You are working on problem set: HW2 - loops (Pause) numberLoops4constant Language/Type: Java for nested loops ascii art class constants The following program uses nested for loops to produce the following output: ....1 ..2. ..3.. .4... 5.... Modify the program to use a single integer constant named SIZE that influences how many lines should be drawn. For example, if the SIZE is changed to 7, the output should become the following: ......1 .2. .3.. .4... ..5.... .6... 7...... 1 public class Number Loops4 { 2 4 6 8 9 10 11 public static void main(String[] args) { for (int i = 1; i <= 5; i++) { for (int j = 1; j <= 5 - i; j++) { System.out.print("."); } } } System.out.print(i); for (int j = 1; j <= i - 1; j++) { System.out.print("."); } System.out.println(); 12 13 14) Class: Write a complete Java class. Submit
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
![## numberLoops4constant
**Language/Type:** Java
### Objective
The following program uses nested `for` loops to produce the output shown below:
```
....
...1
..2
.3
4
5
```
### Task
Modify the program to use a single integer constant named `SIZE` that influences how many lines should be drawn. For example, if the `SIZE` is changed to 7, the output should become the following:
```
......
.....1
....2
...3
..4
.5
6
7
```
### Explanation
The program's code is displayed on the right side:
```java
public class NumberLoops4 {
public static void main(String[] args) {
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= 5 - i; j++) {
System.out.print(".");
}
System.out.print(i);
for (int j = 1; j <= i - 1; j++) {
System.out.print(".");
}
System.out.println();
}
}
}
```
### Code Explanation
- **Outer Loop:** Iterates over each line number `i` from 1 to 5.
- **First Inner Loop:** Prints a decreasing number of periods (dots) before the number. It prints `5 - i` periods.
- **Print Statement:** Outputs the current line number `i`.
- **Second Inner Loop:** Prints an increasing number of periods after the number. It prints `i - 1` periods.
- **New Line:** `System.out.println();` ensures that each line is printed on a new line.
**Class Task:** Modify the program to use a constant `SIZE` to make the output flexible for different numbers of lines.
### Interface
Finalized code should be able to adjust for the value set for `SIZE` and produce accordingly structured output.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdf909276-6ad3-4144-b98c-235c5a32e436%2F49d9ccfd-1b5e-4a80-a0bb-5a7f2b2b3a32%2F0v7n1io_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

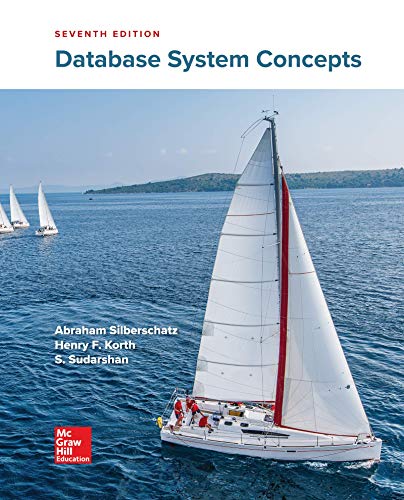
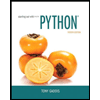
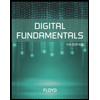
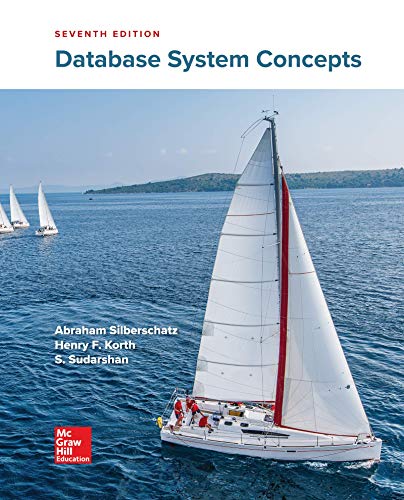
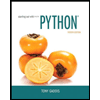
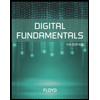
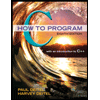
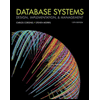
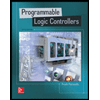