Your friend is working on an app for jobseekers. She sends you this bit of code: name "Tim Tester" age 20 skilll "python" level1 = "beginner" skil12"java" level2 = "veteran" skill3 "programming" level3 "semiprofessional" lower = 2000 upper 3000 print("my name is ", name, ", I am ", age, "years old") print("my skills are") print(" ", skilll, (", level1, ")") print(" ", skil12, "(", level2, ")") print("-", skil13, " (", level3, ")") print("I am looking for a job with a salary of", lower, The program should print out exactly the following: my name is Tim Tester, I am 20 years old my skills are python (beginner) " - java (veteran) - programming (semiprofessional) I am looking for a job with a salary of 2000-3000 euros per month upper, "euros per mo The code works almost correctly, but not quite. This exercise has very strict tests, which check the output for every single bit of whitespace. Please fix the code so that the printout looks right. Notice especially how the comma notation in the print command automatically inserts a space around the different comma-separated parts. Sample output The easiest way to transform the code so that it meets requirements is to use f- strings. 1 2 name = "Tim Tester" 3 age = 20 4 skilll "python" Hint: you can print an empty line by adding an empty print command, or by adding the newline character \n into your string. Do remember to be extra careful when formatting printouts also in the future on this course. Some of the exercises have tests that require your output to be exactly as specified in the examples given. For example, please use actual whitespace characters in your code, instead of ASCII character codes for whitespace, or some such. 5 level1 "beginner" 6 skill2 = "java" 7 level2= "veteran" 8 skill3 "programming" level3 = "semiprofessional" E 9 10 lower = 2000 11 upper = 3000 12 print (f"my name is (name), I am {age} years old\n") 13 print("my skills are") 14 print (f" (skill1} ({level1])") 15 print (f"- {skill2} ({level2))") 16 print (f" (skil113) ({level3})\n") 17 print (f"I am looking for a job with a salary of (lower}-{upper}, euros per month")
Your friend is working on an app for job seekers. She sends you this bit of code as shown in the first photo:
My edits on the already established code are made starting from lines 12-17, however, there is an error message that the lines regarding python (beginner) are incorrect on line 14. See the bottom of the first photo for a reference as to where I made my revisions.
The second photo informs me that I have incorrectly written the code according to Python MOOC 2023, and I'm unsure how to fix my mistake. Please help me. I realise this looks like a portion of a graded assignment; however, this is not from any official university or school content. This is a Python course from the University of Helsinki 2023, which is a free resource that is available to anyone that is interested in learning the



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

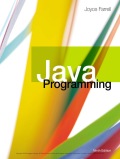
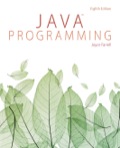
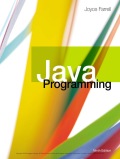
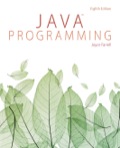