You are responsible to write an assembly function to perform searching of a list of names in stored in ascending order. This function will use the linear search algorithm. The names could be stored in mixed case. Therefore your assembly function must do the comparison in a case insensitive manner. This function will use the C function signature but everything within this function should be assembly code using the ASM block similar to the assembly example shown in class. long search_by_name (char* list, long count, char* token); list – the starting address of the list of structures to be searched count – total number of names in the list token – name to be search in the list long search_by_name (char* list, long count, char* token) { long index = 0; /* Replace the following C code with the ASM block */ // x86-64, in-line assembly struct student *clist = (struct student*)list; for (index = 0; index < count; index++) if ( strcasecmp( clist[index].name, token ) == 0) return clist[index].ID; return 0;
You are responsible to write an assembly function to perform searching of a list of names in stored in ascending order. This function will use the linear search
long search_by_name (char* list, long count, char* token);
list – the starting address of the list of structures to be searched
count – total number of names in the list
token – name to be search in the list
long search_by_name (char* list, long count, char* token)
{
long index = 0;
/* Replace the following C code with the ASM block */
// x86-64, in-line assembly
struct student *clist = (struct student*)list;
for (index = 0; index < count; index++)
if ( strcasecmp( clist[index].name, token ) == 0)
return clist[index].ID;
return 0;

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

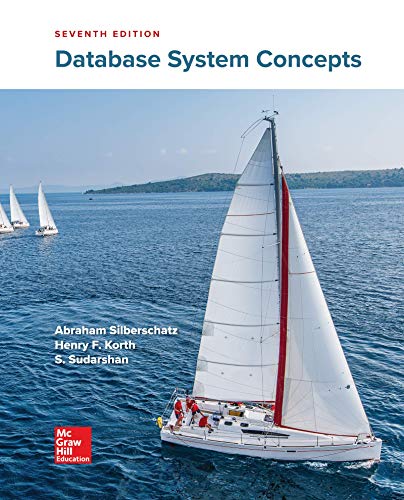
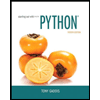
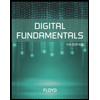
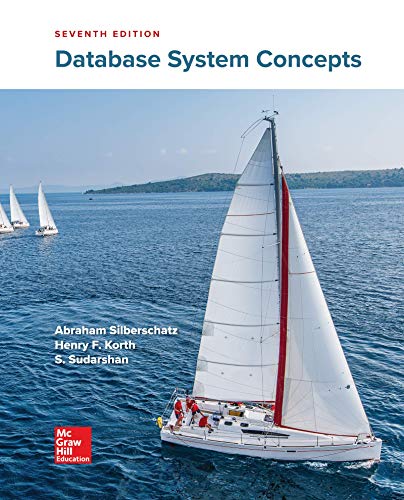
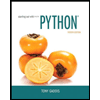
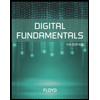
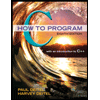
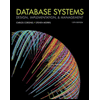
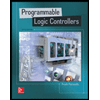