. You must use a string method to convert! Your implementation must use a dictionary Your implementation must use string methods for converting casearrow_forward
- Binus’s Used Cars, Inc. employs several salespeople. Mr. Robert, the owner of the company, has provided a file that contains sales records for each salesperson for the past month. Each record in the file contains the following two fields: The salesperson’s ID number, as an integer The amount of a sale, as a real number The records are already sorted by salesperson ID. Brewster wants you to design a program that prints a sales report. The report should show each salesperson’s sales and the total sales for that salesperson. The report should also show the total sales for all salespeople for the month. Here is an example of how the sales report should appear:arrow_forwardEach of the following files in the Chapter.05 folder of your downloadable student files has syntax and/or logical errors. In each case, determine the problem, and fix the program. After you correct the errors, save each file using the same filename preceded with Fixed. For example, save DebugFive1.cs as FixedDebugFive1 .cs. a. DebugFive1.cs b. DebugFive2.cs c. DebugFive3.cs d. DebugFive4.csarrow_forwardEach of the following files in the Chapter.04 folder of your downloadable student files has syntax and/or logical errors. In each case, determine the problem, and fix the program. After you correct the errors, save each file using the same filename preceded with Fixed. For example, save DebugFour1.cs as FixedDebugFour1.cs. a. DebugFour1.cs b. DebugFour2.cs c. DebugFour3.cs d. DebugFour4.csarrow_forward
- Each of the following files in the Chapter.10 folder of your downloadable student files has syntax and/or logical errors. In each case, determine the problem and fix the program. After you correct the errors, save each file using the same filename preceded with Fixed. For example, DebugTen01.cs will become FixedDebugTen01 .cs. a. DebugTen01.cs b. DebugTen02.cs c. DebugTen03.cs d. DebugTen04.csarrow_forwardEach of the following files in the Chapter.06 folder of your downloadable student files has syntax and/or logical errors. In each case, determine the problem, and fix the program. After you correct the errors, save each file using the same filename preceded with Fixed. For example, DebugSix01.cs will become FixedDebugSix01.cs. a. DebugSix01.cs b. DebugSix02.cs c. DebugSix03.cs d. DebugSix04.csarrow_forwardEach of the following files in the Chapter.01 folder of your downloadable student files has syntax and/or logical errors. In each case, determine the problem and fix the program. After you correct the errors, save each file using the same filename preceded with Fixed. For example, DebugOne1.cs will become FixedDebugOne1 .cs. a. DebugOne1.cs b. DebugOne2.cs c. DebugOne3.cs d. DebugOne4.csarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
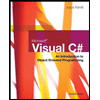
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
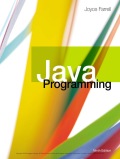
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
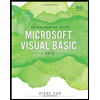
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
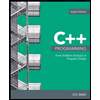
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning