Concept explainers
Explanation of Solution
a.
Th...
Explanation of Solution
b.
Th...
Explanation of Solution
c.
T...
Explanation of Solution
d.
Th...
Explanation of Solution
e.
The default constructor (without parameters) is used to initialize the object fruit1...
Explanation of Solution
f.
The code where each data member can be set individually is shown below. Set functions are used to accomplish the same and these are public functions:
void setName(string);
void setCalories(int);
void setFat(double);
void setSugar(int);
void setCarbohydrate(double);
void setPotassium(double);
The full class definition with the above methods in the public section is shown below:
class foodType
{
public:
void set(string, int, double, int, double, double);
void print() const;
string getName() const;
int getCalories() const;
double getFat() const;
int getSugar() const;
double getCarbohydrate () con...
Explanation of Solution
g.
The statement that would replace the definition of the constructors with a constructor with default parameter is as follows:
foodType(string = "banana", int = 90, double = 0...

Trending nowThis is a popular solution!

Chapter 10 Solutions
EP MINDTAPV2.0 FOR MALIK'S C++ PROGRAMM
- interface StudentsADT{void admissions();void discharge();void transfers(); }public class Course{String cname;int cno;int credits;public Course(){System.out.println("\nDEFAULT constructor called");}public Course(String c){System.out.println("\noverloaded constructor called");cname=c;}public Course(Course ch){System.out.println("\nCopy constructor called");cname=ch;}void setCourseName(String ch){cname=ch;System.out.println("\n"+cname);}void setSelectionNumber(int cno1){cno=cno1;System.out.println("\n"+cno);}void setNumberOfCredits(int cdit){credits=cdit;System.out.println("\n"+credits);}void setLink(){System.out.println("\nset link");}String getCourseName(){System.out.println("\n"+cname);}int getSelectionNumber(){System.out.println("\n"+cno);}int getNumberOfCredits(){System.out.println("\n"+credits); }void getLink(){System.out.println("\ninside get link");}} public class Students{String sname;int cno;int credits;int maxno;public Students(){System.out.println("\nDEFAULT constructor…arrow_forwardPublic classTestMain { public static void main(String [ ] args) { Car myCar1, myCar2; Electric Car myElec1, myElec2; myCar1 = new Car( ); myCar2 = new Car("Ford", 1200, "Green"); myElec1 = new ElectricCar( ); myElec2 = new ElectricCar(15); } }arrow_forward#pyhton programing topic: Introduction to Method and Designing class Method overloading & Constructor overloading ------------------ please find the attached imagearrow_forward
- Q:-Write a statement in ClassA to check out the accessibility of your variable. package package_02; public class ClassB { public int x = 1; int y = 2; private int z =3; } package package_01; import package_02.ClassB; public class ClassA { public static void main(String[] args) { ClassB cb = new ClassB(); // System.out.print(cb.x); /* 1 */ // System.out.print(cb.y); /* 2 */ // System.out.print(cb.z); /* 3 */ } }arrow_forward2arrow_forwardAPEX Test Class - Salesforce Please Desgin the Apex Test Class to solve the below problem public with sharing class MyFirstClass{ // Variable to store the values to perform the multiplication logic static Integer a = 5; static Integer b= 9; public static Integer productOfTwoNumbers; public static void multipicationOfTwoNumber() { //Product of the two Numbers productOfTwoNumbers = a * b; }arrow_forward
