Here the incoming argument should be parsed in the form of "PyObject *", which refers o iterator of Python. The iterator should then allow us to extract all the list data. The xtracted data should be kept in an internal long integer array in your C program. Note C anguage typically require a fixed size for the array. We set it to be 20000 in this exercise fter applying C sorting to the array and finally your extension should return the sorted list s a list object back to the calling Python command. Figure 2 shows the step for you to test rour module. After you successfully test your module, name your module file as selectSort.c.
Here the incoming argument should be parsed in the form of "PyObject *", which refers o iterator of Python. The iterator should then allow us to extract all the list data. The xtracted data should be kept in an internal long integer array in your C program. Note C anguage typically require a fixed size for the array. We set it to be 20000 in this exercise fter applying C sorting to the array and finally your extension should return the sorted list s a list object back to the calling Python command. Figure 2 shows the step for you to test rour module. After you successfully test your module, name your module file as selectSort.c.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
The question is in the first picture. Please provide code and detailed explanation.
![j@Jinchengs-MacBook-Pro:-/Desktop/lab05/answer|
- cat setup.py
from distutils.core import setup, Extension
setup(name - 'myModule', version- '1.0', V
ext modules - [Extension('myModule', ['selectSort.c'])])
jceJinchengs-MacBook-Pro:-/Desktop/lab05/answer|
-python setup.py butld
running butld
running butld_ext
jc@Jinchengs-MacBook-Pro:-/Desktop/lab05/answer|
- python setup.py install
running instali
running butld
running build_ext
running install_lib
copying build/lib.racosx-10.9-x86_64-3.7/myModule.cpython-37m-darwin.so -> /Users/jc/anaconda3/1ib/python3.7/site-packages
running install_egg_info
Removing /Users/jc/anaconda3/Lib/python3.7/site-packages/myModule-1.0-py3.7.egg-info
Writing Users/jc/anaconda3/lib/python3.7/site-packages/nyModule-1.0-py3.7.egg-info
jc@Jinchengs-MacBook-Pro:-/Desktop/lab05/answer|
- python
Python 3.7.4 (default, Aug 13 2019, 15:17:50)
[Clang 4.0.1 (tags/RELEASE_401/final)] :: Anaconda, Inc. on darwin
Type "help", "copyright", "credits" or "license" for more information.
> import myModule
> unsortedlist - 3, 24, 15, 1, 100, 70, 17]
> sortedList - myModule.selectSort(unsortedList)
>>print(sortedList)
[1, 3, 15, 17, 24, 70, 100]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffc8d80de-c6bf-4733-98b7-66f87108d889%2F914979ab-1169-463a-9cec-e81155ed2ea1%2Fdqzjh2s_processed.png&w=3840&q=75)
Transcribed Image Text:j@Jinchengs-MacBook-Pro:-/Desktop/lab05/answer|
- cat setup.py
from distutils.core import setup, Extension
setup(name - 'myModule', version- '1.0', V
ext modules - [Extension('myModule', ['selectSort.c'])])
jceJinchengs-MacBook-Pro:-/Desktop/lab05/answer|
-python setup.py butld
running butld
running butld_ext
jc@Jinchengs-MacBook-Pro:-/Desktop/lab05/answer|
- python setup.py install
running instali
running butld
running build_ext
running install_lib
copying build/lib.racosx-10.9-x86_64-3.7/myModule.cpython-37m-darwin.so -> /Users/jc/anaconda3/1ib/python3.7/site-packages
running install_egg_info
Removing /Users/jc/anaconda3/Lib/python3.7/site-packages/myModule-1.0-py3.7.egg-info
Writing Users/jc/anaconda3/lib/python3.7/site-packages/nyModule-1.0-py3.7.egg-info
jc@Jinchengs-MacBook-Pro:-/Desktop/lab05/answer|
- python
Python 3.7.4 (default, Aug 13 2019, 15:17:50)
[Clang 4.0.1 (tags/RELEASE_401/final)] :: Anaconda, Inc. on darwin
Type "help", "copyright", "credits" or "license" for more information.
> import myModule
> unsortedlist - 3, 24, 15, 1, 100, 70, 17]
> sortedList - myModule.selectSort(unsortedList)
>>print(sortedList)
[1, 3, 15, 17, 24, 70, 100]
![We will develop a C extension module using the myModule framework for sorting applications.
Specifically you are required to develop a C module for applying selection sort algorithm to a
list of data, and returning the sorted list back to Python program as a Python list format.
Remember selection sort is one of the simplest sorting algorithm in that the smallest (largest)
element in the unsorted region is remembered at each pass, and swapped with the sorting
position. The pseudo code is provided below:
// Given an array A[] of size N
for i = 0 to N-2 {
minPos =
position of the smallest element in the array A;
Swap A[i] with A [minPos];
The function prototype for our selection sort in C source should be in the form.
static Py0bject* selectSort(Py0bject* self, Py0bject* args)
Here the incoming argument should be parsed in the form of "PyObject *", which refers
to iterator of Python. The iterator should then allow us to extract all the list data. The
extracted data should be kept in an internal long integer array in your C program. Note C
language typically require a fixed size for the array. We set it to be 20000 in this exercise.
After applying C sorting to the array and finally your extension should return the sorted list
as a list object back to the calling Python command. Figure 2 shows the step for you to test
your module.
After you successfully test your module, name your module file as selectSort.c.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffc8d80de-c6bf-4733-98b7-66f87108d889%2F914979ab-1169-463a-9cec-e81155ed2ea1%2F3i3qqbl_processed.png&w=3840&q=75)
Transcribed Image Text:We will develop a C extension module using the myModule framework for sorting applications.
Specifically you are required to develop a C module for applying selection sort algorithm to a
list of data, and returning the sorted list back to Python program as a Python list format.
Remember selection sort is one of the simplest sorting algorithm in that the smallest (largest)
element in the unsorted region is remembered at each pass, and swapped with the sorting
position. The pseudo code is provided below:
// Given an array A[] of size N
for i = 0 to N-2 {
minPos =
position of the smallest element in the array A;
Swap A[i] with A [minPos];
The function prototype for our selection sort in C source should be in the form.
static Py0bject* selectSort(Py0bject* self, Py0bject* args)
Here the incoming argument should be parsed in the form of "PyObject *", which refers
to iterator of Python. The iterator should then allow us to extract all the list data. The
extracted data should be kept in an internal long integer array in your C program. Note C
language typically require a fixed size for the array. We set it to be 20000 in this exercise.
After applying C sorting to the array and finally your extension should return the sorted list
as a list object back to the calling Python command. Figure 2 shows the step for you to test
your module.
After you successfully test your module, name your module file as selectSort.c.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
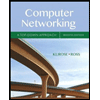
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
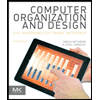
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
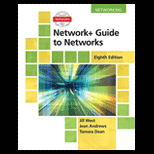
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
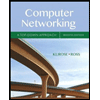
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
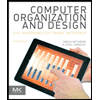
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
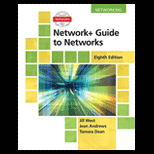
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
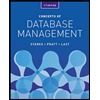
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
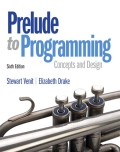
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
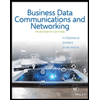
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY