Trees: Create the 2-3 tree with 11 nodes. Also, implement in-order traversal, and findltem functions. struct Node int small; int large; Node * left; Node* middle; Node* right; void inorder (Node tree) ; Node *findItem (Node *tree, int target); 50 90 70 (120 150 (10) 30 40 60 (80 (100 110) (130 140) (160) Sample Run: CAUsers\elee\Desktop\CPSC250-Test\Debug\CPSC250-Test.exe Part 1: in-order traversal 10 20 30 40 50 60 70 80 90 100 110 120 130 140 15o 160 Part 2: find item Search 50 Item was found. Search 100 Item was found.
Trees: Create the 2-3 tree with 11 nodes. Also, implement in-order traversal, and findltem functions. struct Node int small; int large; Node * left; Node* middle; Node* right; void inorder (Node tree) ; Node *findItem (Node *tree, int target); 50 90 70 (120 150 (10) 30 40 60 (80 (100 110) (130 140) (160) Sample Run: CAUsers\elee\Desktop\CPSC250-Test\Debug\CPSC250-Test.exe Part 1: in-order traversal 10 20 30 40 50 60 70 80 90 100 110 120 130 140 15o 160 Part 2: find item Search 50 Item was found. Search 100 Item was found.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please with question in image in C++ language. Thanks

Transcribed Image Text:**2-3 Trees: Create the 2-3 tree with 11 nodes. Also, implement in-order traversal, and findItem functions.**
```c
struct Node {
int small;
int large;
Node *left;
Node *middle;
Node *right;
}
void inOrder(Node *tree);
Node *findItem(Node *tree, int target);
```
The diagram illustrates a 2-3 tree with the following structure:
- The root node contains values 50 and 90.
- The left child of the root contains values 20, with further children containing values 10, and 30/40.
- The middle child of the root contains values 70, with a child containing value 60.
- The right child of the root contains values 120 and 150, with further children containing values 100, 110, 130/140, and 160.
**Sample Run:**
A screenshot shows the console with the following output:
```
Part 1: in-order traversal
10 20 30 40 50 60 70 80 90 100 110 120 130 140 150 160
Part 2: find item
Search 50
Item was found.
Search 100
Item was found.
```
This code implements an in-order traversal that processes nodes in increasing order. The findItem function is used to search for specific values, confirming their presence in the tree.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
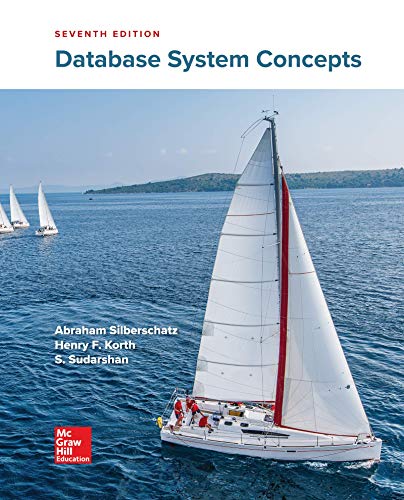
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
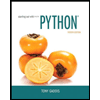
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
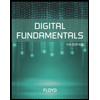
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
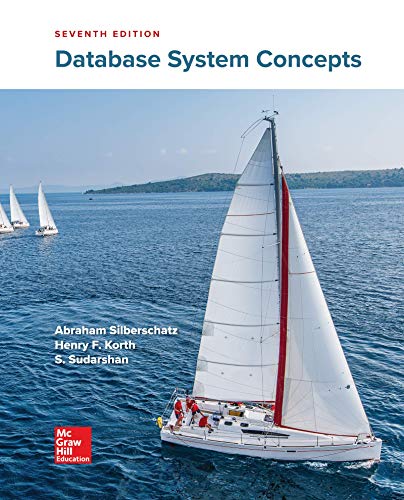
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
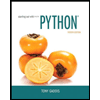
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
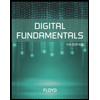
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
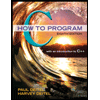
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
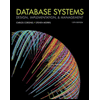
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
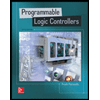
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education