- Consider the following class definition: class circle { public: void print() const; void setRadius(double); void setCenter(double, double); void getCenter(double&, double&); double getRadius(); double area(); circle(); circle(double, double, double); double, double); private: double xCoordinate; double yCoordinate; double radius; } class cylinder: public circle { public: void print() const; void setHeight(double); double getHeight(); double volume(); double area(); cylinder(); cylinder(double, double, private: double height; } Suppose that you have the declaration: cylinder newCylinder; Write the definitions of the member functions of the classes circle and cylinder. Identify the member functions of the class cylinder that overrides the member functions of the class circlearrow_forwardGiven the following class definition: class employee{public: employee(); employee(string, int, double); employee(int, double); employee(string); void setData(string, int, double); void print() const; void updatePay(double); int getNumOfServiceYears() const; double getPay() const;private: string name; int numOfServiceYears; double pay;}; Create a class parttime derived from the above class that includes private members payRate and hoursWorked of type double along with a constructor and the definition of a print function that prints out all information from the base and derived class. Provide a test program that would demonstrate the working parttime class. Note: You can assume all code is stored in a single file.arrow_forwardConsider the following class definition: (8)class base{public:void setXYZ(int a, int b, int c);void setX(int a);int getX() const { return x; }void setY(int b);int getY() const { return y; }int mystryNum() { return (x * y - z * z); }void print() const;base() {}base(int a, int b, int c);protected:void setZ(int c) { z = c; }void secret();int z = 0;private:int x = 0;int y = 0;};a. Which member functions of the class base are protected?b. Which member functions of the class base are inline?c. Write the statements that derive the class myClass from classbase as a public inheritance.d. Determine which members of class base are private, protected,and public in class myClass.arrow_forward
- /* TestCarSensor.java - program to test the CarSensor class.*/public class TestCarSensor{public static void main (String[] args){CarSensor generic = new CarSensor();CarSensor tempCel = new CarSensor("temperature sensor", -50, +300, "C"); CarSensor speed = new CarSensor("speed sensor", 0, 200, "km/h"); CarSensor speed2 = new CarSensor("speed sensor 2", 0, 200, "m/h"); // 2. test changing desc and limitsSystem.out.println ();System.out.println ( generic ); // display generic sensor (zero)generic.setDesc ("special sensor"); // change descriptiongeneric.setLimits (-5,5,"units"); // change limitsSystem.out.println ( generic ); // display generic sensor again// 3. test displaying object (calling .toString() )System.out.println ();System.out.println ( tempCel );System.out.println ( speed );System.out.println ( speed2 );// 4. test setlimits() ruleSystem.out.println ();System.out.println ( generic ); generic.setLimits (10, -10, "blah"); System.out.println ( generic ); generic.setLimits (-10,…arrow_forwardclass Player { protected: string name; double weight; double height; public: Player(string n, double w, double h) { name = n; weight = w; height = h; } string getName() const { return name; } virtual void printStats() const = 0; }; class BasketballPlayer : public Player { private: int fieldgoals; int attempts; public: BasketballPlayer(string n, double w, double h, int fg, int a) : Player(n, w, h) { fieldgoals = fg; attempts = a; } void printStats() const { cout << name << endl; cout << "Weight: " << weight; cout << " Height: " << height << endl; cout << "FG: " << fieldgoals; cout << " attempts: " << attempts; cout << " Pct: " << (double) fieldgoals / attempts << endl; } }; a. What does = 0 after function printStats() do? b. Would the following line in main() compile: Player p; -- why or why not? c. Could…arrow_forwardclass Vehicle{String s = "This is my vehicle" ;}class Car extends Vehicle{String s = "This is my car" ;{System.out.println( super .s);}} class Truck extends Car{String s = "This is my truck" ;{System.out.println( super .s);}}public class MainClass{public static void main(String[] args){Truck t = new Truck ();System.out.println(t.s);}}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
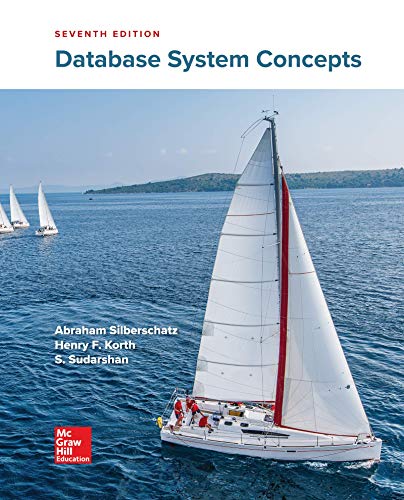
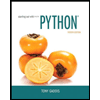
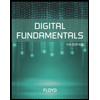
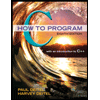
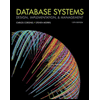
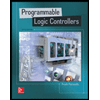