Please write a program that computes the average of 3 exam scores of 3 students. It first asks the user three exam scores for each student and then compute the average of them. The program should have the following functions. - int get TestScore(): This function is called by main function. It asks the user to enter the score of the exam. Once it gets a user input data, the function returns it to the main function. Input Validation: Please check if the entered score is between 0 and 100 inclusive (considered valid). If not, repeat asking to enter a valid score. -double calAverage(int, int, int, int, int): This function is called by main function. It calculates the average of 3 exam scores passed from main function in int data type. Once the function calculates the average, it returns it to main function in double data type. -void display(string, double): This function is called by main function and displays the student's name and average exam score. The arguments are students' name and the average exam score. .main(): Main function calls getTestScore() 3 times for each student to get the student's 3 exam scores. And then, it calls calAverage() and display() to compute and displace the average of the 3 exam scores for that student. Please complete this process for 3 students using loop structure.


NOTE : As the given question does not specify the programming language in which the program has to be written. So C++ will be used to code the same.
Step 1 : Start
Step 2 : Define a function getTestScore() to input the test score of the student. Taking user input for the test score till a valid score is input by the user. Return the input score if valid.
Step 3 : Define a function calcAverage() to calculate and return the average of the 3 scores.
Step 4 : Define a function display() to print the student name and calculated average.
Step 5 : In the main function , Define a for loop to get the scores for 3 students. In the loop input the name of the student.
Step 6 : Call the calcAverage() function and passing the 3 scores as arguments. Call the display() function to print the student name and their average. Repeat these steps for all the 3 students.
Step 7 : Stop
Step by step
Solved in 4 steps with 6 images

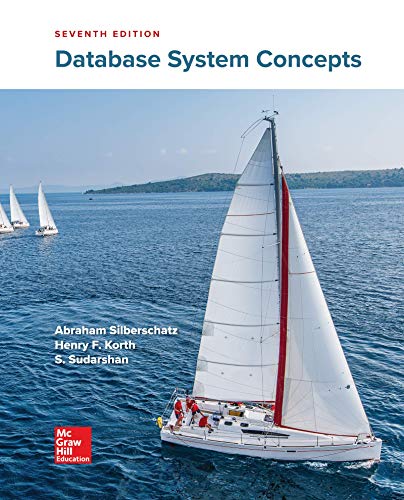
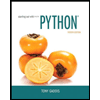
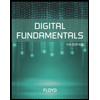
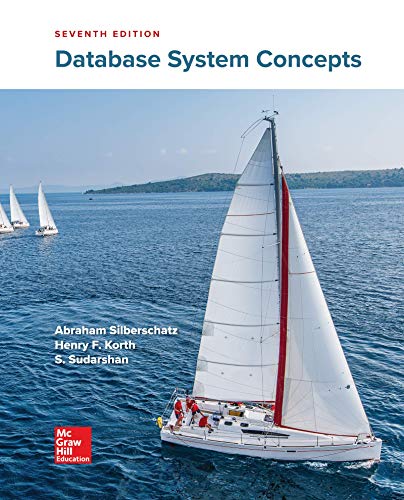
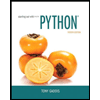
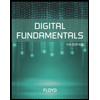
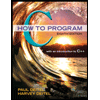
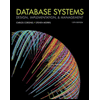
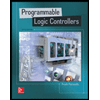