Here are my instructions for my C++ program: Write a program that uses the function isPalindrome given in Example 6-6 (Palindrome). Test your program on the following strings: madam, abba, 22, 67876, 444244, trymeuemyrt Modify the function isPalindrome of Example 6-6 so that when determining whether a string is a palindrome, cases are ignored, that is, uppercase and lowercase letters are considered the same. The isPalindrome function from Example 6-6 has been included below for your convenience. bool isPalindrome(string str) { int length = str.length(); for (int i = 0; i < length / 2; i++) { if (str[i] != str[length – 1 – i]) { return false; } // if } // for loop return true; }// isPalindrome Your program should print a message indicating if a string is a palindrome: madam is a palindrome The lab values require that I have the following string patterns in my lab: .+*isPalindrome\(\"Madam\"\).+* .+*isPalindrome\(\"abBa\"\).+* .+*isPalindrome\(\"22\"\).+* .+*isPalindrome\(\"67876\"\).+* .+*isPalindrome\(\"444244\"\).+* .+*isPalindrome\(\"trYmeuemyRT\"\).+*
Here are my instructions for my C++ program:
Write a program that uses the
function isPalindrome given in
Example 6-6 (Palindrome). Test
your program on the following
strings:
madam, abba, 22, 67876, 444244, trymeuemyrt
Modify the function isPalindrome
of Example 6-6 so that when
determining whether a string is a
palindrome, cases are ignored, that
is, uppercase and lowercase letters
are considered the same.
The isPalindrome function from
Example 6-6 has been included
below for your convenience.
bool isPalindrome(string str)
{
int length = str.length();
for (int i = 0; i < length / 2; i++) {
if (str[i] != str[length – 1 – i]) {
return false;
} // if
} // for loop
return true;
}// isPalindrome
Your program should print a
message indicating if a string is a
palindrome:
madam is a palindrome
The lab values require that I have the following string patterns in my lab:
.+*isPalindrome\(\"Madam\"\).+*
.+*isPalindrome\(\"abBa\"\).+*
.+*isPalindrome\(\"22\"\).+*
.+*isPalindrome\(\"67876\"\).+*
.+*isPalindrome\(\"444244\"\).+*
.+*isPalindrome\(\"trYmeuemyRT\"\).+*



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Your output was correct
The lab values require that I have the following string patterns in my lab:
Description
Searched your code for a specific pattern:
.+*isPalindrome\(\"Madam\"\).+*
.+*isPalindrome\(\"Madam\"\).+*
.+*isPalindrome\(\"abBa\"\).+*
.+*isPalindrome\(\"22\"\).+*
.+*isPalindrome\(\"67876\"\).+*
.+*isPalindrome\(\"444244\"\).+*
.+*isPalindrome\(\"trYmeuemyRT\"\).+*
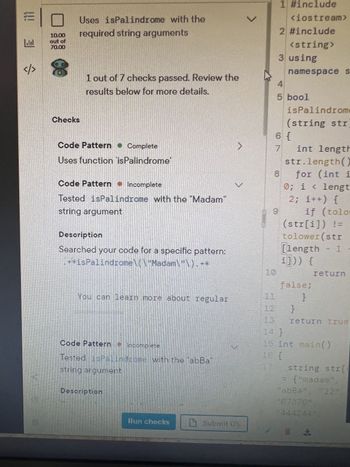
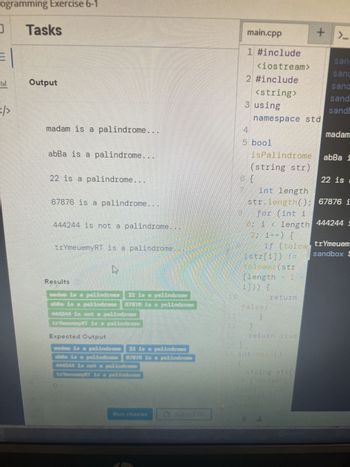
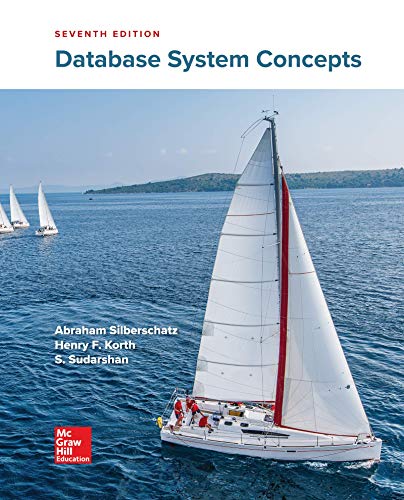
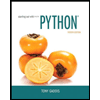
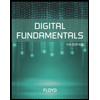
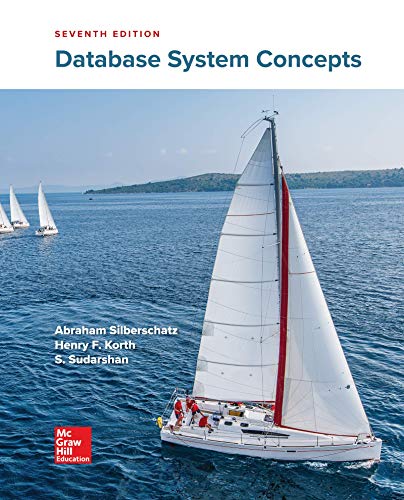
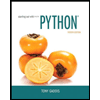
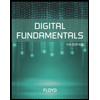
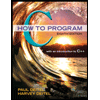
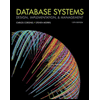
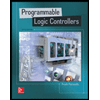