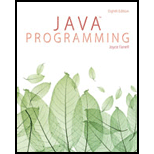
JAVA PROGRAMMING-LMS INTEG.MINDTAP
8th Edition
ISBN: 9781337091503
Author: FARRELL
Publisher: Cengage Learning
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 10, Problem 6PE
Program Plan Intro
Base ball game
Program plan:
Filename: “DemoBaseballGame.java”
- Include the required header files
- Define the class “DemoBaseballGame”
- Define the main method
- Create an object for “BaseballGame” class
- Call the method “SetNames” for setting the names
- Call the method “display”
- Call the “setScore” method for setting the score
- After setting the score, call the “display” method
- Call the “setScore” method in fifth inning
- Call the “setScore” method after setting second inning
- Call the “setScore” method for invalid inning
- Call the “setScore” method for invalid team
- Iterate the “t” value until it reaches “2”
- Iterate the “i” value until it reaches “getInnings”
- Call the “setScore” method
- Iterate the “i” value until it reaches “getInnings”
- Call the “display” method
- Define the “display” method
- Declare the required variables
- Display the teams
- Iterate the “t” value until it reaches “2”
- Iterate the “i” value until it reaches “getInnings”
- Call the “getScore” method
- Check “score” is not equal to “BaseballGame.UNPLAYED”
-
- Check the “i” is equal to 0
- Display the team
- Display the score
- Calculate the total score
-
- Set the value
-
- Check the “i” is equal to 0
- Iterate the “i” value until it reaches “getInnings”
- Check the condition
- Display no innings played yet
- Check the condition
- Check “total[0]” is greater than “total[1]”
- Display the winning name
- Otherwise, check “total [0]” is less than “total [1]”
- Display the winning name
- Otherwise, display game is tie.
- Check “total[0]” is greater than “total[1]”
- Define the main method
Filename: “BaseballGame.java”
- Define the “BaseballGame” class
- Declare the required variables
- Define the constructor
- Declare an array variable
- Iterate “t” until it reaches “scores” length
- Iterate “i” until it reaches “scores [t]” length
- Set the value
- Iterate “i” until it reaches “scores [t]” length
- Define the “setNames” method
- Set the values
- Define the “setScore” method
- Declare the required variables
- Check “team” is less than 0 or greater than “scores” length minus 1
- Display the team is valid
- Check “inning” is less than 0 or greater than “scores” length minus 1
- Display the team is not valid
- Otherwise,
- Iterate “x” until it reaches “inning”
- Check the condition
-
- Set the value
- Check the condition
- Display a score can’t yet be set for inning
- Otherwise, set the value
- Iterate “x” until it reaches “inning”
- Define the “setNames” method
- Return the names
- Define the “getNames” method
- Return the position of the name
- Define the “getScores” method
- Declare the variable
- Check “team” is less than 0 or greater than “scores” length minus 1
- Display invalid team number
- Check “inning” is less than 0 or greater than “scores[0]” length minus 1
- Display invalid inning
- Otherwise, set the value
- Define “getInnings” method
- Return innings
Filename: “HighSchoolBaseballGame.java”
- Declare the variable and assign the value
- Define the constructor
- Declare the array variable
- Iterate “t” until it reaches “scores” length
- Iterate “i” until it reaches “scores[t]” length
- Set the value
- Iterate “i” until it reaches “scores[t]” length
- Define “getInnings” method
- Return innings
Filename: “LittleLeagueBaseballGame.java”
- Declare the variable and assign the value
- Define the constructor
- Declare the array variable
- Iterate “t” until it reaches “scores” length
- Iterate “i” until it reaches “scores[t]” length
- Set the value
- Iterate “i” until it reaches “scores[t]” length
- Define “getInnings” method
- Return innings
Filename: “DemoLLBaseballGame.java”
- Include the required header files
- Define the class “DemoLLBaseballGame”
- Define the main method
- Create an object for “LittleLeagueBaseballGame” class
- Call the method “SetNames” for setting the names
- Call the method “display”
- Call the “setScore” method for setting the score
- After setting the score, call the “display” method
- Call the “setScore” method in fifth inning
- Call the “setScore” method after setting second inning
- Call the “setScore” method for invalid inning
- Call the “setScore” method for invalid team
- Iterate the “t” value until it reaches “2”
- Iterate the “i” value until it reaches “getInnings”
- Call the “setScore” method
- Iterate the “i” value until it reaches “getInnings”
- Call the “display” method
- Define the “display” method
- Declare the required variables
- Display the teams
- Iterate the “t” value until it reaches “2”
- Iterate the “i” value until it reaches “getInnings”
- Call the “getScore” method
- Check “score” is not equal to “BaseballGame.UNPLAYED”
-
- Check the “i” is equal to 0
- Display the team
- Display the score
- Calculate the total score
-
- Otherwise, set the value
-
- Check the “i” is equal to 0
- Iterate the “i” value until it reaches “getInnings”
- Check the condition
- Display no innings played yet
- Check the condition
- Check “total[0]” is greater than “total[1]”
- Display the team 1 is win
- Otherwise, check “total [0]” is less than “total [1]”
- Display the team 2 is win
- Otherwise, display game is tie.
