You need to implement a class named "Queue" that simulates a basic queue data structure. The class should have the following methods: Enqueue(int value) - adds a new element to the end of the queue Dequeue() - removes the element from the front of the queue and returns it Peek() - returns the element from the front of the queue without removing it Count() - returns the number of elements in the queue The class should also have a property named "IsEmpty" that returns a boolean indicating whether the queue is empty or not. Constraints: The queue should be implemented using an array and the array should automatically resize when needed. All methods and properties should have a time complexity of O(1) You can write the program in C# and use the test cases to check if your implementation is correct. An example of how the class should be used: Queue myQueue = new Queue(); myQueue.Enqueue(1); myQueue.Enqueue(2); myQueue.Enqueue(3); Console.WriteLine(myQueue.Peek()); // 1 Console.WriteLine(myQueue.Dequeue()); // 1 Console.WriteLine(myQueue.Count()); // 2 Console.WriteLine(myQueue.IsEmpty); // false
You need to implement a class named "Queue" that simulates a basic queue data structure. The class should have the following methods:
Enqueue(int value) - adds a new element to the end of the queue
Dequeue() - removes the element from the front of the queue and returns it
Peek() - returns the element from the front of the queue without removing it
Count() - returns the number of elements in the queue
The class should also have a property named "IsEmpty" that returns a boolean indicating whether the queue is empty or not.
Constraints:
The queue should be implemented using an array and the array should automatically resize when needed.
All methods and properties should have a time complexity of O(1)
You can write the program in C# and use the test cases to check if your implementation is correct.
An example of how the class should be used:
Queue myQueue = new Queue();
myQueue.Enqueue(1);
myQueue.Enqueue(2);
myQueue.Enqueue(3);
Console.WriteLine(myQueue.Peek()); // 1
Console.WriteLine(myQueue.Dequeue()); // 1
Console.WriteLine(myQueue.Count()); // 2
Console.WriteLine(myQueue.IsEmpty); // false

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

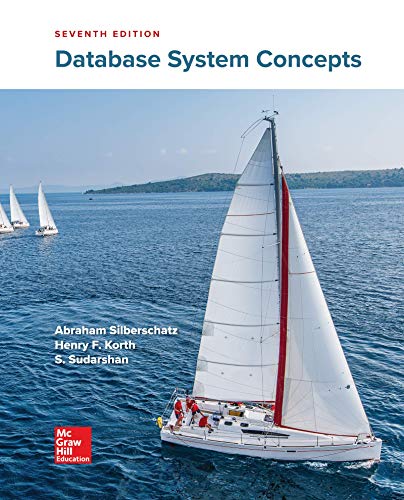
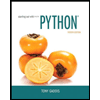
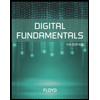
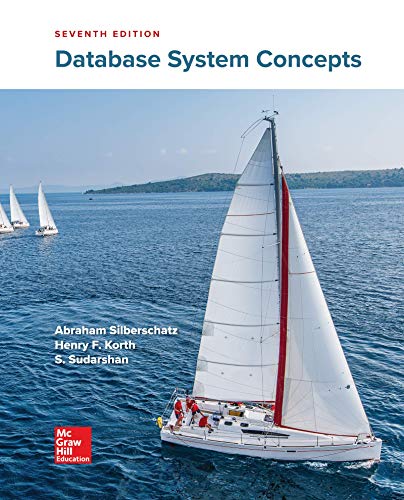
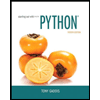
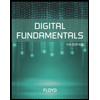
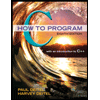
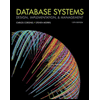
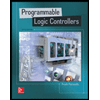