Write Java program. Write an algorithm that takes a sorted list of n integers and remove the duplicate elements from the list and return the new length. The algorithm must run in O(n) time and O(1) space. We assume that: List elements are inetger Input list is already sorted Sample example: INPUT: 1 1 2 2 3 3 4 4 5 5 5 6 7 67 OUTPUT: 8 - DriverMain.java: (Do not change anyparts of the given code, only add more code) import java.util.*; import java.util.stream.Collectors; import java.lang.*; import java.io.*; class DriverMain { public static void main(String args[]) { Scanner input = new Scanner(System.in); String str = input.nextLine(); input.close(); int[] arr = Arrays.stream(str.substring(0, str.length()).split("\\s")) .map(String::trim).mapToInt(Integer::parseInt).toArray(); List list = Arrays.stream(arr).boxed().collect(Collectors.toList()); Collections.sort(list); System.out.println(ProblemSolution.removeDuplicate(list)); } } - solution.java: import java.util.*; import java.util.stream.Collectors; import java.lang.*; import java.io.*; class ProblemSolution { public static int removeDuplicate(List list) { } }
********************Answer question below:*****************
Write Java program.
Write an
We assume that:
- List elements are inetger
- Input list is already sorted
Sample example:
INPUT:
1 1 2 2 3 3 4 4 5 5 5 6 7 67
OUTPUT:
8
- DriverMain.java:
(Do not change anyparts of the given code, only add more code)
import java.util.*;
import java.util.stream.Collectors;
import java.lang.*;
import java.io.*;
class DriverMain {
public static void main(String args[]) {
Scanner input = new Scanner(System.in);
String str = input.nextLine();
input.close();
int[] arr = Arrays.stream(str.substring(0, str.length()).split("\\s"))
.map(String::trim).mapToInt(Integer::parseInt).toArray();
List<Integer> list = Arrays.stream(arr).boxed().collect(Collectors.toList());
Collections.sort(list);
System.out.println(ProblemSolution.removeDuplicate(list));
}
}
- solution.java:
import java.util.*;
import java.util.stream.Collectors;
import java.lang.*;
import java.io.*;
class ProblemSolution {
public static int removeDuplicate(List<Integer> list) {
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

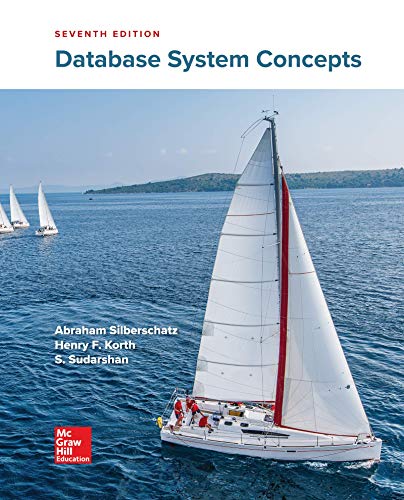
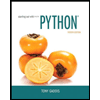
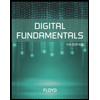
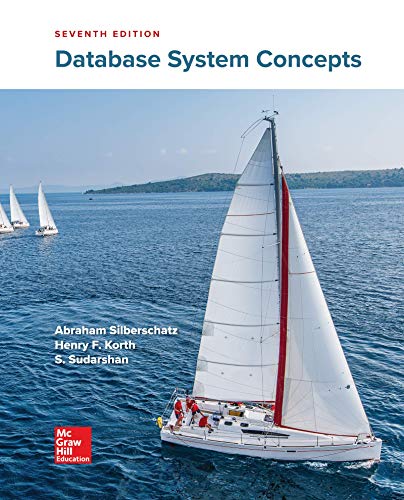
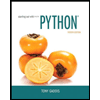
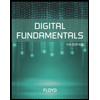
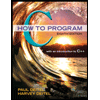
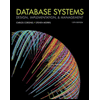
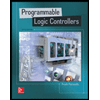