Your program must define and call the following method. The method should return true if the input year is a leap year and false otherwise. public static boolean isLeapYear(int userYear)
Your program must define and call the following method. The method should return true if the input year is a leap year and false otherwise. public static boolean isLeapYear(int userYear)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
The language is Java. The method to use is listed in the picture.

Transcribed Image Text:### Understanding Leap Years
A common year in the modern Gregorian calendar consists of 365 days. However, the Earth takes longer to rotate around the sun than this, so a leap year is added every four years to account for the difference. A leap year contains 366 days, with an extra day added on February 29th. The requirements for a year to be a leap year are:
1. The year must be divisible by 4.
2. If the year is a century year (e.g., 1700, 1800), it must be divisible by 400.
Examples of leap years include 1600, 1712, and 2016.
### Programming Task
Write a program that receives a year as input and determines if it is a leap year.
#### Example Input and Output
- **Input**: `1712`
**Output**: `1712 is a leap year.`
- **Input**: `1913`
**Output**: `1913 is not a leap year.`
### Method Definition
Your program should include and call the following method. The method should return `true` if the input year is a leap year, and `false` otherwise.
```java
public static boolean isLeapYear(int userYear)
```
**Note**: This exercise builds upon a previous chapter and now requires the use of a method to determine leap years.
![```java
import java.util.Scanner;
public class LabProgram {
/* Define your method here */
public static void main(String[] args) {
/* Type your code here. */
}
}
```
### Description
This basic Java program template is designed for educational purposes. It includes:
- **Import Statement:** `import java.util.Scanner;` allows the program to use the Scanner class, which is part of the `java.util` package. This class is commonly used to obtain user input.
- **Class Declaration:** `public class LabProgram` defines a public class named `LabProgram`. This is where the program's logic will reside.
- **Method Definition Placeholder:** `/* Define your method here */` is a comment indicating where to define additional methods within the class. Users can add their own methods above the `main` method.
- **Main Method:**
- `public static void main(String[] args) { ... }` is the entry point of any Java application.
- The comment `/* Type your code here. */` is a placeholder, guiding users to insert their code inside the main method.
### Usage
Students can use this template as a starting point for programming assignments or practice exercises. They are encouraged to explore Java functionalities by defining methods and implementing logic inside the provided structure.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8fe79bd2-2df3-45cc-abc0-ffa837cca5d8%2Fd5725641-7749-4ecc-b6d3-7077cfc42ff1%2Fycl40ps_processed.png&w=3840&q=75)
Transcribed Image Text:```java
import java.util.Scanner;
public class LabProgram {
/* Define your method here */
public static void main(String[] args) {
/* Type your code here. */
}
}
```
### Description
This basic Java program template is designed for educational purposes. It includes:
- **Import Statement:** `import java.util.Scanner;` allows the program to use the Scanner class, which is part of the `java.util` package. This class is commonly used to obtain user input.
- **Class Declaration:** `public class LabProgram` defines a public class named `LabProgram`. This is where the program's logic will reside.
- **Method Definition Placeholder:** `/* Define your method here */` is a comment indicating where to define additional methods within the class. Users can add their own methods above the `main` method.
- **Main Method:**
- `public static void main(String[] args) { ... }` is the entry point of any Java application.
- The comment `/* Type your code here. */` is a placeholder, guiding users to insert their code inside the main method.
### Usage
Students can use this template as a starting point for programming assignments or practice exercises. They are encouraged to explore Java functionalities by defining methods and implementing logic inside the provided structure.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
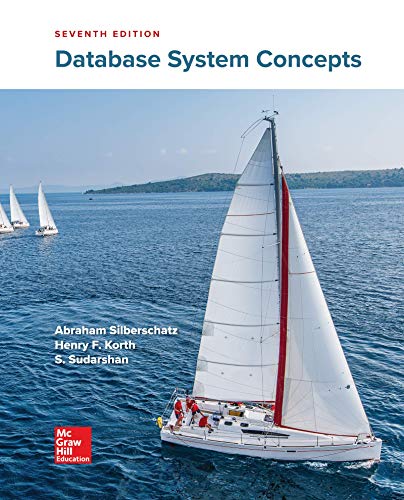
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
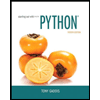
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
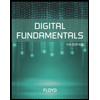
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
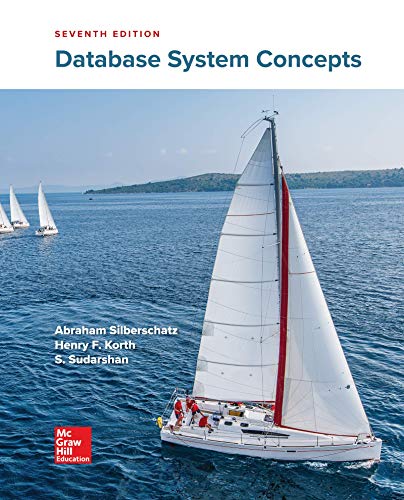
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
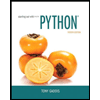
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
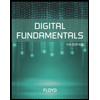
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
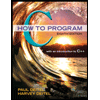
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
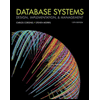
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
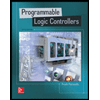
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education