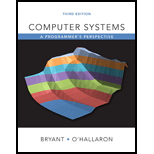
Concept explainers
Opening and Closing files:
Using “Open” function, a process can open an existing file or generates a new file.
- This function is used to converts a filename to a file descriptor and returns the result as a descriptor number.
- The descriptor returned is always the lowest descriptor that is not presently open in the process.
- Each process in the LINUX begins life with three open files.
- Descriptor 0 – standard input.
- Descriptor 1 – standard output.
- Descriptor 2 – standard error.
- The “Open” function consists of three arguments. That is “Open(filename, flags, mode)”.
- The argument “filename” defines the name of the given file
- The argument “flags” represents how the process plans to access the file. Some flags names are as follows.
- O_RDONLY – it means reading only.
- O_WRONLY – it means writing only.
- O_RDWR – it means reading and writing.
- The “mode” argument identifies the access permission bits of new files.
Example:
The example for open an existing file for reading is shown below:
sample1 = Open("foo1.txt", O_RDONLY, 0);
From the above “open” function,
- The filename is “foo1.txt”.
- Flag name is “O_RDONLY”.
- Mode is “0”.

Explanation of Solution
Corresponding code from given question:
Main.c:
//Header file
#include "csapp.h"
//Main function
int main()
{
//Declare int variable
int fd1, fd2;
/* Open the file "foo.txt" using "Open" function and store descriptor number in fd1 */
fd1 = Open("foo.txt",O_RDONLY, 0);
/* Open the file "bar.txt" using "open" function and store descriptor number in fd2 */
fd2 = Open("bar.txt",O_RDONLY, 0);
//Frees up the descriptor number in "fd2"
Close(fd2);
/* Open the file "baz.txt" using "Open" function and store descriptor number in fd2 */
fd2 = Open("baz.txt",O_RDONLY, 0);
//Display the descriptor number in "fd2"
printf("fd2 = %d\n", fd2);
//Exit the process
exit(0);
}
Explanation:
The given code is used to returns the descriptor number for given file.
- Include the header file
- Define the main function.
- Declare two variables “fd1” and “fd2” in “int” data type.
- Open the file “foo.txt” using “Open” function and store its descriptor number in “fd1”.
- Open the file “bar.txt” using “Open” function and store its descriptor number in “fd2”.
- Frees up the descriptor number in “fd2” using “Close” function.
- Open the file “baz.txt” using “Open” function and store its descriptor number in “fd2”.
- Then displays the descriptor number in “fd2”.
- Finally exit the process using “exit” function.
- Before run the program, user needs to create the three files “foo.txt”, “bar.txt” and “baz.txt”.
Reasons for displaying given output:
- The “Open” function always returned the smallest unopened descriptor.
- From the given code, first call to “Open” returns the descriptor 3 that is the descriptor 3 is in “fd1”.
- Then call to “Open” returns the descriptor 4 that is the descriptor 4 is in “fd2”.
- After that “fd2” frees up using “Close” function.
- Now, call to “Open” function returns the descriptor 4 in “fd2”. Therefore, the output of the given code is “fd2 = 4”.
fd2 = 4
Want to see more full solutions like this?
Chapter 10 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
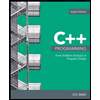