You plan to revise on all learnt topics and explore the sequence of repetitive printing using while loop. For this, you decide to write a modular C++ program to neatly print a table of sine, cosine and tangent for a range (i.e. lower and upper bounds) of radian values based on userentered angle range, on screen as well as in an output file. The increment between successive angle values is based user-entered angle value. You plan to implement at least THREE user-defined functions and your program must first check to ensure that the lower bound angle value is smaller than the upper bound angle value and that the incremental angle value is smaller than the absolute value of lower bound angle. If not, an error message is to be displayed and the program ends.
The topics are:
>>How to declare constants.
>>How to Implement Arithmetic Operations.
>>How to Implement Decision (Part 1: if - elseif - else).
please this is a C++ program.


main.cpp
#include <iostream>
#include <cstdlib>
#include <math.h>
#include <fstream>
#include <iomanip>
using namespace std;
int validate(float lowerValue, float upperValue,float incValue)
{
if(lowerValue>=upperValue)
return 0;
else if(abs(lowerValue)<=incValue)
return 0;
return 1;
}
void print(float lowerValue, float upperValue,float incValue)
{
float angle;
cout<<"\n --------------------------------------------------------------------------";
cout<<"\n Angle \t sine \t\t cosine \t tangent";
cout<<"\n --------------------------------------------------------------------------"<<endl;
for(float i = lowerValue;lowerValue <= upperValue;lowerValue+=incValue)
{
angle = lowerValue * 3.14159/180;
std::cout<<" "<<lowerValue<<"\t\t"<<sin(angle)<<"\t"<<cos(angle)<<"\t"<<tan(angle)<<endl;
}
}
void printToFile(float lowerValue, float upperValue,float incValue)
{
ofstream outputFile;
outputFile.open("output.txt");
float angle;
outputFile<<"\n --------------------------------------------------------------------------";
outputFile<<"\n Angle \t sine \t\t cosine \t tangent";
outputFile<<"\n --------------------------------------------------------------------------"<<endl;
for(float i = lowerValue;lowerValue <= upperValue;lowerValue+=incValue)
{
angle = lowerValue * 3.14159/180;
outputFile<<" "<<lowerValue<<"\t\t"<<sin(angle)<<"\t"<<cos(angle)<<"\t"<<tan(angle)<<endl;
}
outputFile.close();
}
int main()
{
float lowerValue, upperValue, incValue;
cout<<"\n Lower bound angle value: ";
cin>>lowerValue;
cout<<"\n Upper bound angle value: ";
cin>>upperValue;
cout<<"\n Incremental angle value: ";
cin>>incValue;
if(validate(lowerValue, upperValue, incValue))
{
print(lowerValue, upperValue, incValue);
printToFile(lowerValue, upperValue, incValue);
}
else
{
cout<<"\n Please enter valid input.";
}
return 0;
}
Step by step
Solved in 2 steps with 2 images

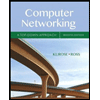
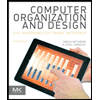
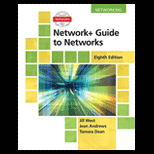
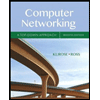
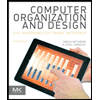
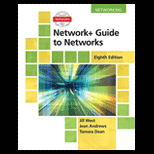
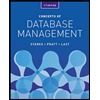
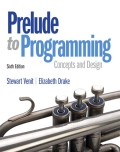
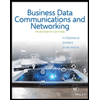