- Check “total[0]” is greater than “total[1]”
- Define the main method
Filename: “DemoHSBaseballGame.java”
- Include the required header files
- Define the class “DemoHSBaseballGame”
- Define the main method
- Create an object for “HighSchoolBaseballGame” class
- Call the method “SetNames” for setting the names
- Call the method “display”
- Call the “setScore” method for setting the score
- After setting the score, call the “display” method
- Call the “setScore” method in fifth inning
- Call the “setScore” method after setting second inning
- Call the “setScore” method for invalid inning
- Call the “setScore” method for invalid team
- Iterate the “t” value until it reaches “2”
- Iterate the “i” value until it reaches “getInnings”
- Call the “setScore” method
- Iterate the “i” value until it reaches “getInnings”
- Call the “display” method
- Define the “display” method
- Declare the required variables
- Display the teams
- Iterate the “t” value until it reaches “2”
- Iterate the “i” value until it reaches “getInnings”
- Call the “getScore” method
- Check “score” is not equal to “BaseballGame.UNPLAYED”
-
- Check the “i” is equal to 0
- Display the team
- Display the score
- Calculate the total score
-
- Otherwise, set the value
-
- Check the “i” is equal to 0
- Iterate the “i” value until it reaches “getInnings”
- Check the condition
- Display no innings played yet
- Check the condition
- Check “total[0]” is greater than “total[1]”
- Display the team 1 is win
- Otherwise, check “total [0]” is less than “total [1]”
- Display the team 2 is win
- Otherwise, display game is tie.
- Check “total[0]” is greater than “total[1]”
- Define the main method
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
D. S. Malik, Data Structures Using C++, 2nd Edition, 2010
Methods (Ch6) - Review
1. (The MyRoot method) Below is a manual implementation of the Math.sqrt() method in Java.
There are two methods, method #1 which calculates the square root for positive integers, and
method #2, which calculates the square root of positive doubles (also works for integers).
public class SquareRoot {
public static void main(String[] args) {
}
// implement a loop of your choice here
// Method that calculates the square root of integer variables
public static double myRoot(int number) {
double root;
root=number/2;
double root old;
do {
root old root;
root (root_old+number/root_old)/2;
} while (Math.abs(root_old-root)>1.8E-6);
return root;
}
// Method that calculates the square root of double variables
public static double myRoot(double number) {
double root;
root number/2;
double root_old;
do {
root old root;
root (root_old+number/root_old)/2;
while (Math.abs (root_old-root)>1.0E-6);
return root;
}
}
Program-it-Yourself: In the main method, create a program that…
I would like to know the main features about the following 3 key concepts:1. Backup Domain Controller (BDC)2. Access Control List (ACL)3. Dynamic Memory
Chapter 10 Solutions
JAVA PROGRAMMING-LMS INTEG.MINDTAP
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In cell C21, enter a formula to calculate the number of miles you expect to drive each month. Divide the value of number of miles (cell A5 from the Data sheet) by the average MPG for the vehicle multiplied by the price of a gallon of gas (cell A6 from the Data sheet).arrow_forwardMicrosoft Excelarrow_forwardIn cell C16, enter a formula to calculate the price of the vehicle minus your available cash (from cell A3 in the Data worksheet). Use absolute references where appropriate—you will be copying this formula across the row what fomula would i use and how do i solve itarrow_forward
- What types of data visualizations or tools based on data visualizations have you used professionally, whether in a current or past position? What types of data did they involve? What, in your experience, is the value these data views or tools added to your performance or productivity?arrow_forwardQuestion: Finding the smallest element and its row index and column index in 2D Array: 1. Write a public Java class min2D. 2. In min2D, write a main method. 3. In the main method, create a 2-D array myArray with 2 rows and 5 columns: {{10, 21, 20, 13, 1}, {2, 6, 7, 8, 14}}. 4. Then, use a nested for loop to find the smallest element and its row index and column index. 5. Print the smallest element and its row index and column index on Java Consolearrow_forward(using R)The iris data set in R gives the measurements in centimeters of the variables sepal length and width andpetal length and width, respectively, for 50 flowers from each of 3 species of iris, setosa, versicolor, andvirginica. Use the iris data set and the t.test function, test if the mean of pepal length of iris flowers isgreater than the mean of sepal length.The iris data set in R gives the measurements in centimeters of the variables sepal length and width andpetal length and width, respectively, for 50 flowers from each of 3 species of iris, setosa, versicolor, andvirginica. Use the iris data set and the t.test function, test if the mean of pepal length of iris flowers isgreater than the mean of sepal length.arrow_forward
