Xadd_to LogisticRegression def fit(self, X, Y, epochs=1000, print_loss=True): This function implements the Gradient Descent Algorithm Arguments: x -- training data matrix: each column is a training example. The number of columns is equal to the number of training examples Y -- true "label" vector: shape (1, m) epochs -- Return: params -- dictionary containing weights losses -- loss values of every 100 epochs grads dictionary containing dw and dw_e -- losses = [1] for i in range(epochs): # Get the number of training examples m = x. shape[1] ### START YOUR CODE HERE ### # Calculate the hypothesis outputs A (* 2 lines of code) Z = A = # Calculate loss (* 1 line of code) loss = # calculate the gredients for W and w_e dw = dw_e = # weight updates self.W = self.w_e = ### YOUR CODE ENDS ###
Xadd_to LogisticRegression def fit(self, X, Y, epochs=1000, print_loss=True): This function implements the Gradient Descent Algorithm Arguments: x -- training data matrix: each column is a training example. The number of columns is equal to the number of training examples Y -- true "label" vector: shape (1, m) epochs -- Return: params -- dictionary containing weights losses -- loss values of every 100 epochs grads dictionary containing dw and dw_e -- losses = [1] for i in range(epochs): # Get the number of training examples m = x. shape[1] ### START YOUR CODE HERE ### # Calculate the hypothesis outputs A (* 2 lines of code) Z = A = # Calculate loss (* 1 line of code) loss = # calculate the gredients for W and w_e dw = dw_e = # weight updates self.W = self.w_e = ### YOUR CODE ENDS ###
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![```python
%%add_to_LogisticRegression
def fit(self, X, Y, epochs=1000, print_loss=True):
"""
This function implements the Gradient Descent Algorithm
Arguments:
X -- training data matrix: each column is a training example.
The number of columns is equal to the number of training examples
Y -- true "label" vector: shape (1, m)
epochs --
Return:
params -- dictionary containing weights
losses -- loss values of every 100 epochs
grads -- dictionary containing dw and dw_0
"""
losses = []
for i in range(epochs):
# Get the number of training examples
m = X.shape[1]
### START YOUR CODE HERE ###
# Calculate the hypothesis outputs A (≈ 2 lines of code)
Z =
A =
# Calculate loss (≈ 1 line of code)
loss =
# Calculate the gradients for W and w_0
dw =
dw_0 =
# Weight updates
self.W =
self.w_0 =
### YOUR CODE ENDS ###
```
This code snippet belongs to a Python class method intended to implement gradient descent for logistic regression. Here is a detailed explanation:
- **Function Definition**: The `fit` function is defined within a class, likely `LogisticRegression`. It accepts training data `X` and labels `Y`, along with optional parameters `epochs` and `print_loss`.
- **Arguments**:
- `X`: A matrix where each column represents a training example. The number of columns matches the number of training examples.
- `Y`: A vector containing the true labels for the training data. It has a shape of (1, m).
- `epochs`: Specifies the number of iterations for the gradient descent.
- **Returns**:
- `params`: A dictionary of weight parameters.
- `losses`: Captures the loss value every 100 epochs for evaluation purposes.
- `grads`: A dictionary containing the gradients `dw` and `dw_0`.
- **Core Logic**:
- The code runs a loop over the specified number of `epochs`.
- It calculates the number of training examples `m` from the shape of `X`.
- A placeholder is left for calculating the hypothesis outputs `A`, loss, and](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F87a3401d-ebaa-4c6f-8559-6e893e4d1e7e%2F9ebc536d-14b8-435e-a983-2b0665030d1a%2F1x3g1ov_processed.png&w=3840&q=75)
Transcribed Image Text:```python
%%add_to_LogisticRegression
def fit(self, X, Y, epochs=1000, print_loss=True):
"""
This function implements the Gradient Descent Algorithm
Arguments:
X -- training data matrix: each column is a training example.
The number of columns is equal to the number of training examples
Y -- true "label" vector: shape (1, m)
epochs --
Return:
params -- dictionary containing weights
losses -- loss values of every 100 epochs
grads -- dictionary containing dw and dw_0
"""
losses = []
for i in range(epochs):
# Get the number of training examples
m = X.shape[1]
### START YOUR CODE HERE ###
# Calculate the hypothesis outputs A (≈ 2 lines of code)
Z =
A =
# Calculate loss (≈ 1 line of code)
loss =
# Calculate the gradients for W and w_0
dw =
dw_0 =
# Weight updates
self.W =
self.w_0 =
### YOUR CODE ENDS ###
```
This code snippet belongs to a Python class method intended to implement gradient descent for logistic regression. Here is a detailed explanation:
- **Function Definition**: The `fit` function is defined within a class, likely `LogisticRegression`. It accepts training data `X` and labels `Y`, along with optional parameters `epochs` and `print_loss`.
- **Arguments**:
- `X`: A matrix where each column represents a training example. The number of columns matches the number of training examples.
- `Y`: A vector containing the true labels for the training data. It has a shape of (1, m).
- `epochs`: Specifies the number of iterations for the gradient descent.
- **Returns**:
- `params`: A dictionary of weight parameters.
- `losses`: Captures the loss value every 100 epochs for evaluation purposes.
- `grads`: A dictionary containing the gradients `dw` and `dw_0`.
- **Core Logic**:
- The code runs a loop over the specified number of `epochs`.
- It calculates the number of training examples `m` from the shape of `X`.
- A placeholder is left for calculating the hypothesis outputs `A`, loss, and
Expert Solution

Step 1
Answer:
We have complete the function. also attached the code in next page.
Step by step
Solved in 4 steps with 1 images

Recommended textbooks for you
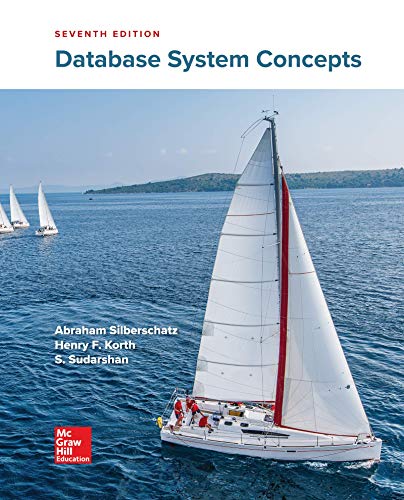
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
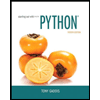
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
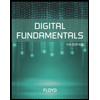
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
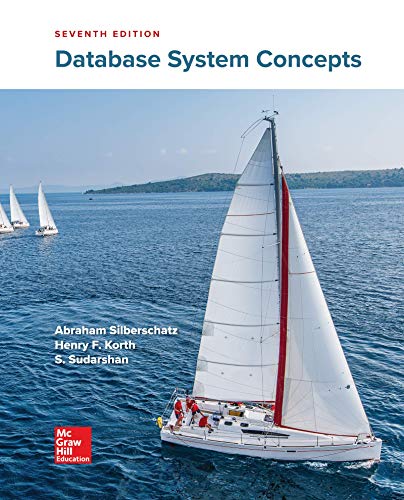
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
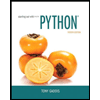
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
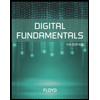
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
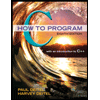
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
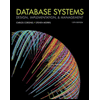
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
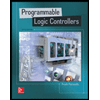
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education