Write it in Racket Define a function (bst? n) to test for membership to determine if a collection of nodes satisfies the bst property (is a bst). The BST property is that for any node n in the bst: all nodes to left must have value < (node-value n) all nodes to right must have value > (node-value n) Skeleton for function (define (bst? n) (cond )) The function can be defined as one big conditional with multiple cases. Some of the cases are base cases, others will require a recursive call to bst? There is more than one way to develop the cases, but here are some starting point ideas. If n is null or empty, result is true #t else if n is not a node structure, then result is false #f else if n has no child nodes, then result is true #t else if n has one child on the left, then result is n.left.value < n.value AND bst?(n.left) is true else if n has one child on the right, then result is n.right.value > n.value AND bst?(n.right) is true else if n has two children, then result is n.left.value < n.value AND bst?(n.left) AND n.right.value > n.value AND bst?(n.right)
Write it in Racket
Define a function (bst? n) to test for membership to determine if a collection of nodes satisfies the bst property (is a bst). The BST property is that for any node n in the bst:
all nodes to left must have value < (node-value n)
all nodes to right must have value > (node-value n)
Skeleton for function
(define (bst? n)
(cond
))
The function can be defined as one big conditional with multiple cases. Some of the cases are base cases, others will require a recursive call to bst? There is more than one way to develop the cases, but here are some starting point ideas.
If n is null or empty, result is true #t
else if n is not a node structure, then result is false #f
else if n has no child nodes, then result is true #t
else if n has one child on the left, then result is
n.left.value < n.value AND bst?(n.left) is true
else if n has one child on the right, then result is
n.right.value > n.value AND bst?(n.right) is true
else if n has two children, then result is
n.left.value < n.value AND bst?(n.left) AND n.right.value > n.value AND bst?(n.right)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

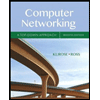
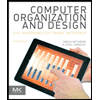
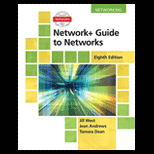
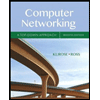
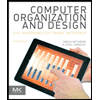
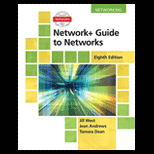
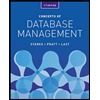
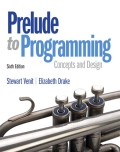
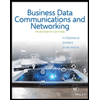