- Recognizing the Use of Steganography in Forensic Evidence (4e)Digital Forensics, Investigation, and Response, Fourth Edition - Lab 02arrow_forwardWrite a Java Program to manage student information of a university. The Javaprogram does the following steps:a) The program must use single-dimensional arrays to store the studentinformation such as Student ID, Name and Major.b) The program asks the user to provide the number of students.c) The program asks the user to enter the Student IDs for the number of studentsand stores them.d) The program asks the user to enter the corresponding names for the numberof students and stores them.e) The program then asks the user to provide the corresponding major for thestudents and stores them.f) The program then should display the following options:1. ID Search2. Major Enrollment3. Exitg) On selecting option 1, the user can search for a student using Student ID. Theprogram asks the user to enter a Student ID. It then should print thecorresponding student’s details such as Name and Major if the user providedStudent ID number is present in the stored data. If the user’s Student IDnumber does not…arrow_forward(a) Algebraically determine the output state |q3q2q1q0> (which is a 4-qubitvector in 16-dimensional Hilbert space). Show all steps of your calculations. (b) Run a Qiskit code which implements the circuit and append threemeasurement gates to measure the (partial) output state |q2q1q0> (which is a 3-qubit vector in 8-dimensional Hilbert space). this is for quantum soft dev class, you can use stuff like Deutsch Jozsa if u wantarrow_forward
- Write a C++ program that will count from 1 to 10 by 1. The default output should be 1, 2, 3, 4, 5, 6 , 7, 8, 9, 10 There should be only a newline after the last number. Each number except the last should be followed by a comma and a space. To make your program more functional, you should parse command line arguments and change behavior based on their values. Argument Parameter Action -f, --first yes, an integer Change place you start counting -l, --last yes, an integer Change place you end counting -s, --skip optional, an integer, 1 if not specified Change the amount you add to the counter each iteration -h, --help none Print a help message including these instructions. -j, --joke none Tell a number based joke. So, if your program is called counter counter -f 10 --last 4 --skip 2 should produce 10, 8, 6, 4 Please use the last supplied argument. If your code is called counter, counter -f 4 -f 5 -f 6 should count from 6. You should count from first to last inclusively.…arrow_forwardWrite a program that will count from 1 to 10 by 1. The default output should be 1, 2, 3, 4, 5, 6 , 7, 8, 9, 10 There should be only a newline after the last number. Each number except the last should be followed by a comma and a space. To make your program more functional, you should parse command line arguments and change behavior based on their values. Argument Parameter Action -f, --first yes, an integer Change place you start counting -l, --last yes, an integer Change place you end counting -s, --skip optional, an integer, 1 if not specified Change the amount you add to the counter each iteration -h, --help none Print a help message including these instructions. -j, --joke none Tell a number based joke. So, if your program is called counter counter -f 10 --last 4 --skip 2 should produce 10, 8, 6, 4 Please use the last supplied argument. If your code is called counter, counter -f 4 -f 5 -f 6 should count from 6. You should count from first to last inclusively. You…arrow_forwardWas What is the deference betwem full At Adber and Hold?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
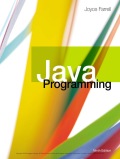
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
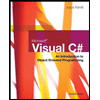
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
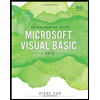
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
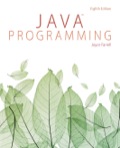
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY