You have a Tamagotchi toy (kids still know what these are these days, right?) and it requires a lot of attention. Complete the function tamagotchi, which takes three arguments that describe the state of your pet (food_level, dirt_level, playful) and a fourth that indicates how many 10-minute time periods to simulate (num_time_periods): food_level: an integer that represents how full the pet's tummy is (higher numbers indicate feeling more full) • dirt_level: an integer that represents how dirty the pet is (higher numbers indicate more dirt) • playful: a Boolean indicating if the pet is in a playful mood num_time_periods: how many time periods to simulate (i.e., how many loop iterations to execute) For each time period, determine what action your pet takes and add a string to a list that records the action. At the end of the function, return the list of strings. Pick the correct action based on the following set of conditions, which you should check in this order: • If the pet is dirty (dirt_level is at least 10), it cleans itself (dirt_level goes down by 10). Append wash to the list of actions. • If the pet is in a playful mood and very hungry (food_level is less than 50), the pet binges on food (food_level goes up by 20) and gets very dirty (dirt_level goes up by 7). Append binge to the list of actions. • If the pet is in a playful mood but only a little hungry (food_level is in the range 50-70, inclusive), the pet eats modestly (food_level goes up by 5). Append eat to the list of actions. • If the pet is in playful mood and not hungry, it plays. Its dirt_level goes up by 3, and food_level goes down by 2. Append play to the list of actions. • If none of the above actions applies, the pet does nothing. Add idle to the list of actions. Example #1: tamagotchi (56, 6, True, 10) Return value: ['eat', 'eat', 'eat', 'play', 'eat', 'play', 'wash', 'play', 'eat', 'play'] Example #2: tamagotchi (25, 16, True, 8) Return value: ['wash', 'binge', 'wash', 'binge', 'wash', 'eat', 'play'] Example # 3: tamagotchi (72, 47, False, 13) Return value: ['wash', 'wash', 'wash', 'wash' 'idle' idle 'idle', 'idle', 'idle 'idle', 'idle', 'idle', 'idle'] Example #4: tamagotchi (23, 19, True, 10) Return value: ['wash', 'binge', 'wash', 'binge', 'wash', 'eat' 'eat', 'play', 'play', 'eat'] [ ] 1 def tamagotchi (food_level, dirt_level, playful, num_time_periods): 2 return 'Error' # DELETE THIS LINE and start coding here. 3 # Remember: end all of your functions with a return statement, not a print statement! 4 5 6 # Test cases 7 print (tamagotchi (56, 6, True, 10)) 8 print (tamagotchi (25, 16, True, 8)) 9 print (tamagotchi (72, 47, False, 13)) 10 print (tamagotchi (23, 19, True, 10)) 11 print ()
You have a Tamagotchi toy (kids still know what these are these days, right?) and it requires a lot of attention. Complete the function tamagotchi, which takes three arguments that describe the state of your pet (food_level, dirt_level, playful) and a fourth that indicates how many 10-minute time periods to simulate (num_time_periods): food_level: an integer that represents how full the pet's tummy is (higher numbers indicate feeling more full) • dirt_level: an integer that represents how dirty the pet is (higher numbers indicate more dirt) • playful: a Boolean indicating if the pet is in a playful mood num_time_periods: how many time periods to simulate (i.e., how many loop iterations to execute) For each time period, determine what action your pet takes and add a string to a list that records the action. At the end of the function, return the list of strings. Pick the correct action based on the following set of conditions, which you should check in this order: • If the pet is dirty (dirt_level is at least 10), it cleans itself (dirt_level goes down by 10). Append wash to the list of actions. • If the pet is in a playful mood and very hungry (food_level is less than 50), the pet binges on food (food_level goes up by 20) and gets very dirty (dirt_level goes up by 7). Append binge to the list of actions. • If the pet is in a playful mood but only a little hungry (food_level is in the range 50-70, inclusive), the pet eats modestly (food_level goes up by 5). Append eat to the list of actions. • If the pet is in playful mood and not hungry, it plays. Its dirt_level goes up by 3, and food_level goes down by 2. Append play to the list of actions. • If none of the above actions applies, the pet does nothing. Add idle to the list of actions. Example #1: tamagotchi (56, 6, True, 10) Return value: ['eat', 'eat', 'eat', 'play', 'eat', 'play', 'wash', 'play', 'eat', 'play'] Example #2: tamagotchi (25, 16, True, 8) Return value: ['wash', 'binge', 'wash', 'binge', 'wash', 'eat', 'play'] Example # 3: tamagotchi (72, 47, False, 13) Return value: ['wash', 'wash', 'wash', 'wash' 'idle' idle 'idle', 'idle', 'idle 'idle', 'idle', 'idle', 'idle'] Example #4: tamagotchi (23, 19, True, 10) Return value: ['wash', 'binge', 'wash', 'binge', 'wash', 'eat' 'eat', 'play', 'play', 'eat'] [ ] 1 def tamagotchi (food_level, dirt_level, playful, num_time_periods): 2 return 'Error' # DELETE THIS LINE and start coding here. 3 # Remember: end all of your functions with a return statement, not a print statement! 4 5 6 # Test cases 7 print (tamagotchi (56, 6, True, 10)) 8 print (tamagotchi (25, 16, True, 8)) 9 print (tamagotchi (72, 47, False, 13)) 10 print (tamagotchi (23, 19, True, 10)) 11 print ()
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![This instructional content explains how to complete the function `tamagotchi`, which simulates the behavior of a Tamagotchi toy over a specified number of time periods. The function requires four parameters:
- **food_level:** An integer representing how full the pet's tummy is (higher numbers mean more full).
- **dirt_level:** An integer representing how dirty the pet is (higher numbers mean more dirt).
- **playful:** A Boolean indicating if the pet is in a playful mood.
- **num_time_periods:** The number of time periods to simulate (i.e., how many loop iterations to execute).
For each time period, the function determines which action the pet takes and adds a corresponding string to a list:
1. If the pet is dirty (dirt_level ≥ 10), it cleans itself (dirt_level decreases by 10). Append "wash" to the actions list.
2. If the pet is playful and very hungry (food_level < 50), it binges on food (food_level increases by 20) and gets very dirty (dirt_level increases by 7). Append "binge" to the actions list.
3. If the pet is playful and moderately hungry (food_level is between 50 and 70), it eats modestly (food_level increases by 5). Append "eat" to the actions list.
4. If the pet is playful and not hungry, it plays (dirt_level increases by 3 and food_level decreases by 2). Append "play" to the actions list.
5. If none of the conditions apply, the pet does nothing. Append "idle" to the actions list.
**Example Outputs:**
- **Example #1:** `tamagotchi(56, 6, True, 10)`
- Return value: `['eat', 'eat', 'eat', 'play', 'eat', 'play', 'wash', 'play', 'eat', 'play']`
- **Example #2:** `tamagotchi(25, 16, True, 8)`
- Return value: `['wash', 'binge', 'wash', 'binge', 'wash', 'eat', 'eat', 'play']`
- **Example #3:** `tamagotchi(72, 47, False, 13)`
- Return value: `['wash', 'wash', 'wash', 'wash', 'idle](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa684ef64-b106-44e3-b477-7930de74cd26%2F7efce05d-41a6-4580-ad73-38c4638b6992%2Fli9l7or_processed.png&w=3840&q=75)
Transcribed Image Text:This instructional content explains how to complete the function `tamagotchi`, which simulates the behavior of a Tamagotchi toy over a specified number of time periods. The function requires four parameters:
- **food_level:** An integer representing how full the pet's tummy is (higher numbers mean more full).
- **dirt_level:** An integer representing how dirty the pet is (higher numbers mean more dirt).
- **playful:** A Boolean indicating if the pet is in a playful mood.
- **num_time_periods:** The number of time periods to simulate (i.e., how many loop iterations to execute).
For each time period, the function determines which action the pet takes and adds a corresponding string to a list:
1. If the pet is dirty (dirt_level ≥ 10), it cleans itself (dirt_level decreases by 10). Append "wash" to the actions list.
2. If the pet is playful and very hungry (food_level < 50), it binges on food (food_level increases by 20) and gets very dirty (dirt_level increases by 7). Append "binge" to the actions list.
3. If the pet is playful and moderately hungry (food_level is between 50 and 70), it eats modestly (food_level increases by 5). Append "eat" to the actions list.
4. If the pet is playful and not hungry, it plays (dirt_level increases by 3 and food_level decreases by 2). Append "play" to the actions list.
5. If none of the conditions apply, the pet does nothing. Append "idle" to the actions list.
**Example Outputs:**
- **Example #1:** `tamagotchi(56, 6, True, 10)`
- Return value: `['eat', 'eat', 'eat', 'play', 'eat', 'play', 'wash', 'play', 'eat', 'play']`
- **Example #2:** `tamagotchi(25, 16, True, 8)`
- Return value: `['wash', 'binge', 'wash', 'binge', 'wash', 'eat', 'eat', 'play']`
- **Example #3:** `tamagotchi(72, 47, False, 13)`
- Return value: `['wash', 'wash', 'wash', 'wash', 'idle
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
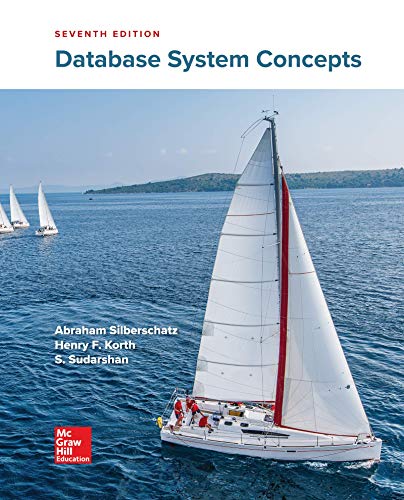
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
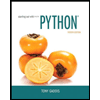
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
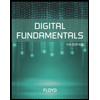
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
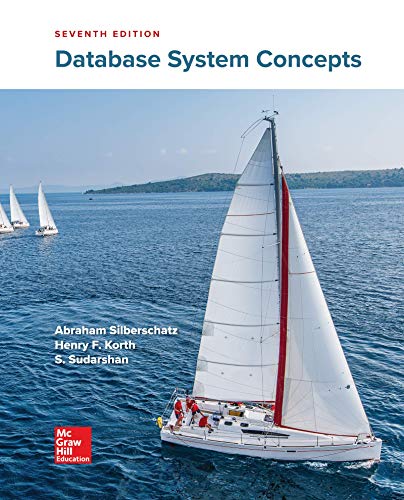
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
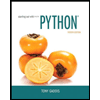
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
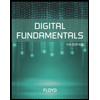
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
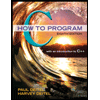
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
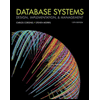
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
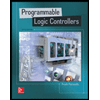
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education