Now you will create a simple C++ matrix class. Do not be intimidated if you have no experience with matrices Instead of the word "matrix" just think 2-D vectors. Download the starter code nain.cpp and Matrix.h from CCLE. We have provided main.cpp to give you an idea of how we intend to use the class. You may not use global variables • num.rovs represents the number of rows in the Matrix object; • num.cols represents the number of columns in the Matrix object; • vector< vector > values stores all the entries in the matrix. • The default constructor creates a matrix class object with size 1 by 1 and entry equal to 1. • The other constructor initializes a matrix using user supplied number of rows and number of columns, and sets the entries of the matrix to be the sum of row-index squares and columns-index squares.
Now you will create a simple C++ matrix class. Do not be intimidated if you have no experience with matrices Instead of the word "matrix" just think 2-D vectors. Download the starter code nain.cpp and Matrix.h from CCLE. We have provided main.cpp to give you an idea of how we intend to use the class. You may not use global variables • num.rovs represents the number of rows in the Matrix object; • num.cols represents the number of columns in the Matrix object; • vector< vector > values stores all the entries in the matrix. • The default constructor creates a matrix class object with size 1 by 1 and entry equal to 1. • The other constructor initializes a matrix using user supplied number of rows and number of columns, and sets the entries of the matrix to be the sum of row-index squares and columns-index squares.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Just the default matrix (2D

Transcribed Image Text:### Creating a Simple C++ Matrix Class
In this module, you will learn how to create a simple C++ matrix class. Don't be intimidated if you have no prior experience with matrices. For the purpose of this exercise, think of the word "matrix" as simply a set of 2D vectors. To get started, download the starter code files `main.cpp` and `Matrix.h` from CCLE. We have also provided `main.cpp` to give you an idea of how we intend to use the class. It is important to note that you may not use global variables in your implementation.
#### Key Components of the Matrix Class
1. **Attributes**:
- `num_rows`: Represents the number of rows in the Matrix object.
- `num_cols`: Represents the number of columns in the Matrix object.
- `vector< vector<int> > values`: Stores all the entries in the matrix.
2. **Constructors**:
- **Default Constructor**: Creates a matrix class object with a default size of 1 by 1, with the entry equal to 1.
- **Parameterized Constructor**: Initializes a matrix using user-supplied numbers of rows and columns. It sets each entry of the matrix to be the sum of the row index and the square of the column index.
3. **Methods**:
- Addition and other matrix operations will be performed using instances of matrix objects.
This information provides a foundation for understanding how to work with and manipulate matrices using C++. As you proceed through this module, you will be able to understand the essentials of creating and using matrix objects, ultimately enhancing your programming skills in C++.

Transcribed Image Text:## Understanding the Matrix Class in C++
### Header File: matrix.h
In the provided code snippet, we have the header file `matrix.h` which defines a Matrix class. This file contains the necessary includes, namespace usage, class definition, and member declarations.
#### Code Explanation
```cpp
// matrix.h
#ifndef MATRIX_H
#define MATRIX_H
#include <iostream>
#include <vector>
#include <fstream>
using namespace std;
class Matrix
{
public:
Matrix();
Matrix(int new_num_rows, int new_num_cols);
Matrix add(const Matrix& M2) const;
void print(ofstream& fout) const;
private:
int num_rows;
int num_cols;
vector<vector<int>> values;
};
#endif
#pragma once
```
### Detailed Breakdown
1. **Include Guard**
```cpp
#ifndef MATRIX_H
#define MATRIX_H
```
This part of the code ensures that the `matrix.h` file is included only once during the compilation process, preventing potential redefinition errors.
2. **Including Standard Libraries**
```cpp
#include <iostream>
#include <vector>
#include <fstream>
```
These includes are essential for input-output operations, using vectors to store matrix data, and handling file operations.
3. **Namespace Usage**
```cpp
using namespace std;
```
The `using namespace std` statement allows us to use all the entities in the `std` namespace without explicitly prefixing `std::`.
4. **Matrix Class Definition**
```cpp
class Matrix
{
```
Here begins the definition of the `Matrix` class.
5. **Public Member Functions**
```cpp
public:
Matrix();
Matrix(int new_num_rows, int new_num_cols);
Matrix add(const Matrix& M2) const;
void print(ofstream& fout) const;
```
This segment defines the public interface of the Matrix class:
- **Matrix()**: Default constructor.
- **Matrix(int new_num_rows, int new_num_cols)**: Parameterized constructor to initialize a matrix with specified number of rows and columns.
- **Matrix add(const Matrix& M2) const**: A function to add two matrices and return the result.
- **void print(ofstream& fout) const**: A function to print the matrix to an output file stream.
6.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 8 images

Recommended textbooks for you
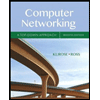
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
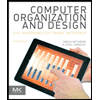
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
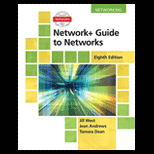
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
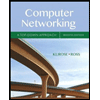
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
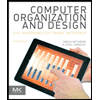
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
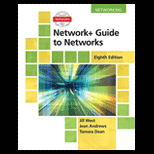
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
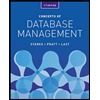
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
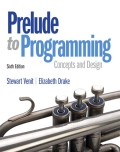
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
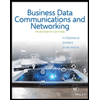
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY