Your tasks Implement the functions marked with ??? in withProb.scala. (as shown in below) package withProb: /** * NOTE: The seed should be System.nanoTime or something similar * in order to get real pseudo-randomness but we fix it now * for the sake of repeatability. */ val rand = new scala.util.Random(2105) /** * Executes the given code with probability "prob". * For instance, * doWithProb(30.0) { Console.println("I'm feeling lucky!") } * shall print the text to the console with probability of 30 percent. * * Note that you have to modify the type of the "thenAction" parameter so that it admits code * to be passed in "call-by-name" evaluation mode. * Please see the course material and * http://docs.scala-lang.org/tour/automatic-closures.html */ def doWithProb(prob: Double)(thenAction: Unit): Unit = require(0.0 <= prob && prob <= 100.0) ??? end doWithProb /** * The expression * withProb(prob) {choice1} otherwise {choice2} * returns the value of the first choice code with probability prob and * the value of the second choice with probability 1-prob. * * For instance, * val x = 2 * val y = 3 * val z: Int = withProb(30.0) {x*y} otherwise {0} * shall return 6 with 30% probability and 0 with 70% probability. * * Observe that we can also write a statement like * withProb(70.0) { println("first action") } otherwise { println("second action") } * The return value is just ignored. * * Note that you have to modify the types of the choice1 and choice2 parameters so that they admit code * with correct return type to be passed in "call-by-name" evaluation mode. * Again, please see the course material and especially * http://docs.scala-lang.org/tour/automatic-closures.html * for help. */ def withProb[T](prob: Double)(choice1: Any): withProbElse[T] = require(0.0 <= prob && prob <= 100.0) ??? end withProb protected class withProbElse[T](prob: Double, choice1: Any): def otherwise(choice2: Any): T = ??? end otherwise
Your tasks Implement the functions marked with ??? in withProb.scala. (as shown in below) package withProb: /** * NOTE: The seed should be System.nanoTime or something similar * in order to get real pseudo-randomness but we fix it now * for the sake of repeatability. */ val rand = new scala.util.Random(2105) /** * Executes the given code with probability "prob". * For instance, * doWithProb(30.0) { Console.println("I'm feeling lucky!") } * shall print the text to the console with probability of 30 percent. * * Note that you have to modify the type of the "thenAction" parameter so that it admits code * to be passed in "call-by-name" evaluation mode. * Please see the course material and * http://docs.scala-lang.org/tour/automatic-closures.html */ def doWithProb(prob: Double)(thenAction: Unit): Unit = require(0.0 <= prob && prob <= 100.0) ??? end doWithProb /** * The expression * withProb(prob) {choice1} otherwise {choice2} * returns the value of the first choice code with probability prob and * the value of the second choice with probability 1-prob. * * For instance, * val x = 2 * val y = 3 * val z: Int = withProb(30.0) {x*y} otherwise {0} * shall return 6 with 30% probability and 0 with 70% probability. * * Observe that we can also write a statement like * withProb(70.0) { println("first action") } otherwise { println("second action") } * The return value is just ignored. * * Note that you have to modify the types of the choice1 and choice2 parameters so that they admit code * with correct return type to be passed in "call-by-name" evaluation mode. * Again, please see the course material and especially * http://docs.scala-lang.org/tour/automatic-closures.html * for help. */ def withProb[T](prob: Double)(choice1: Any): withProbElse[T] = require(0.0 <= prob && prob <= 100.0) ??? end withProb protected class withProbElse[T](prob: Double, choice1: Any): def otherwise(choice2: Any): T = ??? end otherwise
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Your tasks
- Implement the functions marked with ??? in withProb.scala. (as shown in below)
package withProb:
/**
* NOTE: The seed should be System.nanoTime or something similar
* in order to get real pseudo-randomness but we fix it now
* for the sake of repeatability.
*/
val rand = new scala.util.Random(2105)
/**
* Executes the given code with probability "prob".
* For instance,
* doWithProb(30.0) { Console.println("I'm feeling lucky!") }
* shall print the text to the console with probability of 30 percent.
*
* Note that you have to modify the type of the "thenAction" parameter so that it admits code
* to be passed in "call-by-name" evaluation mode.
* Please see the course material and
* http://docs.scala-lang.org/tour/automatic-closures.html
*/
def doWithProb(prob: Double)(thenAction: Unit): Unit =
require(0.0 <= prob && prob <= 100.0)
???
end doWithProb
/**
* The expression
* withProb(prob) {choice1} otherwise {choice2}
* returns the value of the first choice code with probability prob and
* the value of the second choice with probability 1-prob.
*
* For instance,
* val x = 2
* val y = 3
* val z: Int = withProb(30.0) {x*y} otherwise {0}
* shall return 6 with 30% probability and 0 with 70% probability.
*
* Observe that we can also write a statement like
* withProb(70.0) { println("first action") } otherwise { println("second action") }
* The return value is just ignored.
*
* Note that you have to modify the types of the choice1 and choice2 parameters so that they admit code
* with correct return type to be passed in "call-by-name" evaluation mode.
* Again, please see the course material and especially
* http://docs.scala-lang.org/tour/automatic-closures.html
* for help.
*/
def withProb[T](prob: Double)(choice1: Any): withProbElse[T] =
require(0.0 <= prob && prob <= 100.0)
???
end withProb
protected class withProbElse[T](prob: Double, choice1: Any):
def otherwise(choice2: Any): T =
???
end otherwise
end withProbElse
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
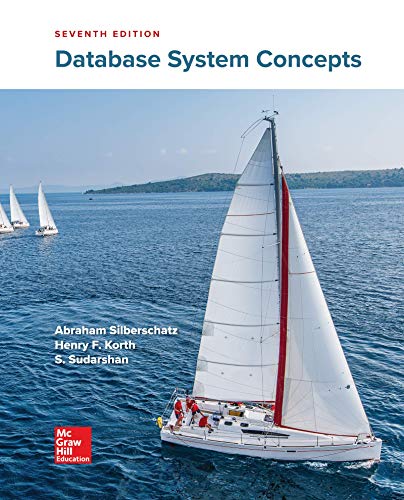
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
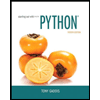
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
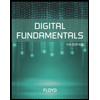
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
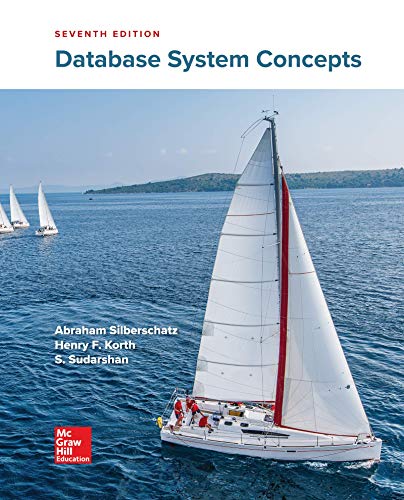
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
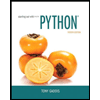
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
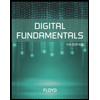
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
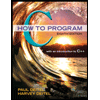
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
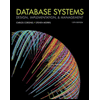
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
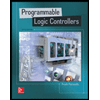
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education