Write a C code that gets a string and reverses it in place. If the reversed string is the same as the initial string, it should return 1 otherwise should return 0
- Write a C code that gets a string and reverses it in place. If the reversed string is the same as the initial string, it should return 1 otherwise should return 0
Suggested prototype: int reverseIt(char str[])
Reference
This is a list of function prototypes of the C library functions presented in class. You may use any of these functions in your solutions (unless the requirements explicitly indicate otherwise). As you have been provided the function prototypes, you are expected to use the functions correctly in your solutions.
double atof(char *string);
int atoi(char *string);
long atol(char *string);
int fclose(FILE *filePointer);
char *fgets(char *string, int n, FILE *filePointer);
FILE *fopen(char *fileName, char *access);
int fprintf(FILE *filePointer, char *format, ...);
int fputs(char *string, FILE *filePointer);
int fscanf(FILE *filePointer, char *format, ...);
char *gets(char *ptrString);
int printf(char *format, ...);
int puts(char *ptrString);
int scanf(char *format, ...);
char *strcat(char *stringTo, char *stringFrom);
int strcmp(char *string1, char *string2);
char *strcpy(char *stringTo, char *stringFrom);
int strlen(char *string);
char *strncat(char *stringTo, char *stringFrom, int size);
int strncmp(char *string1, char *string2, int size);
char *strncpy(char *stringTo, char *stringFrom, int size);
int tolower(char oldchar);
int toupper(char oldchar);
ASCII Table
32 40 ( 48 0 56 8 64 @ 72 H 80 P 88 X 96 ` 104 h 112 p 120 x
33 ! 41 ) 49 1 57 9 65 A 73 I 81 Q 89 Y 97 a 105 i 113 q 121 y
34 " 42 * 50 2 58 : 66 B 74 J 82 R 90 Z 98 b 106 j 114 r 122 z
35 # 43 + 51 3 59 ; 67 C 75 K 83 S 91 [ 99 c 107 k 115 s 123 {
36 $ 44 , 52 4 60 < 68 D 76 L 84 T 92 \ 100 d 108 l 116 t 124 |
37 % 45 - 53 5 61 = 69 E 77 M 85 U 93 ] 101 e 109 m 117 u 125 }
38 & 46 . 54 6 62 > 70 F 78 N 86 V 94 ^ 102 f 110 n 118 v 126 ~
39 ' 47 / 55 7 63 ? 71 G 79 O 87 W 95 _ 103 g 111 o 119 w 127
Hint: the answer need include both output and use c code to answer it

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

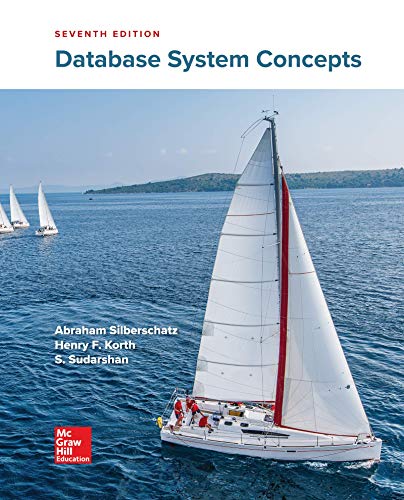
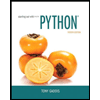
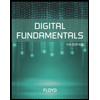
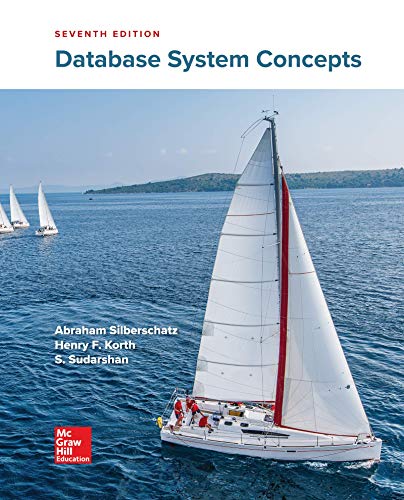
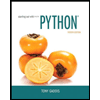
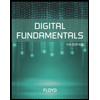
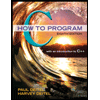
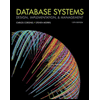
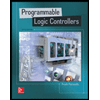