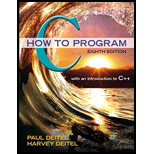
Concept explainers
(a)
Create a Structure inventory which contains the following:
- A character array partName[30]
- An integer partNumber,
- A floating point price
- An integer stock
- An integer reorder.
(a)

Explanation of Solution
Explanation:
A structure is a user defined data type which contains related variables which have same name.
Following syntax is used to create a structure with a keyword struct:
struct <structure name> { variable 1; variable 2; ---; };
Structure named Inventory with the given variables is defined as follows:
struct Inventory { char partName[30]; int partNumber; float pointprice; int stock; int reorder; };
(b)
Define the union data containing char c, short s, long b, float f and double d.
(b)

Explanation of Solution
Explanation:
Union is a user defined data type which contains related variables just like structure which uses same data space for its variable.
Following syntax is used to create an Union data type:
union <union name> { variable 1; variable 2; ---; };
Union named data which contains char c, short s, long b, float f and double d is defined as follows:
union data { char c; short s; long b; float f; double d; };
(c)
Create a structure called address that contains character arrays
- streetAddress[ 25]
- city[ 20 ]
- state[ 3]
- zipCode[ 6].
(c)

Explanation of Solution
Explanation:
A structure is a derived defined data type which contains related variables which have same name.
Following syntax is used to create a structure with a keyword struct:
struct <structure name> { variable 1; variable 2; ---; };
Structure with the name address containing character arrays is defined as follows:
struct address { char streetAddress[25]; char city[20]; char state[3]; char zipCode[6]; };
(d)
Create a structure student that contains the following :
- An character array firstName[15]
- An character array lastName[15]
- variable homeAddress of type struct address.
(d)

Explanation of Solution
Given Information
Structure named address as follows:
struct address { char streetAddress[25]; char city[20]; char state[3]; char zipCode[6]; };
Explanation:
A structure is a derived defined data type which contains related variables which have same name but each variable in the structure has its own data space.
Keyword struct is used to create a structure as follows:
struct <structure name> { variable 1; variable 2; ---; };
Structures can be used to declare variable which can access variables of the structure with the use of following syntax:
struct <structure_name><variable_name>;
Structure name Student which contains a structure
A structure student that contains the variable homeAddress of type struct address along with character arrays firstName and lastName is defined as follows:
struct student { char firstName[15]; char lastName[15]; struct address homeAddress; };
(e)
Create a structure test which contains a 16 bit fields with widths of 1 bit. The names of the bit fields are the letters a to p.
(e)

Explanation of Solution
Explanation:
Bit fields can be defined in the structure which enables user to specify the number of bits a unsigned or int member of variable can store.
Bit fields are defined with the following syntax:
<data type> <bit-field name> <number of bits>
A structure test which contains a 16 bit fields with widths of 1 bit is defined as follows:
struct test { unsigned int a : 1; unsigned int b : 1; unsigned int c : 1; unsigned int d : 1; unsigned int e : 1; unsigned int f : 1; unsigned int g : 1; unsigned int h : 1; unsigned int i : 1; unsigned int j : 1; unsigned int k : 1; unsigned int l : 1; unsigned int m : 1; unsigned int n : 1; unsigned int o : 1; unsigned int p : 1; };
Want to see more full solutions like this?
Chapter 10 Solutions
C How To Program Plus Mylab Programming With Pearson Etext -- Access Card Package (8th Edition)
- whats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forwardWhat is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.arrow_forward
- Consider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forwardassume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forward
- Consider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forwardNo aiarrow_forwardGiven the dependency diagram of attributes {C1,C2,C3,C4,C5) in a table shown in the following figure, (the primary key attributes are underlined)arrow_forward
- What are 3 design techniques that enable data representations to be effective and engaging? What are some usability considerations when designing data representations? Provide examples or use cases from your professional experience.arrow_forward2D array, Passing Arrays to Methods, Returning an Array from a Method (Ch8) 2. Read-And-Analyze: Given the code below, answer the following questions. 2 1 import java.util.Scanner; 3 public class Array2DPractice { 4 5 6 7 8 9 10 11 12 13 14 15 16 public static void main(String args[]) { 17 } 18 // Get an array from the user int[][] m = getArray(); // Display array elements System.out.println("You provided the following array "+ java.util.Arrays.deepToString(m)); // Display array characteristics int[] r = findCharacteristics(m); System.out.println("The minimum value is: " + r[0]); System.out.println("The maximum value is: " + r[1]); System.out.println("The average is: " + r[2] * 1.0/(m.length * m[0].length)); 19 // Create an array from user input public static int[][] getArray() { 20 21 PASSTR2222322222222222 222323 F F F F 44 // Create a Scanner to read user input Scanner input = new Scanner(System.in); // Ask user to input a number, and grab that number with the Scanner…arrow_forwardGiven the dependency diagram of attributes C1,C2,C3,C4,C5 in a table shown in the following figure, the primary key attributes are underlined Make a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NFarrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
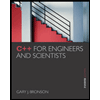
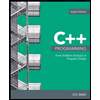
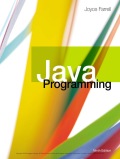
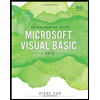
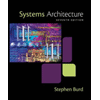